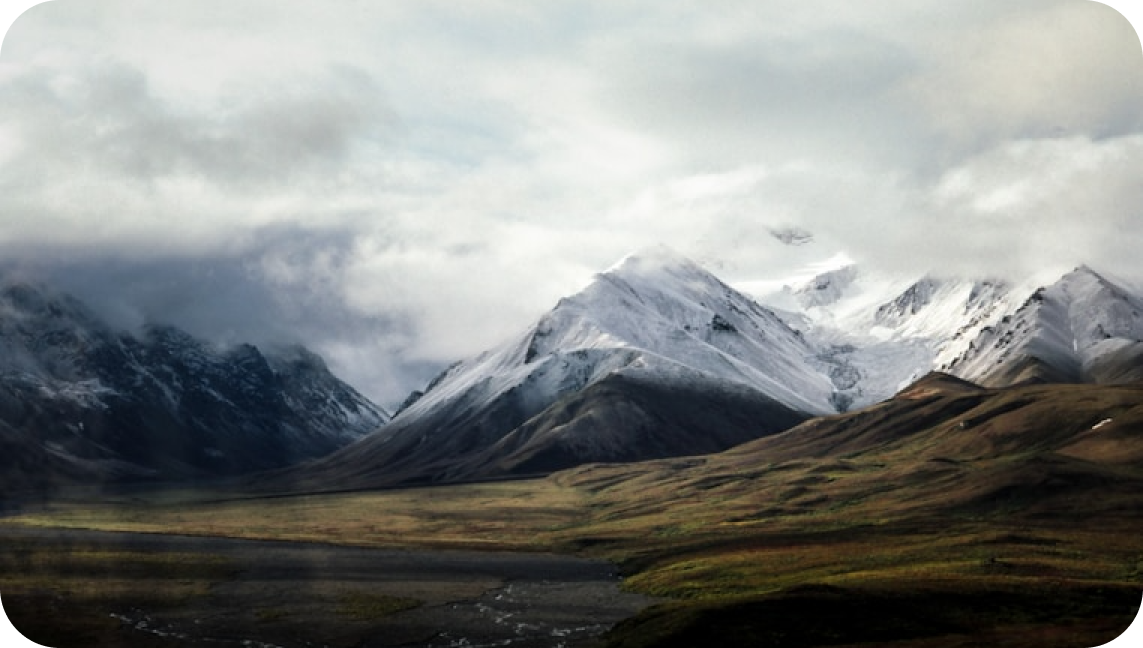
The Importance of Data Visualization
Hello friends, today we're going to discuss data visualization techniques in Python. In this era of big data, data visualization is undoubtedly an essential skill. Visualization not only helps us better understand large datasets but also allows us to discover hidden trends and patterns in the data. Therefore, mastering Python data visualization libraries has become particularly important.
Commonly Used Data Visualization Libraries
There are many powerful visualization libraries to choose from in the Python ecosystem. Let's take a look at some of the mainstream libraries.
Matplotlib
Matplotlib is definitely a "veteran" player in the field of Python visualization. As the earliest and most comprehensive plotting library, Matplotlib has always been the first choice for various data visualization tasks. It is powerful, highly extensible, supports various chart types and custom styles, allowing you to unleash your creativity and create all kinds of beautiful statistical charts.
However, Matplotlib has a steep learning curve, which can be challenging for beginners. But once you get past this threshold, Matplotlib can become your powerful assistant. You can use it to generate publication-quality charts or create dynamic graphics, 3D plots, and more.
Bokeh
Compared to Matplotlib, Bokeh focuses more on web interactive visualization. It can generate various beautiful interactive charts that can be directly embedded in web pages, making it very suitable for building modern data products and dashboards.
Bokeh has many customization options and allows you to add rich interactive functionality through callback functions. It also provides optimized support for large datasets, maintaining efficiency when handling large-scale data. Additionally, Bokeh supports interactive data exploration based on Jupyter Notebook.
Besides Matplotlib and Bokeh, there are many other excellent libraries in the Python data visualization field, such as Plotly for scientific computing, Seaborn for statistical graphics, high-performance Altair, and so on. You can choose the appropriate library based on your needs and preferences.
Basic Data Visualization Techniques
Regardless of which visualization library you use, there are several essential techniques.
Data Preparation and Preprocessing
The quality of visualization largely depends on the quality of the data. So before plotting, we need to clean and normalize the data to ensure its integrity and consistency. Pandas is a powerful data manipulation tool that can help us efficiently complete these tasks.
