Have you ever felt overwhelmed when faced with a pile of numbers and tables? Or perhaps you have a brilliant idea but don't know how to express it through charts? Don't worry, Python data visualization is here to help! Today, let's explore the magical world of Python data visualization together and see how it can turn dull data into something vivid and interesting.
Why Visualize?
First, let's talk about why data visualization is so important. Imagine you have an Excel spreadsheet with thousands of rows of data recording your company's sales over the past year. If you were to find sales trends from this pile of numbers, it might take you several hours, and you might not even reach an accurate conclusion. However, if we plot this data into a beautiful line chart, you might be able to see the ups and downs of sales in just a few seconds.
Data visualization is magical in this way; it can make complex information intuitive and easy to understand. Not only that, good data visualization can help us discover hidden patterns and relationships in the data, providing powerful support for decision-making. You see, isn't it useful?
Python: A Powerful Ally in Visualization
So, why choose Python for data visualization? I can proudly tell you that Python is like adding wings to a tiger in this aspect! It not only has rich visualization libraries but also has simple syntax and a gentle learning curve. Whether you're a data analysis novice or an experienced data scientist, Python can meet your needs.
Python's visualization libraries are diverse and powerful. From the most basic Matplotlib to the more advanced Seaborn, to the interactive Plotly, each library has its unique advantages. You can choose the most suitable tool based on your needs and preferences. Moreover, these libraries can work together, making your data visualization more flexible and varied.
Alright, after saying all this, are you eager to get started? Don't rush, let's take it step by step, starting with the most basic Matplotlib!
Matplotlib: The "Veteran" of the Plotting World
Matplotlib can be said to be the "elder" of Python data visualization. Although it may not look as cool as emerging libraries, its functionality is the most comprehensive. From simple line charts to complex 3D charts, Matplotlib can do almost anything.
Let's first look at how to draw a simple line chart with Matplotlib:
import matplotlib.pyplot as plt
import numpy as np
x = np.linspace(0, 10, 100)
y = np.sin(x)
plt.figure(figsize=(10, 6))
plt.plot(x, y, label='sin(x)')
plt.title('Sine Function Graph', fontsize=20)
plt.xlabel('x', fontsize=14)
plt.ylabel('sin(x)', fontsize=14)
plt.legend(fontsize=12)
plt.grid(True)
plt.show()
Look, with just a few lines of code, we've drawn a beautiful sine function graph! Isn't it simple?
But Matplotlib's capabilities are far beyond this. You can also use it to draw bar charts, scatter plots, pie charts, and various other types of charts. For example, let's draw a bar chart:
import matplotlib.pyplot as plt
import numpy as np
categories = ['A', 'B', 'C', 'D', 'E']
values = [23, 35, 14, 28, 39]
plt.figure(figsize=(10, 6))
plt.bar(categories, values, color='skyblue')
plt.title('Value Comparison by Category', fontsize=20)
plt.xlabel('Category', fontsize=14)
plt.ylabel('Value', fontsize=14)
for i, v in enumerate(values):
plt.text(i, v + 0.5, str(v), ha='center')
plt.show()
This bar chart clearly shows the value comparison of each category, and we've even labeled the specific values above each bar, making the information more at a glance.
See, isn't drawing with Matplotlib interesting? But if you want more beautiful and professional statistical charts, then we need to introduce our next protagonist - Seaborn!
Seaborn: The Beauty Master of Statistical Charts
Seaborn is a statistical data visualization library based on Matplotlib. It not only provides prettier default styles but can also easily draw complex statistical charts. Drawing charts with Seaborn is like dressing up your data in beautiful clothes, making them stand out in the crowd.
Let's see how to draw a bar chart with mean and standard deviation using Seaborn:
import seaborn as sns
import matplotlib.pyplot as plt
import pandas as pd
import numpy as np
np.random.seed(0)
data = pd.DataFrame({
'group': ['A', 'B', 'C', 'D'] * 30,
'value': np.random.normal(10, 2, 120)
})
sns.set_style("whitegrid")
plt.figure(figsize=(12, 6))
sns.barplot(x='group', y='value', data=data, ci='sd', palette='cool')
plt.title('Value Comparison by Group (with Standard Deviation)', fontsize=20)
plt.xlabel('Group', fontsize=14)
plt.ylabel('Value', fontsize=14)
plt.show()
Look, this chart not only shows the average value of each group but also marks the standard deviation with error bars. Such charts are particularly useful when presenting experimental results or statistical data.
