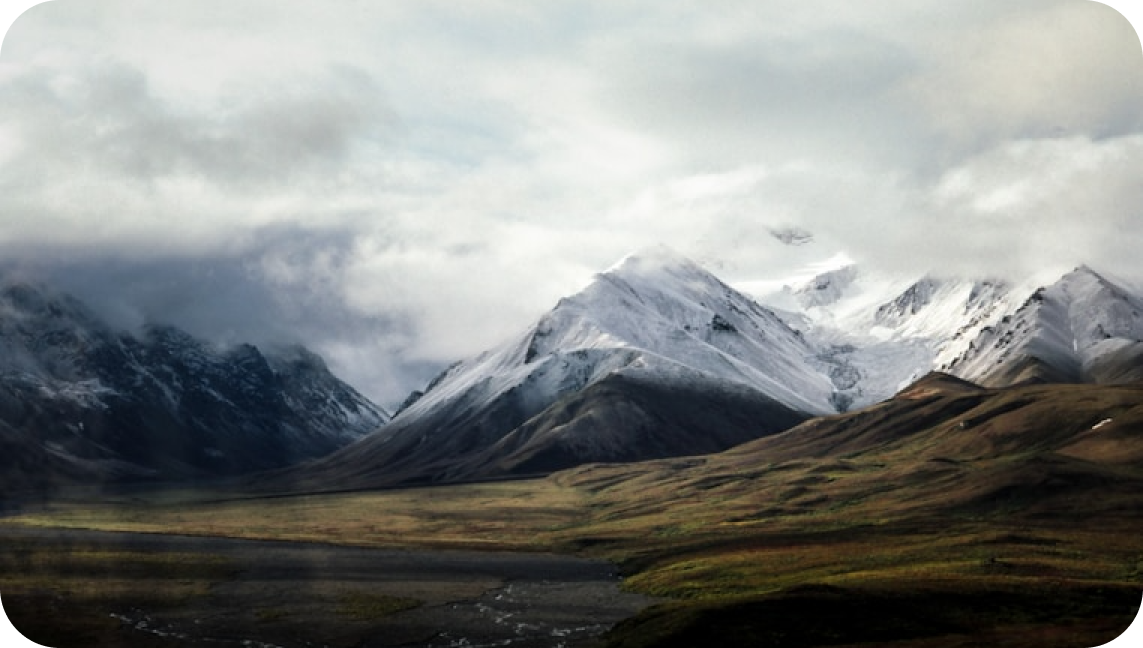
Hello everyone, while helping friends with data visualization issues recently, I've noticed many people have confusion about choosing and using Python visualization tools. Are you also often troubled by these questions: which visualization library should you choose? How to make charts more professional and aesthetically pleasing? How to handle visualization needs for large-scale data? Let's explore these questions together today.
The Challenge of Tool Selection
I remember hesitating for a long time when choosing visualization tools for a data analysis project last year. I had to think carefully about the three mainstream options: Matplotlib, Seaborn, and Plotly. Looking back now, this selection process actually helped me gain a deeper understanding of these tools.
Matplotlib is like a Swiss Army knife - it can do everything, but doing it well requires considerable effort. When I first used it, I spent a long time just adjusting font sizes and positions. However, this "low-level" nature makes it particularly suitable for scenarios with high customization requirements. I especially rely on its flexibility when handling specialized scientific data visualization.
For example, this code can generate a basic but professional chart:
import matplotlib.pyplot as plt
import numpy as np
x = np.linspace(0, 10, 1000)
y1 = np.sin(x)
y2 = np.cos(x)
plt.style.use('seaborn')
plt.figure(figsize=(12, 6))
plt.plot(x, y1, label='Sin(x)', linewidth=2)
plt.plot(x, y2, label='Cos(x)', linewidth=2)
plt.title('Trigonometric Functions', fontsize=16)
plt.xlabel('x', fontsize=12)
plt.ylabel('y', fontsize=12)
plt.legend(fontsize=12)
plt.grid(True, alpha=0.3)
plt.show()
Seaborn is like a thoughtful assistant, packaging many commonly used statistical charts and coming with pleasing default color schemes. I frequently use it in daily data analysis work, especially when I need to quickly generate statistical charts.
