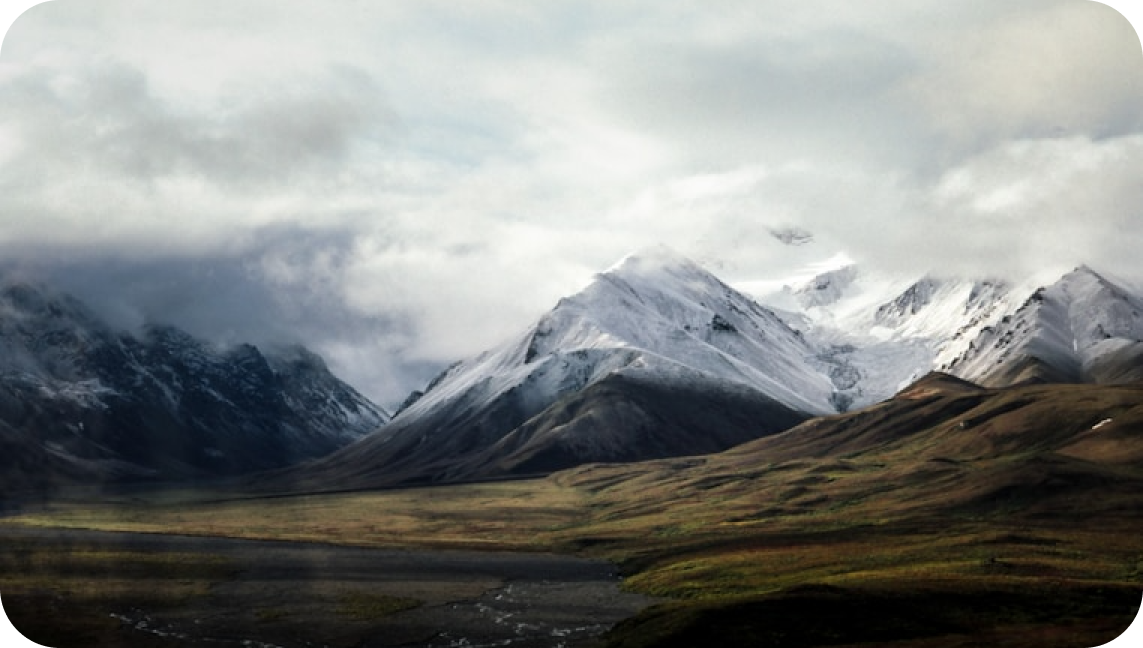
Introduction
Have you ever felt frustrated that despite mastering basic Python programming, you still can't create those eye-catching data visualizations? Or do you often struggle with choosing the right chart type? Today, let's explore how to use Matplotlib, this powerful tool, to create professional and elegant data visualizations.
Basics
When it comes to data visualization, many people's first thought is "drawing a chart with Matplotlib." But did you know? Real data visualization goes far beyond that. In my years of teaching experience, I've found that many learners often stay at the API-calling level while overlooking the design thinking behind visualization.
Let's start with the most basic concepts. Matplotlib is Python's most fundamental and important visualization library - it's like a canvas, and we are the artists on this canvas. I often tell my students: "Mastering Matplotlib is like learning the fundamentals of painting."
import matplotlib.pyplot as plt
import numpy as np
plt.figure(figsize=(10, 6))
x = np.linspace(0, 10, 100)
y = np.sin(x)
plt.plot(x, y, label='sin(x)', color='#2E86C1', linewidth=2)
plt.title('Sine Function Curve', fontsize=15)
plt.xlabel('X-axis', fontsize=12)
plt.ylabel('Y-axis', fontsize=12)
plt.grid(True, linestyle='--', alpha=0.7)
plt.legend(fontsize=10)
plt.savefig('sine_wave.png', dpi=300, bbox_inches='tight')
plt.show()
Advanced Level
After mastering the basics, let's talk about how to enhance the professionalism of charts. I remember once when I was conducting data analysis training for a financial company, their requirement was to show stock price trends. Most people might just draw a simple line chart, but professional data visualization requires consideration of more details.