Seaborn also has a great feature that allows you to easily plot relationships between multiple variables. For example, we can use one chart to show the relationship between four variables at once:
import seaborn as sns
import matplotlib.pyplot as plt
import pandas as pd
import numpy as np
np.random.seed(0)
data = pd.DataFrame({
'x': np.random.normal(10, 2, 100),
'y': np.random.normal(10, 2, 100),
'size': np.random.randint(100, 1000, 100),
'category': np.random.choice(['A', 'B', 'C', 'D'], 100)
})
sns.set_style("darkgrid")
plt.figure(figsize=(12, 8))
sns.scatterplot(x='x', y='y', size='size', hue='category', data=data, palette='deep')
plt.title('Multivariate Relationship Chart', fontsize=20)
plt.xlabel('X Value', fontsize=14)
plt.ylabel('Y Value', fontsize=14)
plt.show()
This chart simultaneously shows the relationship between four variables: x, y, size, and category. The position of the points represents the values of x and y, the size of the points represents the value of size, and the color of the points represents the category. Isn't it cool that one chart contains so much information?
But if you want even cooler interactive charts, then you need to check out our next protagonist - Plotly!
Plotly: The Magician of Interactive Visualization
Plotly is a powerful interactive data visualization library. It not only can create various beautiful charts but also can make these charts interactive. Users can zoom in and out, hover to view detailed information, select data to display, and so on. This interactivity makes data exploration more interesting and efficient.
Let's see how to create an interactive scatter plot with Plotly:
import plotly.express as px
import pandas as pd
import numpy as np
np.random.seed(0)
data = pd.DataFrame({
'x': np.random.normal(10, 2, 100),
'y': np.random.normal(10, 2, 100),
'size': np.random.randint(5, 20, 100),
'category': np.random.choice(['A', 'B', 'C', 'D'], 100)
})
fig = px.scatter(data, x='x', y='y', size='size', color='category',
hover_data=['x', 'y', 'size', 'category'])
fig.update_layout(
title='Interactive Multivariate Scatter Plot',
xaxis_title='X Value',
yaxis_title='Y Value'
)
fig.show()
This chart may look similar to the previous scatter plot, but it's interactive! You can zoom in and out, pan the view, and even hover over points to view detailed information. Doesn't it feel like playing a game?
Plotly can also easily create animated charts. For example, we can create a dynamic bubble chart showing data from different countries changing over time:
import plotly.express as px
import pandas as pd
import numpy as np
np.random.seed(0)
countries = ['China', 'USA', 'Japan', 'Germany', 'UK']
years = range(2010, 2021)
data = []
for country in countries:
for year in years:
data.append({
'country': country,
'year': year,
'gdp': np.random.randint(1000, 20000),
'population': np.random.randint(50, 1400),
'life_expectancy': np.random.uniform(60, 85)
})
df = pd.DataFrame(data)
fig = px.scatter(df, x="gdp", y="life_expectancy", animation_frame="year",
animation_group="country", size="population", color="country",
hover_name="country", log_x=True, size_max=60,
range_x=[1000,20000], range_y=[60,85])
fig.update_layout(
title='Changes in GDP, Population, and Life Expectancy by Country (2010-2020)',
xaxis_title='GDP (Log Scale)',
yaxis_title='Life Expectancy'
)
fig.show()
This animated bubble chart shows how GDP, population, and life expectancy of different countries change over time. You can click the play button to see how the bubbles move and change over time. This dynamic display method makes the trends of data changes more intuitive.
Time Series Data: Pandas to the Rescue
Speaking of data analysis, we often encounter time series data. For example, stock prices, temperature changes, website traffic, and so on. This type of data has its peculiarities and requires special handling methods. Fortunately, Pandas provides powerful time series data processing and visualization capabilities.
Let's see how to use Pandas to process and visualize time series data:
import pandas as pd
import matplotlib.pyplot as plt
import numpy as np
dates = pd.date_range(start='2020-01-01', end='2020-12-31', freq='D')
values = np.cumsum(np.random.randn(len(dates))) + 100
df = pd.DataFrame({'date': dates, 'value': values})
df.set_index('date', inplace=True)
plt.figure(figsize=(12, 6))
df.plot(title='Value Trend in 2020', xlabel='Date', ylabel='Value')
plt.grid(True)
plt.show()
df['MA7'] = df['value'].rolling(window=7).mean()
df['MA30'] = df['value'].rolling(window=30).mean()
plt.figure(figsize=(12, 6))
df['value'].plot(label='Original Data')
df['MA7'].plot(label='7-day Moving Average')
df['MA30'].plot(label='30-day Moving Average')
plt.title('Value Trend in 2020 (with Moving Averages)')
plt.xlabel('Date')
plt.ylabel('Value')
plt.legend()
plt.grid(True)
plt.show()
This code first generates random time series data for a year, then uses Pandas' plot
method to draw a chart of the original data. Next, we calculate 7-day and 30-day moving averages and plot them together on the chart. Such charts are very common in financial analysis and can help us better understand the long-term trends of data.
