Hello, Python enthusiasts! Today, let's talk about the fascinating and practical topic of Python data visualization. As a Python programming blogger, I've always had a soft spot for data visualization. Do you find yourself, like me, irresistibly drawn to those beautiful charts and animations every time you see them? Today, let's dive deep into the mysteries of Python data visualization together. From basic knowledge to advanced techniques, I'll share my insights with you without holding anything back. Are you ready? Then let's embark on this wonderful journey!
Getting to Know the Magic Tool
First, we need to get acquainted with the "superhero" of Python data visualization - Matplotlib. It's like a paintbrush in the Python world, allowing us to easily transform dull data into vivid and interesting graphics. However, you might ask, "Why choose Matplotlib?" Good question! Let me give you a few reasons:
- Powerful functionality: From simple line graphs to complex 3D graphics, Matplotlib can do almost anything.
- High flexibility: It provides two plotting interfaces, allowing both quick plotting and fine-tuned control.
- Good compatibility: It works perfectly with data processing libraries like NumPy and Pandas.
- Active community: You can always find solutions when you encounter problems.
After hearing all this, are you eager to start using it? Don't rush, let's first look at how to apply Matplotlib in practical work.
Practical Exercises
Magic in IPython Notebooks
Remember how we always had to prepare experimental equipment first when doing experiments in school? It's the same when using Matplotlib. If you like working in IPython notebooks (which I personally highly recommend), there's a little trick that can make your work even smoother:
%matplotlib inline
This line of code is like giving Matplotlib a "privilege" to display graphics directly in the notebook, instead of popping up a new window. Isn't that convenient?
Histogram: The Best Presentation of Data Distribution
Next, let's draw a histogram. Histograms are great tools for displaying data distribution, especially when you want to quickly understand the overall situation of a set of data. Let's look at the code:
import matplotlib.pyplot as plt
import numpy as np
data = np.random.randn(1000)
plt.hist(data, bins=30, alpha=0.7)
plt.title('Distribution of Random Data')
plt.xlabel('Value')
plt.ylabel('Frequency')
plt.show()
When you run this code, you'll see a beautiful histogram. The bins
parameter determines the number of bins in the histogram, while the alpha
parameter controls transparency. You can try adjusting these parameters to see how the effect changes.
Interestingly, each time you run this code, you'll get a slightly different graph because we're using random data. Doesn't this perfectly demonstrate the charm of data visualization? It allows us to intuitively feel the characteristics and changes of data.
Adding Labels to Histograms
If you want to make your histogram more informative, why not add a numerical label to each bin? This not only makes your chart more professional but also makes it easier for readers to understand the data. Let's see how to implement this:
import matplotlib.pyplot as plt
import numpy as np
data = np.random.randn(1000)
counts, bins, patches = plt.hist(data, bins=30, alpha=0.7)
for count, x in zip(counts, bins):
plt.text(x, count, f'{int(count)}', ha='center', va='bottom')
plt.title('Distribution of Random Data with Labels')
plt.xlabel('Value')
plt.ylabel('Frequency')
plt.show()
See that? We used the plt.text()
function to add a label to each bin. The ha='center'
and va='bottom'
parameters ensure that the label is centered just above each bin.
This technique is very useful in practical work. For example, when analyzing user age distribution, this type of labeled histogram can give you an at-a-glance view of the number of users in each age group.
Line Graph: A Great Helper for Trend Analysis
After discussing histograms, let's look at another common type of chart - the line graph. Line graphs are particularly suitable for showing trends in data changes. Let's illustrate with a simple example:
import matplotlib.pyplot as plt
import numpy as np
x = np.linspace(0, 10, 100)
y = np.sin(x)
plt.plot(x, y, label='sin(x)')
plt.title('Sine Function Graph')
plt.xlabel('x')
plt.ylabel('y')
plt.legend()
plt.grid(True)
plt.show()
This code draws a graph of the sine function. plt.legend()
adds a legend, and plt.grid(True)
adds grid lines. These small details can make your chart clearer and easier to read.
In practical work, line graphs are often used to show data that changes over time, such as stock prices or website traffic. You can try replacing the sine function here with your own data to see what kind of graph you get.
Advanced Techniques
Now, let's explore some more advanced techniques that can make your charts more professional and beautiful.
Adjusting Axis Position
Sometimes, we might need to change the position of the axes to highlight certain data features. For example, moving the x-axis to the top of the graph:
import matplotlib.pyplot as plt
import numpy as np
x = np.linspace(0, 10, 100)
y = np.sin(x)
fig, ax = plt.subplots()
ax.plot(x, y)
ax.xaxis.set_ticks_position('top')
ax.xaxis.set_label_position('top')
ax.set_xlabel('x (top)')
ax.set_ylabel('sin(x)')
ax.set_title('Sine Function Graph with x-axis at the Top')
plt.show()
This technique is particularly useful in certain special scenarios. For example, when you want to emphasize certain important markers on the x-axis, moving the x-axis to the top can make these markers more prominent.