Choosing the Right Chart Type
Different datasets are suitable for different chart types. For example, line charts are suitable for showing how data changes over time; bar charts are better for comparing data across different categories; scatter plots are often used to show the relationship between two variables, and so on. Choosing the right chart type can make the information in the data more intuitive and persuasive.
Matplotlib in Detail
Next, let's delve into how to use Matplotlib. I'll guide you through Matplotlib's charm with some specific examples.
Basic Usage
To use Matplotlib, we first need to import the relevant module:
import matplotlib.pyplot as plt
Then we can start plotting. Here's an example of creating a simple line chart:
x = [1, 2, 3, 4, 5]
y = [2, 4, 6, 8, 10]
plt.plot(x, y)
plt.show()
This generates a basic line chart. You can beautify and customize the chart by setting various properties such as title, axis labels, legend, etc.
plt.title("A Simple Line Plot")
plt.xlabel("X Axis")
plt.ylabel("Y Axis")
plt.plot(x, y, label="Line 1")
plt.legend()
plt.show()
Customizing Chart Styles
Matplotlib allows you to fine-tune every component of the chart, including line styles, colors, marker shapes, etc. You can also set the layout, margins, background color, and other properties of the chart to create a unique personalized style.
I personally quite like this high level of customization in Matplotlib. It gives me unlimited creative space to create unique works. Of course, if you just want to quickly draw some basic charts, that's totally fine too, Matplotlib can equally meet your needs.
Advanced Features
In addition to regular 2D plotting, Matplotlib also supports some advanced features such as multiple subplots, 3D plotting, dynamic graph generation, etc.
Multiple subplots are great for displaying multiple related charts on the same canvas. You can freely combine and arrange various subplots to make data visualization more compact and clear.
Dynamic charts bring your images to "life", allowing you to display the process of data changes, making data analysis more intuitive and vivid. I often use this feature when analyzing stock data, as it allows for a clearer observation of price trends.
Bokeh Applications
After talking so much about Matplotlib, I believe you now have a deep understanding of it. Next, let's turn to Bokeh and see what unique features this web interactive visualization library has.
Interactive Map Drawing
Bokeh excels at drawing interactive maps. It has built-in support for map services like Google Maps and OpenStreetMap, allowing you to add various layers and annotations to the map.
For example, you can draw scatter points of different sizes and colors on the map to represent population density in different areas. When you zoom the map, the size and color of these scatter points will automatically adjust to fit the new zoom level. You can also add a toolbar to support basic operations like panning and zooming.
Integrating Google Maps
I remember a friend of mine encountered a problem integrating Google Maps in Bokeh. His API key and data were fine, but he just couldn't render data points on the map.
This problem is quite representative. At that time, I suggested he carefully check the data format to ensure there were no errors or missing latitude and longitude values. I also shared a sample code snippet showing how to correctly load CSV data and plot data points on Google Maps.
from bokeh.plotting import gmap
import pandas as pd
data = pd.read_csv('data.csv')
gmap = gmap("YOUR_API_KEY", gmap.google_maps.Map, zoom=10, height=600)
source = ColumnDataSource(data)
gmap.circle(x='lon', y='lat', source=source)
show(gmap)
Through this example, you should be able to grasp the basic method of embedding Google Maps in Bokeh and visualizing geographic data.
Adding Geographic Data Points
In addition to scatter plot layers, Bokeh also supports adding other data points to the map, such as lines, polygons, etc. This is very useful when displaying flight routes, area divisions, and other data.
Performance Optimization
For large-scale datasets, Bokeh provides some techniques to optimize plotting performance, such as data subsampling, using WebGL rendering, etc. This ensures smooth interactive experience, allowing you to efficiently handle large amounts of data.
Exploring the Holoviz Ecosystem
We've introduced Matplotlib and Bokeh, two major libraries. However, besides these, there's a noteworthy "rising star" in the Python data visualization field - the Holoviz ecosystem.
Holoviz is a project that integrates multiple visualization libraries, aiming to provide a unified interface and workflow. It includes several libraries such as HoloViews, Panel, GeoViews, etc., covering the entire process from exploratory data analysis to interactive dashboard construction.
Using HoloViews and Panel
HoloViews is the core of the Holoviz ecosystem, providing a declarative interface for data analysis and visualization. You can easily create various statistical charts with it, and all charts are highly composable and shareable.