For instance, when I need to quickly analyze the distribution of data, Seaborn's violinplot is particularly useful:
import seaborn as sns
import pandas as pd
np.random.seed(0)
data = pd.DataFrame({
'group': np.repeat(['A', 'B', 'C', 'D'], 250),
'values': np.concatenate([
np.random.normal(0, 1, 250),
np.random.normal(2, 1.5, 250),
np.random.normal(-1, 2, 250),
np.random.normal(3, 0.5, 250)
])
})
plt.figure(figsize=(12, 6))
sns.violinplot(x='group', y='values', data=data, inner='box')
plt.title('Distribution of Values Across Groups', fontsize=16)
plt.xlabel('Group', fontsize=12)
plt.ylabel('Values', fontsize=12)
plt.show()
Plotly represents modern data visualization, capable of generating interactive charts, which is particularly useful for data presentation. I remember once creating a sales data dashboard with it, and when presenting to clients, they could zoom and view specific values directly on the charts. This interactive experience left a deep impression on the clients.
Deep Thoughts
In practical applications, I've found that choosing visualization tools is actually about balancing several dimensions:
- Development efficiency vs. Customization flexibility
- Performance vs. Visual aesthetics
- Interactive experience vs. Output portability
For example, last year when I was working on a financial data analysis project, I needed to visualize over 1 million transaction records. Using Matplotlib directly to create scatter plots would have performed poorly. Later, I adopted this strategy:
import numpy as np
import pandas as pd
import matplotlib.pyplot as plt
from scipy.stats import gaussian_kde
def plot_density_scatter(x, y, sample_size=10000):
# Downsample if data volume is too large
if len(x) > sample_size:
idx = np.random.choice(len(x), sample_size, replace=False)
x = x[idx]
y = y[idx]
# Calculate point density
xy = np.vstack([x,y])
z = gaussian_kde(xy)(xy)
# Draw scatter plot, color indicates density
plt.scatter(x, y, c=z, s=20, alpha=0.5)
plt.colorbar(label='Density')
n_points = 1000000
x = np.random.normal(0, 1, n_points)
y = x * 0.5 + np.random.normal(0, 0.5, n_points)
plt.figure(figsize=(10, 8))
plot_density_scatter(x, y)
plt.title('Large Dataset Visualization with Density', fontsize=14)
plt.xlabel('X', fontsize=12)
plt.ylabel('Y', fontsize=12)
plt.show()
Practical Experience
Through years of practice, I've summarized several points of experience that I hope will be helpful:
- Data preprocessing is crucial
Before visualization, it's essential to do proper data cleaning and preprocessing. For example, handling outliers:
def preprocess_for_viz(df, columns):
df_clean = df.copy()
for col in columns:
# Calculate IQR
Q1 = df[col].quantile(0.25)
Q3 = df[col].quantile(0.75)
IQR = Q3 - Q1
# Set boundaries
lower_bound = Q1 - 1.5 * IQR
upper_bound = Q3 + 1.5 * IQR
# Handle outliers
df_clean.loc[df_clean[col] > upper_bound, col] = upper_bound
df_clean.loc[df_clean[col] < lower_bound, col] = lower_bound
return df_clean
- Color schemes should be professional
I now habitually use predefined professional color schemes:
color_palette = {
'primary': ['#2C3E50', '#E74C3C', '#ECF0F1', '#3498DB', '#2ECC71'],
'sequential': ['#f7fbff', '#deebf7', '#c6dbef', '#9ecae1', '#6baed6'],
'diverging': ['#d73027', '#f46d43', '#fdae61', '#fee090', '#ffffbf']