Using Custom Backgrounds
Sometimes, to make your chart more eye-catching, you might want to use an image as a background. Sounds cool, right? Let's see how to implement it:
import matplotlib.pyplot as plt
import matplotlib.image as mpimg
import numpy as np
img = mpimg.imread('background.png')
x = np.linspace(0, 10, 100)
y = np.sin(x)
fig, ax = plt.subplots(figsize=(10, 6))
ax.imshow(img, extent=[0, 10, -1, 1], aspect='auto', alpha=0.5)
ax.plot(x, y, color='red', linewidth=2)
ax.set_title('Sine Function with Background Image')
ax.set_xlabel('x')
ax.set_ylabel('sin(x)')
plt.show()
This code first loads an image named 'background.png' as the background, then draws the sine function graph on top of it. The extent
parameter defines the range of the background image, and the alpha
parameter controls the transparency of the background.
This technique can make your charts more personalized. Imagine if you're doing a data analysis on marine life, using an ocean background image would make your chart more thematic. Isn't that creative?
Adjusting Transparency and Color
Adjusting colors and transparency can make your charts more beautiful while also conveying more information. Let's look at an example:
import matplotlib.pyplot as plt
import numpy as np
x = np.linspace(0, 10, 100)
y1 = np.sin(x)
y2 = np.cos(x)
plt.figure(figsize=(10, 6))
plt.plot(x, y1, color='blue', alpha=0.7, linewidth=2, label='sin(x)')
plt.plot(x, y2, color='red', alpha=0.5, linewidth=2, label='cos(x)')
plt.fill_between(x, y1, y2, where=(y1 > y2), color='green', alpha=0.3)
plt.fill_between(x, y1, y2, where=(y1 <= y2), color='yellow', alpha=0.3)
plt.title('Sine and Cosine Functions')
plt.xlabel('x')
plt.ylabel('y')
plt.legend()
plt.grid(True, alpha=0.3)
plt.show()
This code draws graphs of sine and cosine functions and fills the areas between them with different colors. By adjusting the alpha
parameter, we can control the transparency of the lines and filled areas, making the chart clearer and easier to read.
This technique is particularly useful when comparing multiple data series. For example, when analyzing sales trends of different products, using different colors and transparencies can make the data for each product easier to distinguish.
Practical Case Analysis
Now, let's use a practical case to apply the knowledge we've learned comprehensively. Suppose we have a set of student score data, and we want to analyze and visualize this data.
import matplotlib.pyplot as plt
import numpy as np
np.random.seed(42)
scores = np.random.normal(70, 15, 200).clip(0, 100)
plt.figure(figsize=(12, 6))
counts, bins, patches = plt.hist(scores, bins=20, alpha=0.7, color='skyblue', edgecolor='black')
for count, x in zip(counts, bins):
plt.text(x, count, f'{int(count)}', ha='center', va='bottom')
plt.title('Distribution of Student Scores', fontsize=16)
plt.xlabel('Score', fontsize=12)
plt.ylabel('Number of Students', fontsize=12)
mean_score = np.mean(scores)
median_score = np.median(scores)
plt.axvline(mean_score, color='red', linestyle='dashed', linewidth=2, label=f'Mean: {mean_score:.2f}')
plt.axvline(median_score, color='green', linestyle='dashed', linewidth=2, label=f'Median: {median_score:.2f}')
plt.legend()
plt.grid(True, alpha=0.3)
plt.show()
This code does the following:
- Generates 200 simulated student score data points.
- Draws a histogram to show the distribution of scores.
- Adds numerical labels to each bin.
- Adds vertical lines for the mean and median.
- Sets the title, axis labels, and legend.
- Adds grid lines to improve readability.
Through this chart, we can intuitively see:
- The overall distribution of scores
- The number of students in each score range
- The positions of the mean and median
This visualization method can help teachers quickly understand the overall performance of the class and identify student groups that may need extra attention. For example, if they find that many students are concentrated in the low score range, they might need to consider adjusting teaching methods or providing additional tutoring.
Summary and Outlook
Through this blog post, we started from the basics of Matplotlib, gradually delved into some advanced techniques, and finally applied this knowledge comprehensively through a practical case. I hope this content can spark your interest in data visualization and help you create more beautiful and informative charts in your actual work.
Remember, data visualization is not just a technique, but also an art. It requires constant practice and trying new methods and techniques. Every time you see that the chart you created can clearly convey complex information, that sense of achievement is unparalleled.
So, are you ready to start your data visualization journey? Why not start with what you learned today and create some charts with your own data. You might discover some interesting techniques that we didn't mention today. Feel free to share your discoveries and thoughts in the comments section.
Finally, let's look forward to the future of Python data visualization together. With the continuous development of technology, I believe we will have more powerful tools and cooler visualization methods. Maybe one day, we can even create AI assistants that can automatically understand data and generate the best visualization solutions. What an exciting moment that would be!
Alright, that's all for today's sharing. I hope you've gained some inspiration from it and will go further on your own data visualization journey. Remember, practice makes perfect, and only by trying more can you truly master these techniques. I look forward to seeing your works and ideas in the comments section. See you next time!
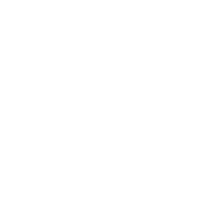
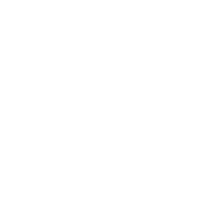