Background
Have you ever been in a situation where you have a large amount of data but don't know how to turn it into intuitive charts? Or spent a lot of time coding visualizations only to get unsatisfactory results? As a Python enthusiast, I deeply understand both the importance and challenges of data visualization. Today, let's explore the secrets of Python data visualization together.
Basics
When it comes to Python data visualization, we must mention Matplotlib, the fundamental library. It's like a Swiss Army knife - while it might not seem trendy, it's one of the most reliable tools available. I remember finding Matplotlib's syntax a bit cumbersome when I first started, but as I used it more, I discovered its power and flexibility.
Let's start with a simple example:
import matplotlib.pyplot as plt
import numpy as np
x = np.linspace(0, 10, 100)
y = np.sin(x)
plt.figure(figsize=(10, 6))
plt.plot(x, y, 'b-', label='sin(x)')
plt.title('Sine Wave')
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.grid(True)
plt.legend()
plt.show()
Would you like me to explain or break down this code?
Advanced Topics
Once you've mastered basic plotting, you'll discover that data visualization goes far beyond this. For instance, we often need to display multiple data series on one graph or create subplots to compare different data characteristics.
Here's a more complex example:
import matplotlib.pyplot as plt
import numpy as np
x = np.linspace(0, 10, 100)
y1 = np.sin(x)
y2 = np.cos(x)
y3 = np.tan(x)
fig, axes = plt.subplots(2, 2, figsize=(12, 10))
axes[0, 0].plot(x, y1, 'r-', label='sin(x)')
axes[0, 0].plot(x, y2, 'b--', label='cos(x)')
axes[0, 0].set_title('Trigonometric Functions Comparison')
axes[0, 0].legend()
axes[0, 0].grid(True)
axes[0, 1].scatter(y1, y2, c=x, cmap='viridis')
axes[0, 1].set_title('Correlation between sin(x) and cos(x)')
axes[0, 1].grid(True)
axes[1, 0].hist(y1, bins=30, alpha=0.5, label='sin(x)')
axes[1, 0].hist(y2, bins=30, alpha=0.5, label='cos(x)')
axes[1, 0].set_title('Value Distribution')
axes[1, 0].legend()
axes[1, 1].fill_between(x, y1, y2, alpha=0.3)
axes[1, 1].set_title('Area between sin(x) and cos(x)')
plt.tight_layout()
plt.show()
Would you like me to explain or break down this code?
Practical Application
In real-world scenarios, we often need to handle more complex data visualization requirements. For example, I recently worked on a stock data analysis project that needed to show stock price trends, trading volume, moving averages, and other indicators. This is where Matplotlib's flexibility becomes particularly important.
Let's look at an example of stock data visualization:
import matplotlib.pyplot as plt
import numpy as np
import pandas as pd
dates = pd.date_range(start='2023-01-01', end='2023-12-31', freq='D')
np.random.seed(42)
price = 100 + np.random.randn(len(dates)).cumsum()
volume = np.random.randint(1000, 5000, size=len(dates))
ma5 = pd.Series(price).rolling(window=5).mean()
ma20 = pd.Series(price).rolling(window=20).mean()
fig, (ax1, ax2) = plt.subplots(2, 1, figsize=(12, 8), height_ratios=[3, 1])
ax1.plot(dates, price, 'b-', label='Price', alpha=0.6)
ax1.plot(dates, ma5, 'r-', label='5-day MA')
ax1.plot(dates, ma20, 'g-', label='20-day MA')
ax1.set_title('Stock Price Trend')
ax1.set_ylabel('Price')
ax1.legend()
ax1.grid(True)
ax2.bar(dates, volume, color='gray', alpha=0.5)
ax2.set_title('Trading Volume')
ax2.set_ylabel('Volume')
plt.tight_layout()
plt.show()
Would you like me to explain or break down this code?
Insights
Through years of practice, I've summarized several key points about data visualization:
-
Clarity First: The primary purpose of charts is to convey information. Don't sacrifice readability for aesthetics. I've seen many cases where pursuit of visual effects made charts harder to understand.
-
Moderate Customization: Matplotlib offers rich customization options, but not all need to be used. It's wise to adjust based on actual needs.
-
Color Schemes: Choosing appropriate color schemes is important. I usually use high-contrast colors to distinguish different data series while considering colorblind-friendly schemes.
-
Chart Types: Selecting the right chart type is crucial. For instance, line charts are usually best for time series data, while bar charts might be more suitable for comparing different categories.
-
Interactive Considerations: If your charts need to be displayed on web pages, consider using interactive libraries like Plotly. However, for reports or papers, static chart libraries like Matplotlib are recommended.