}
plt.style.use('seaborn')
fig, axes = plt.subplots(1, 3, figsize=(15, 5))
for i, (name, colors) in enumerate(color_palette.items()):
data = np.random.randn(5)
axes[i].bar(range(5), data, color=colors)
axes[i].set_title(f'{name.capitalize()} Color Scheme')
- Pay attention to details
Chart details determine professionalism. For example, I often use this function to beautify charts:
def style_chart(ax, title, xlabel, ylabel):
# Set title and labels
ax.set_title(title, fontsize=14, pad=20)
ax.set_xlabel(xlabel, fontsize=12)
ax.set_ylabel(ylabel, fontsize=12)
# Set grid lines
ax.grid(True, linestyle='--', alpha=0.7)
# Set borders
ax.spines['top'].set_visible(False)
ax.spines['right'].set_visible(False)
# Set ticks
ax.tick_params(labelsize=10)
return ax
Future Outlook
Have you thought about how data visualization technology will develop in the future? I think several trends are worth watching:
- Real-time visualization will become more prevalent. Much data is now generated in real-time, and handling real-time data visualization elegantly is an important topic:
import matplotlib.animation as animation
def create_live_plot(data_generator):
fig, ax = plt.subplots(figsize=(10, 6))
line, = ax.plot([], [])
def init():
ax.set_xlim(0, 100)
ax.set_ylim(-1, 1)
return line,
def update(frame):
data = next(data_generator)
line.set_data(range(len(data)), data)
return line,
ani = animation.FuncAnimation(fig, update, init_func=init,
interval=100, blit=True)
return ani
def data_generator():
data = []
while True:
data.append(np.random.normal())
if len(data) > 100:
data.pop(0)
yield data
ani = create_live_plot(data_generator())
plt.show()
- 3D visualization will become more important. With increasing data dimensions, displaying multidimensional data in limited 2D space is a challenge:
from mpl_toolkits.mplot3d import Axes3D
def plot_3d_scatter(x, y, z, colors=None):
fig = plt.figure(figsize=(12, 8))
ax = fig.add_subplot(111, projection='3d')
scatter = ax.scatter(x, y, z, c=colors, cmap='viridis')
ax.set_xlabel('X axis')
ax.set_ylabel('Y axis')
ax.set_zlabel('Z axis')
plt.colorbar(scatter)
return fig, ax
- Automation and intelligence in visualization. More AI-based visualization recommendation systems might emerge in the future, helping users automatically select the most suitable chart types and styles.
What are your thoughts on the future of Python data visualization? Feel free to share your views and experiences in the comments.
Remember, data visualization is not just a technology, but also an art. It requires us to find the perfect balance between technical implementation and visual presentation. I hope this article brings you some inspiration, and let's continue to explore and progress together in this field.
Next
Mastering Data Visualization in Python: A Complete Guide to Matplotlib
A comprehensive guide exploring data visualization fundamentals in Python, covering core concepts, visualization types, and practical implementations using popular libraries like Matplotlib, Seaborn, and Plotly, with detailed examples and use cases
Overview of Python Data Visualization
Explore the field of Python data visualization, introducing the characteristics and applications of mainstream libraries such as Matplotlib, Bokeh, and Holoviz.