See how convenient it is to handle time series data with Pandas? It not only helps us easily handle dates and times but can also directly call Matplotlib for plotting. This is the power of Python's ecosystem, where different libraries can seamlessly collaborate, making our work twice as efficient with half the effort.
ggplot Style: Elegant Statistical Charts
When it comes to data visualization, we can't ignore the ggplot2 library in R. Its unique layer grammar and elegant design style are deeply loved by data scientists and statisticians. The good news is that Python also has a similar library - plotnine, which almost completely replicates the syntax and functionality of ggplot2.
Let's see how to create a ggplot-style chart using plotnine:
from plotnine import *
import pandas as pd
import numpy as np
np.random.seed(0)
data = pd.DataFrame({
'x': np.repeat(['A', 'B', 'C', 'D'], 100),
'y': np.random.normal(10, 2, 400),
'group': np.tile(['Group1', 'Group2'], 200)
})
(ggplot(data, aes(x='x', y='y', fill='group'))
+ geom_boxplot()
+ geom_jitter(alpha=0.5, size=1)
+ labs(title='Data Distribution by Group', x='Category', y='Value')
+ theme_minimal()
+ scale_fill_brewer(palette="Set2")
)
This code creates a chart that combines a box plot and a jittered scatter plot. The box plot shows the distribution of the data, while the scatter plot displays the original data points. This combination allows us to see both the overall distribution and individual differences in the data.
The syntax of plotnine is very intuitive: we first use the ggplot
function to define the data and basic aesthetic mapping, then use the +
sign to add graphic elements, labels, themes, etc. layer by layer. This method allows us to build complex charts like building blocks, which is very flexible and powerful.
Let's look at a more complex example:
from plotnine import *
import pandas as pd
import numpy as np
np.random.seed(0)
data = pd.DataFrame({
'x': np.random.normal(10, 2, 1000),
'y': np.random.normal(10, 2, 1000),
'group': np.random.choice(['A', 'B', 'C'], 1000)
})
(ggplot(data, aes(x='x', y='y', color='group'))
+ geom_point(alpha=0.7)
+ geom_smooth(method='lm')
+ facet_wrap('~group')
+ labs(title='Data Distribution and Linear Trends by Group', x='X Value', y='Y Value')
+ theme_minimal()
+ scale_color_brewer(palette="Set1")
)
This chart shows scatter plots and linear trend lines for three groups, displayed separately using facets. This method allows us to easily compare differences between different groups.
See, aren't the charts created with plotnine both beautiful and informative? They are particularly suitable for statistical analysis and scientific papers, allowing you to easily create professional-grade charts.
Conclusion: Endless Possibilities of Data Visualization
Alright, our Python data visualization journey comes to a temporary end. We've explored from the most basic Matplotlib, to the statistical chart expert Seaborn, to the interactive visualization master Plotly, and finally learned how to handle time series data with Pandas and how to create ggplot-style charts with plotnine. Don't you feel your data visualization skills have skyrocketed?
But this is just the tip of the iceberg in the world of Python data visualization. There are many more powerful libraries waiting for us to explore, such as Folium for geographic data visualization, NetworkX for network graph visualization, Mayavi for 3D visualization, and so on. Each library has its unique advantages and applicable scenarios.
Remember, choosing the right visualization tool and method depends on your data type, analysis purpose, and target audience. Sometimes, a simple line chart might be more effective than a complex 3D chart. The key is to let your data speak and make your charts convey clear information.
Finally, I want to say that data visualization is not just a technology, but also an art. It requires us to constantly learn and practice, cultivating aesthetic ability and creativity. I hope this article can open the door to Python data visualization for you, sparking your interest and enthusiasm. Let's explore and create together in this wonderful world of data visualization!
Do you have any questions or thoughts about Python data visualization? Feel free to leave a comment, let's discuss and learn together. Remember, on the path of data visualization, we are always learners. Keep your curiosity, be brave to try, and you will surely discover more fun and magic in data visualization!
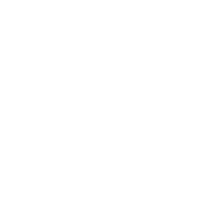
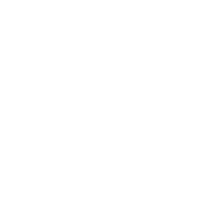