import matplotlib.pyplot as plt
import numpy as np
from matplotlib.ticker import FuncFormatter
plt.rcParams['font.sans-serif'] = ['SimHei']
plt.rcParams['axes.unicode_minus'] = False
dates = pd.date_range(start='2023-01-01', end='2023-12-31', freq='D')
stock_prices = np.random.normal(loc=100, scale=10, size=len(dates))
stock_prices = np.maximum(0, stock_prices.cumsum())
fig, ax = plt.subplots(figsize=(15, 8))
ax.plot(dates, stock_prices, color='#2E86C1', linewidth=2)
ax.set_facecolor('#f8f9fa')
plt.grid(True, linestyle='--', alpha=0.3)
def currency_formatter(x, p):
return f'¥{x:,.2f}'
ax.yaxis.set_major_formatter(FuncFormatter(currency_formatter))
plt.xticks(rotation=45)
plt.title('2023 Stock Price Trend', fontsize=16, pad=20)
plt.xlabel('Date', fontsize=12)
plt.ylabel('Stock Price', fontsize=12)
fig.text(0.99, 0.01, 'Data Source: Sample Data',
ha='right', va='bottom',
fontsize=8, color='gray', alpha=0.5)
plt.tight_layout()
plt.show()
Professional Level
As data visualization applications become more widespread, we need to master more advanced techniques. In my view, professional data visualization should have three qualities: clear data expression, reasonable visual hierarchy, and good user experience.
import matplotlib.pyplot as plt
import numpy as np
from matplotlib.gridspec import GridSpec
fig = plt.figure(figsize=(15, 10))
gs = GridSpec(2, 2, figure=fig)
ax1 = fig.add_subplot(gs[0, :])
x = np.linspace(0, 10, 100)
y1 = np.sin(x)
y2 = np.cos(x)
ax1.plot(x, y1, label='sin(x)', color='#2E86C1', linewidth=2)
ax1.plot(x, y2, label='cos(x)', color='#E74C3C', linewidth=2)
ax1.set_title('Trigonometric Function Comparison', fontsize=14)
ax1.legend()
ax1.grid(True, linestyle='--', alpha=0.3)
ax2 = fig.add_subplot(gs[1, 0])
data = np.random.normal(0, 1, 1000)
ax2.hist(data, bins=30, color='#2E86C1', alpha=0.7)
ax2.set_title('Data Distribution', fontsize=12)
ax3 = fig.add_subplot(gs[1, 1])
sizes = [30, 20, 25, 15, 10]
labels = ['A', 'B', 'C', 'D', 'E']
colors = ['#2E86C1', '#E74C3C', '#27AE60', '#F4D03F', '#8E44AD']
ax3.pie(sizes, labels=labels, colors=colors, autopct='%1.1f%%')
ax3.set_title('Proportion Analysis', fontsize=12)
plt.tight_layout()
plt.show()
Practical Application
In real work scenarios, data visualization often involves handling large amounts of real data. I remember once needing to analyze user behavior data from an e-commerce platform, with millions of raw data records. At this point, besides considering visualization aesthetics, we also need to balance code performance and efficiency.
import matplotlib.pyplot as plt
import numpy as np
import pandas as pd
from matplotlib.animation import FuncAnimation
np.random.seed(42)
n_customers = 1000000
purchase_amounts = np.random.lognormal(mean=4, sigma=1, size=n_customers)
purchase_dates = pd.date_range(start='2023-01-01', end='2023-12-31', periods=n_customers)
df = pd.DataFrame({
'date': purchase_dates,
'amount': purchase_amounts
})
monthly_stats = df.set_index('date').resample('M').agg({
'amount': ['count', 'mean', 'sum']
}).round(2)
fig, (ax1, ax2) = plt.subplots(2, 1, figsize=(15, 12))
def animate(frame):
ax1.clear()
ax2.clear()
# Slice data
data = monthly_stats.iloc[:frame+1]
# Top chart: Transaction volume trend
ax1.plot(data.index, data['amount']['count'],
color='#2E86C1', linewidth=2, marker='o')
ax1.set_title('Monthly Transaction Volume Trend', fontsize=14)
ax1.grid(True, linestyle='--', alpha=0.3)
# Bottom chart: Transaction amount trend
ax2.bar(data.index, data['amount']['sum'],
color='#27AE60', alpha=0.7)
ax2.set_title('Monthly Transaction Amount Trend (Unit: Yuan)', fontsize=14)
ax2.grid(True, linestyle='--', alpha=0.3)
# Rotate x-axis labels
plt.setp(ax1.xaxis.get_majorticklabels(), rotation=45)
plt.setp(ax2.xaxis.get_majorticklabels(), rotation=45)
plt.tight_layout()
anim = FuncAnimation(fig, animate, frames=len(monthly_stats),
interval=500, repeat=False)
plt.show()
Conclusion
Through this article, we've deeply explored how to create professional-level data visualizations using Matplotlib. From basic chart creation to advanced multi-plot layouts, and to big data visualization in practical applications, each step requires attention to detail and pursuit of perfection.
Remember, data visualization is not just technology, but also an art. It requires us to find a balance between technical implementation and visual design. What do you think? Feel free to share your thoughts and experiences in the comments.
Finally, here's a question to ponder: In your actual work, how do you choose appropriate chart types to display different types of data? And how do you balance visualization aesthetics with readability? Let's discuss together.