The Art and Practice of Python Data Visualization
Discuss the importance and practical methods of Python data visualization, introduce common libraries such as Matplotlib and Plotnine, and use StackOverflow data as an example to explain in detail the steps of data acquisition, preprocessing, basic statistics, correlation analysis, and time series analysis, demonstrating the powerful role of data visualization in revealing data patterns and insights
Next
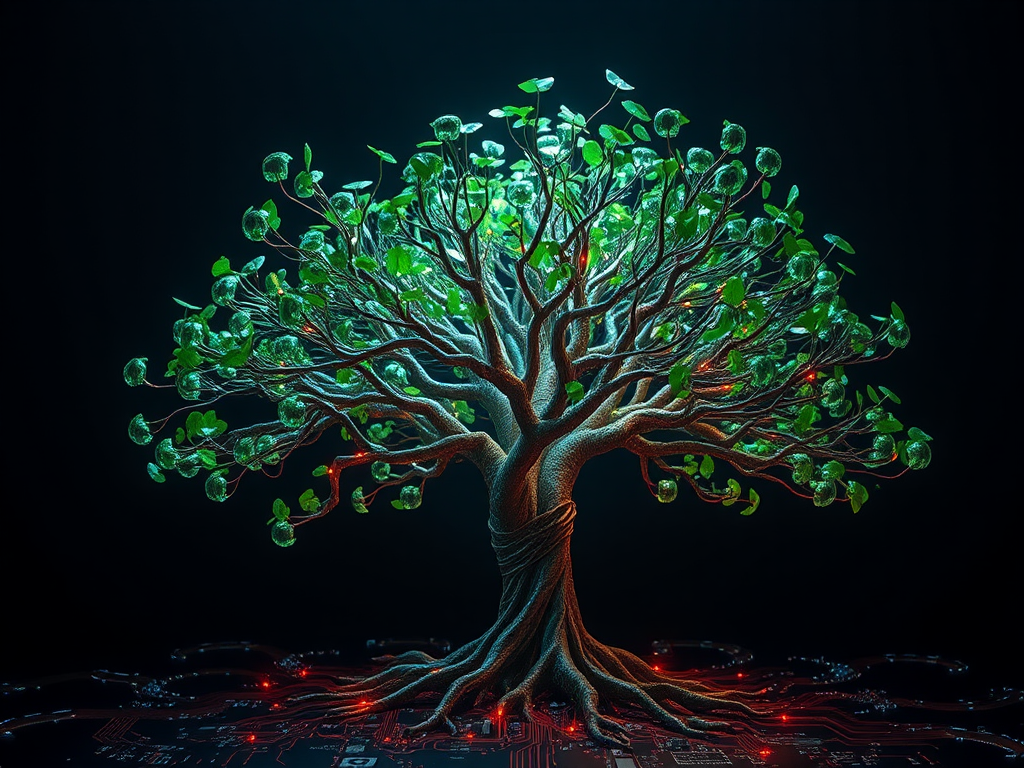
Mastering Data Visualization in Python: A Complete Guide to Matplotlib
A comprehensive guide exploring data visualization fundamentals in Python, covering core concepts, visualization types, and practical implementations using popular libraries like Matplotlib, Seaborn, and Plotly, with detailed examples and use cases
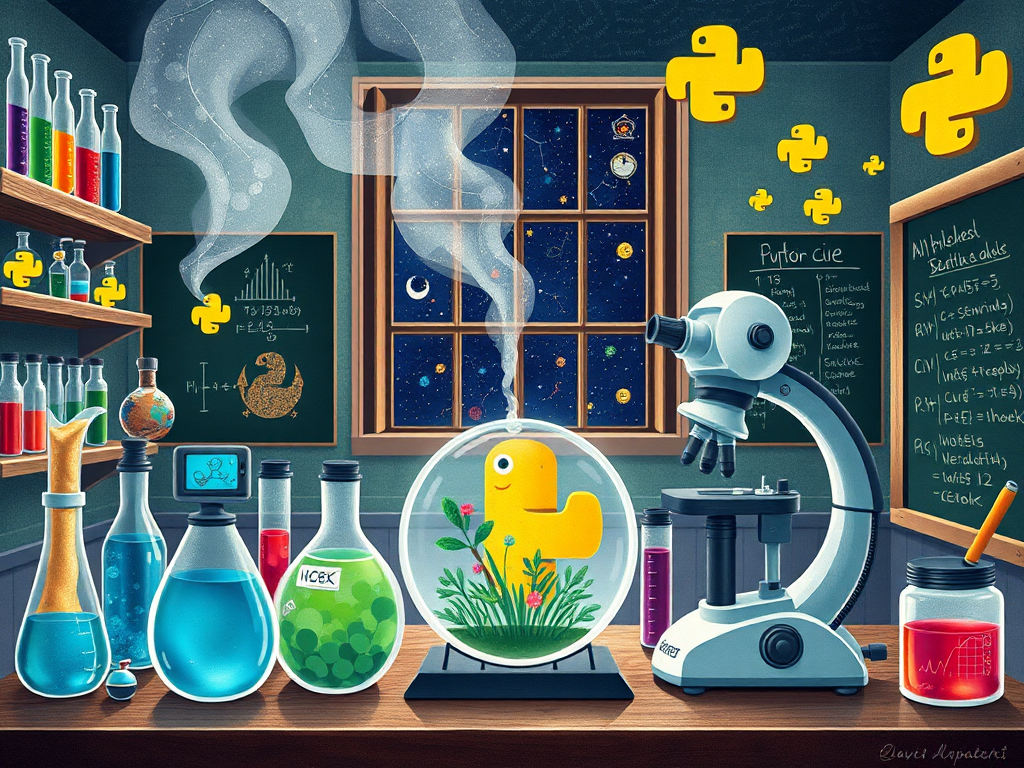
Overview of Python Data Visualization
Explore the field of Python data visualization, introducing the characteristics and applications of mainstream libraries such as Matplotlib, Bokeh, and Holoviz.