Future Outlook
The field of Python data visualization is rapidly evolving. Besides Matplotlib, there are many excellent visualization libraries worth noting:
- Seaborn: A statistical visualization library based on Matplotlib, offering more advanced plotting interfaces
- Plotly: A library for creating interactive charts, especially suitable for web display
- Bokeh: Another powerful interactive visualization library
- Altair: A declarative visualization library based on Vega and Vega-Lite
Which library do you prefer for data visualization? Feel free to share your experience in the comments.
Finally, I want to say that data visualization isn't just a technology, it's an art. It requires continuous learning and practice to truly master its essence. What do you think?
Summary
Today we've explored various aspects of Python data visualization, from basic Matplotlib usage to practical cases. Data visualization is an essential part of data analysis, and mastering this tool will make your data analysis work much more efficient.
I hope this article has been helpful. If you have any questions or suggestions, feel free to leave a comment. Let's continue advancing together on the path of data visualization.
What difficulties do you commonly encounter in data visualization? Or do you have any unique solutions? Looking forward to hearing your thoughts.
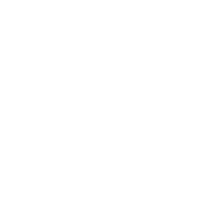
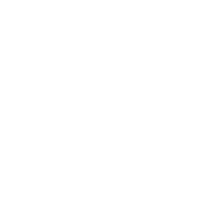
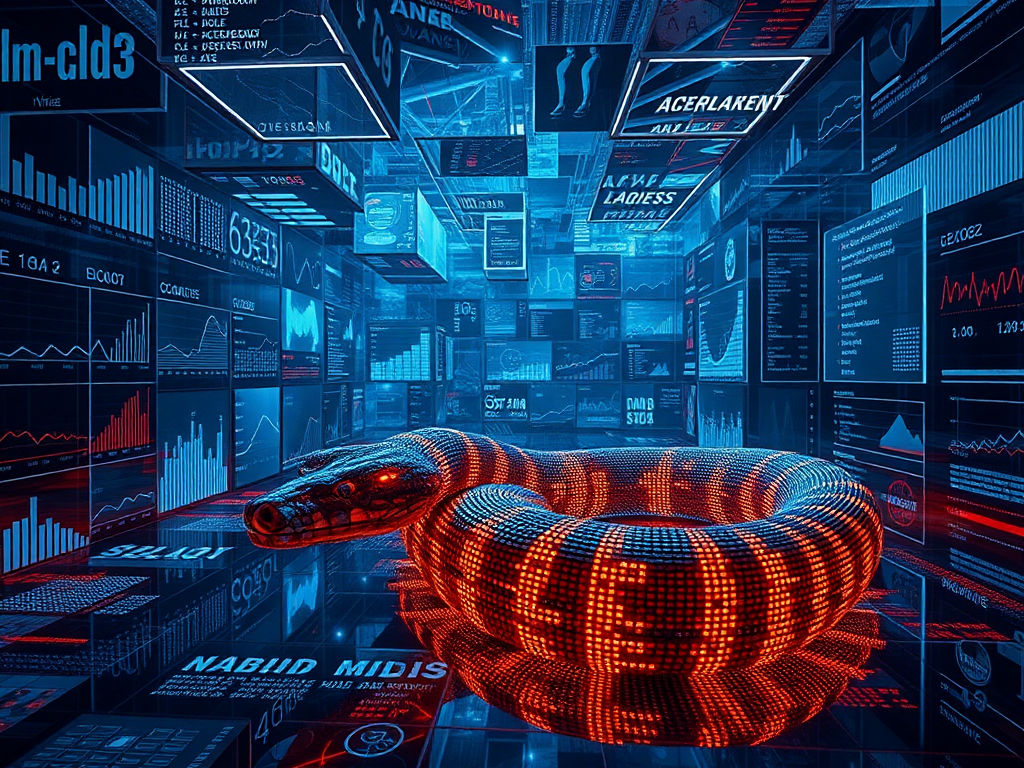
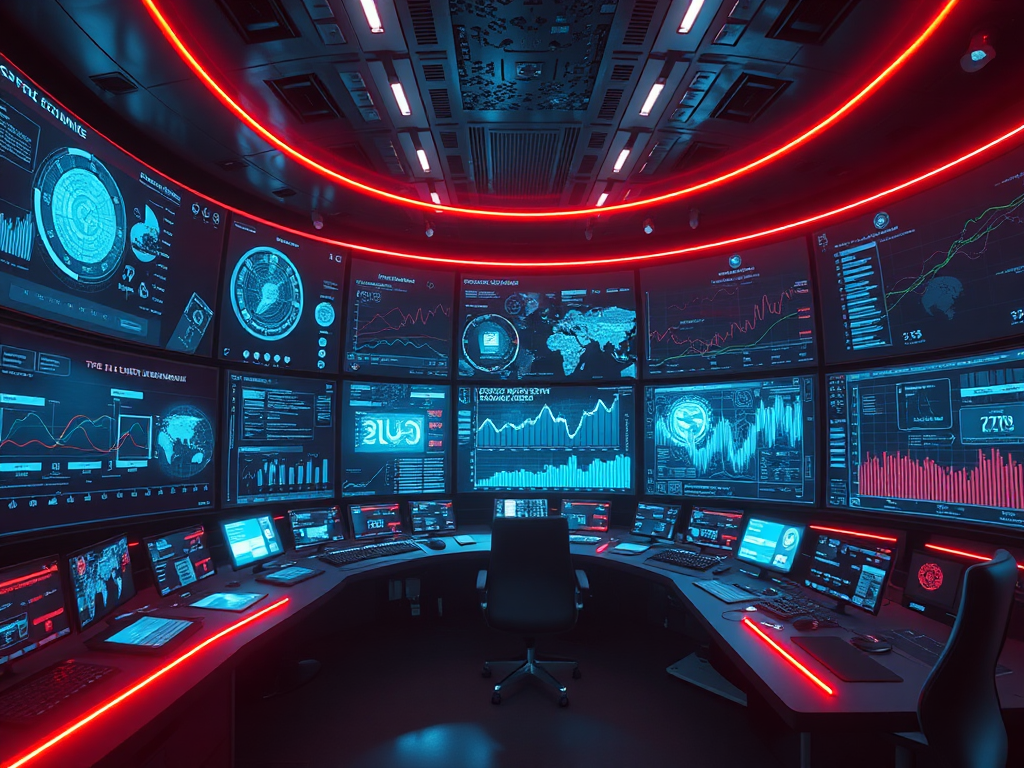
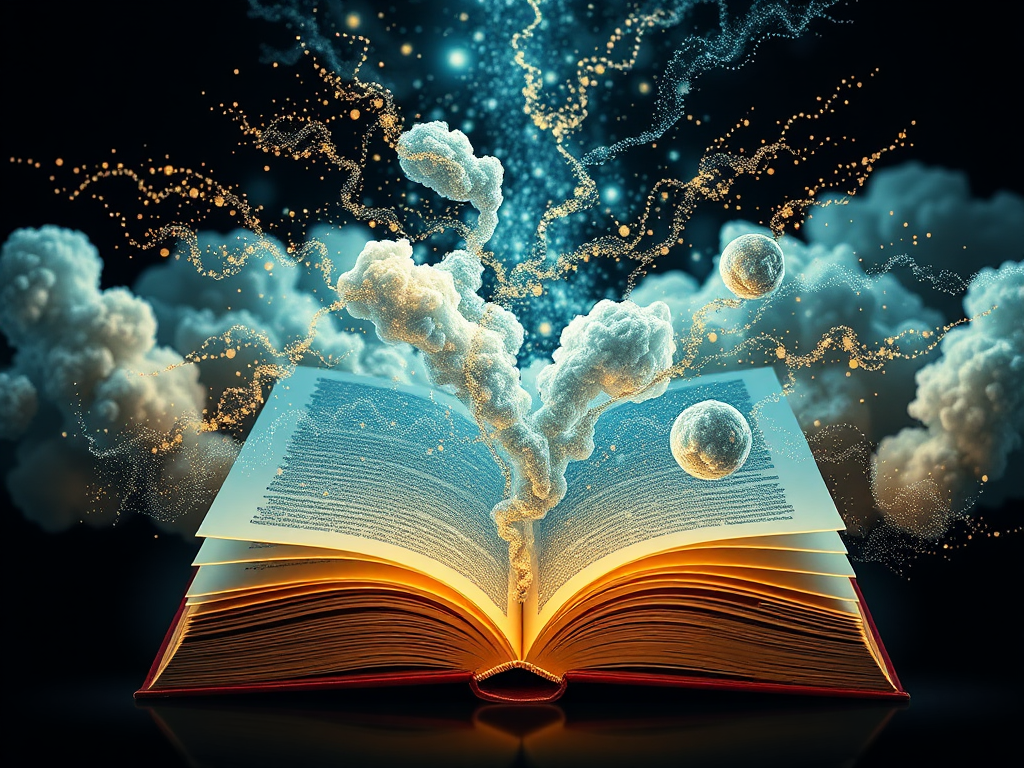