Next
Mastering Data Visualization in Python: A Complete Guide to Matplotlib
A comprehensive guide exploring data visualization fundamentals in Python, covering core concepts, visualization types, and practical implementations using popular libraries like Matplotlib, Seaborn, and Plotly, with detailed examples and use cases
Overview of Python Data Visualization
Explore the field of Python data visualization, introducing the characteristics and applications of mainstream libraries such as Matplotlib, Bokeh, and Holoviz.
The Art and Practice of Python Data Visualization
Discuss the importance and practical methods of Python data visualization, introduce common libraries such as Matplotlib and Plotnine, and use StackOverflow data as an example to explain in detail the steps of data acquisition, preprocessing, basic statistics, correlation analysis, and time series analysis, demonstrating the powerful role of data visualization in revealing data patterns and insights
Next
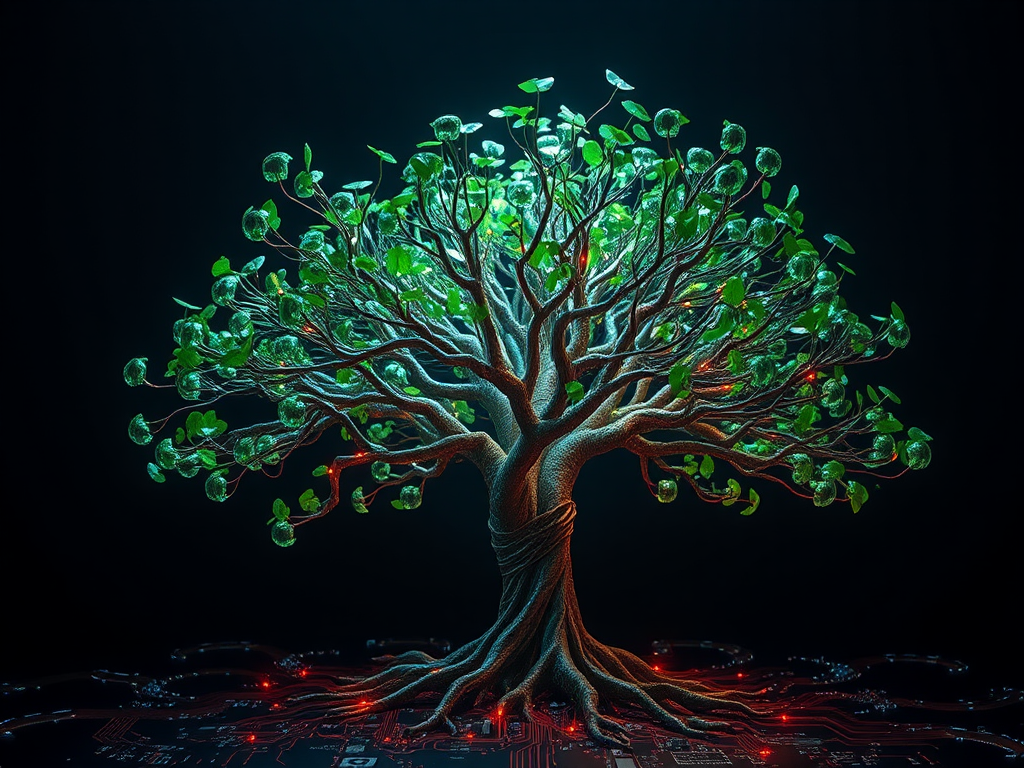