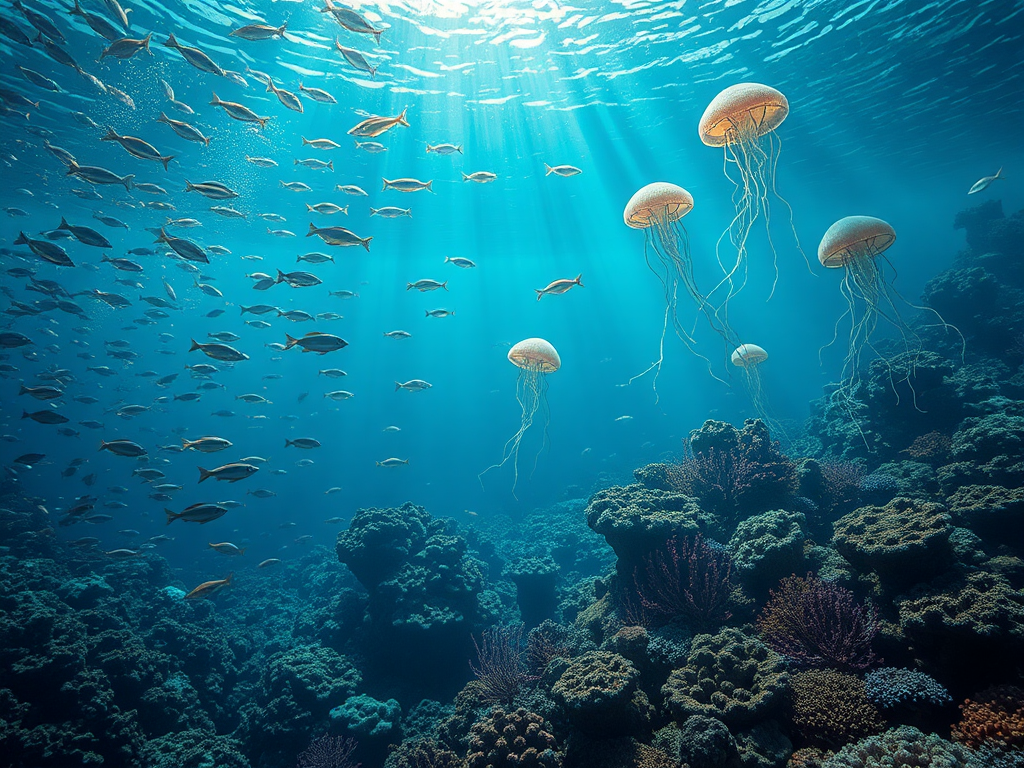
The Art and Practice of Python Data Visualization
Discuss the importance and practical methods of Python data visualization, introduce common libraries such as Matplotlib and Plotnine, and use StackOverflow data as an example to explain in detail the steps of data acquisition, preprocessing, basic statistics, correlation analysis, and time series analysis, demonstrating the powerful role of data visualization in revealing data patterns and insights