Mastering Data Visualization in Python: A Complete Guide to Matplotlib
A comprehensive guide exploring data visualization fundamentals in Python, covering core concepts, visualization types, and practical implementations using popular libraries like Matplotlib, Seaborn, and Plotly, with detailed examples and use cases
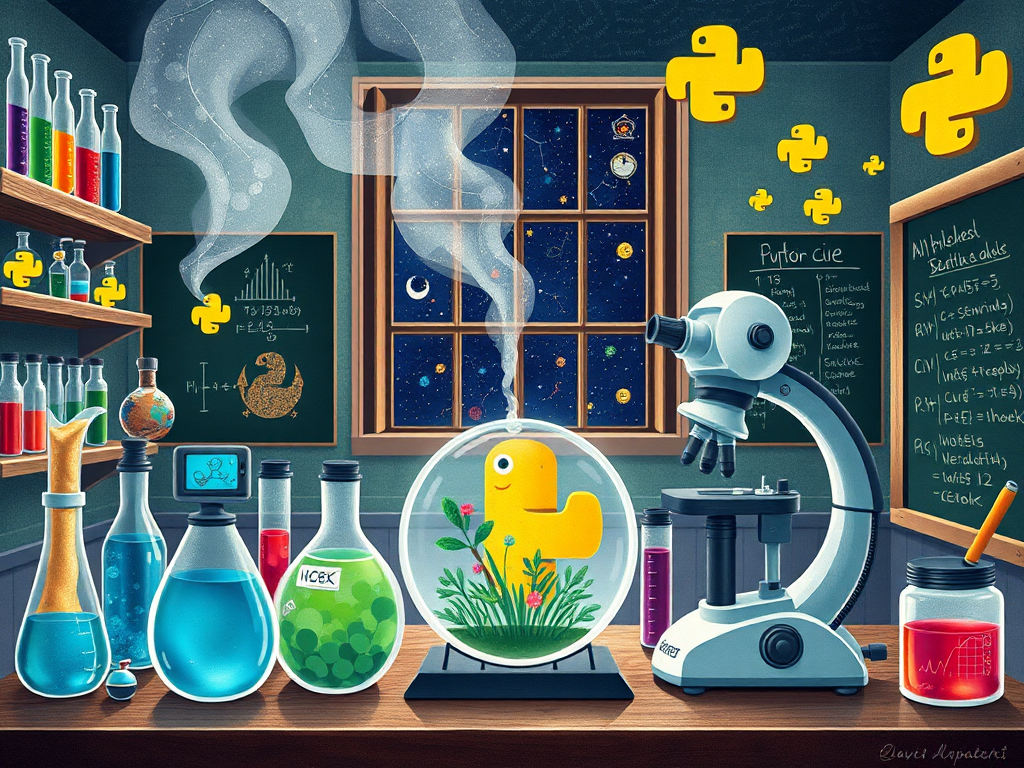
Overview of Python Data Visualization
Explore the field of Python data visualization, introducing the characteristics and applications of mainstream libraries such as Matplotlib, Bokeh, and Holoviz.
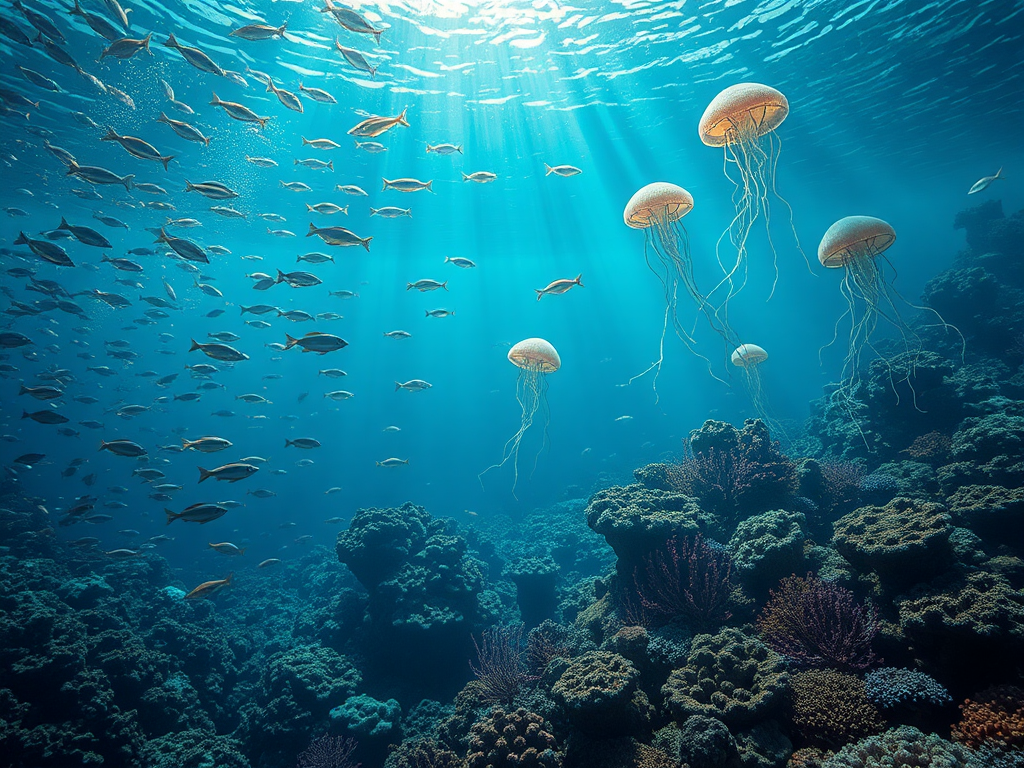
The Art and Practice of Python Data Visualization
Discuss the importance and practical methods of Python data visualization, introduce common libraries such as Matplotlib and Plotnine, and use StackOverflow data as an example to explain in detail the steps of data acquisition, preprocessing, basic statistics, correlation analysis, and time series analysis, demonstrating the powerful role of data visualization in revealing data patterns and insights