Panel is an interactive dashboard library for analytical applications that seamlessly integrates the functions of libraries like HoloViews and Bokeh. With Panel, you can quickly build feature-rich data exploration and visualization applications.
Creating Interactive Dashboards
One of my favorite features of the Holoviz ecosystem is its interactivity. Using HoloViews and Panel, you can create various interactive dashboards, making data visualization no longer a static process.
For example, you can add various sliders and dropdown boxes to let users freely explore different facets of the data; you can also support interactions like zooming and panning to enhance the data analysis experience. For data science practitioners, this is undoubtedly a powerful tool.
Data Exploration and Visualization
In addition to the final visualization output, the Holoviz ecosystem also emphasizes the process of data exploration. HoloViews provides rich data processing tools to help you quickly understand the structure and distribution characteristics of the data.
You can easily generate various statistical charts, such as histograms, box plots, scatter plots, etc., to gain insights into the essence of the data from multiple angles. This exploratory workflow is very helpful for discovering potential patterns and anomalies in the data.
Cross-platform Compatibility
Finally, the Holoviz ecosystem also focuses on cross-platform compatibility. Whether you're using it in a local environment or cloud platform (like Google Colab), you can get a consistent experience.
Performance Optimization in Google Colab
However, I have indeed encountered performance issues when using Holoviz in Google Colab. Once, when I was rendering a large interactive visualization application in Colab, the interface response was very sluggish.
By consulting documentation and forums, I learned that this was mainly due to Colab's insufficient support for WebGL. To address this issue, I took the following optimization measures:
- Reduce the amount of data, only loading necessary parts
- Use more efficient backend renderers, such as Node.js instead of Python server
- Adjust some rendering parameters, such as disabling shadows and anti-aliasing
Through these adjustments, I finally solved the performance problem and achieved a smooth interactive experience in Colab.
Local Environment vs. Cloud Environment
Of course, if you're using a local environment (like VSCode), you don't need to do much optimization at all. But regardless of the environment, the Holoviz ecosystem is worth trying. It integrates many excellent visualization libraries, provides a unified workflow, allowing you to efficiently and elegantly complete the entire process from data exploration to visualization construction.
Best Practices for Data Visualization
Through the above introduction, I believe you now have a comprehensive understanding of the field of Python data visualization. Finally, I'd like to share some experiences and suggestions from practice to help you better apply the techniques you've learned.
Data Processing and Analysis
The quality of visualization depends on the quality of the data, so data processing is of paramount importance. You need to first use tools like Pandas to clean, transform, and normalize the raw data to ensure its integrity and consistency.
NumPy is also a powerful assistant in the processing. It provides efficient numerical computation functions that can help you quickly complete common tasks such as missing value filling and outlier handling.
Asynchronous Processing and Performance Improvement
For large-scale datasets, if you perform visualization directly in the main thread, it may cause interface freezing and slow response. In this case, you can consider using task queues like Celery to asynchronize data processing and visualization tasks.
By executing time-consuming calculations and rendering in the background, you can significantly improve the response speed of the application, bringing a better user experience. In addition, you can combine other optimization techniques, such as data subsampling, delayed rendering, etc., to further improve the performance of large visualization applications.
Summary
Data visualization is a vast field, and today we've only scratched the surface. There are many excellent visualization libraries in the Python ecosystem, such as Matplotlib, Bokeh, Holoviz, etc., each with its own characteristics and application scenarios.
I encourage you to practice hands-on and explore the powerful features of different libraries. At the same time, pay attention to mastering some general visualization techniques, such as data preprocessing, chart type selection, etc. I believe that with sincere learning, you can definitely become an expert in data visualization.
So, let's continue to work hard and use beautiful visualization works to tell the stories hidden in the data! If you have any questions, feel free to ask me anytime.
Next
Overview of Python Data Visualization
Explore the field of Python data visualization, introducing the characteristics and applications of mainstream libraries such as Matplotlib, Bokeh, and Holoviz.
Advanced Python Data Visualization: How to Create Professional Visualizations with Matplotlib
An in-depth exploration of data visualization and Python programming, covering fundamental concepts, chart types, Python visualization ecosystem, and its practical applications in business analysis and scientific research
The Art and Practice of Python Data Visualization
Discuss the importance and practical methods of Python data visualization, introduce common libraries such as Matplotlib and Plotnine, and use StackOverflow data as an example to explain in detail the steps of data acquisition, preprocessing, basic statistics, correlation analysis, and time series analysis, demonstrating the powerful role of data visualization in revealing data patterns and insights
Next
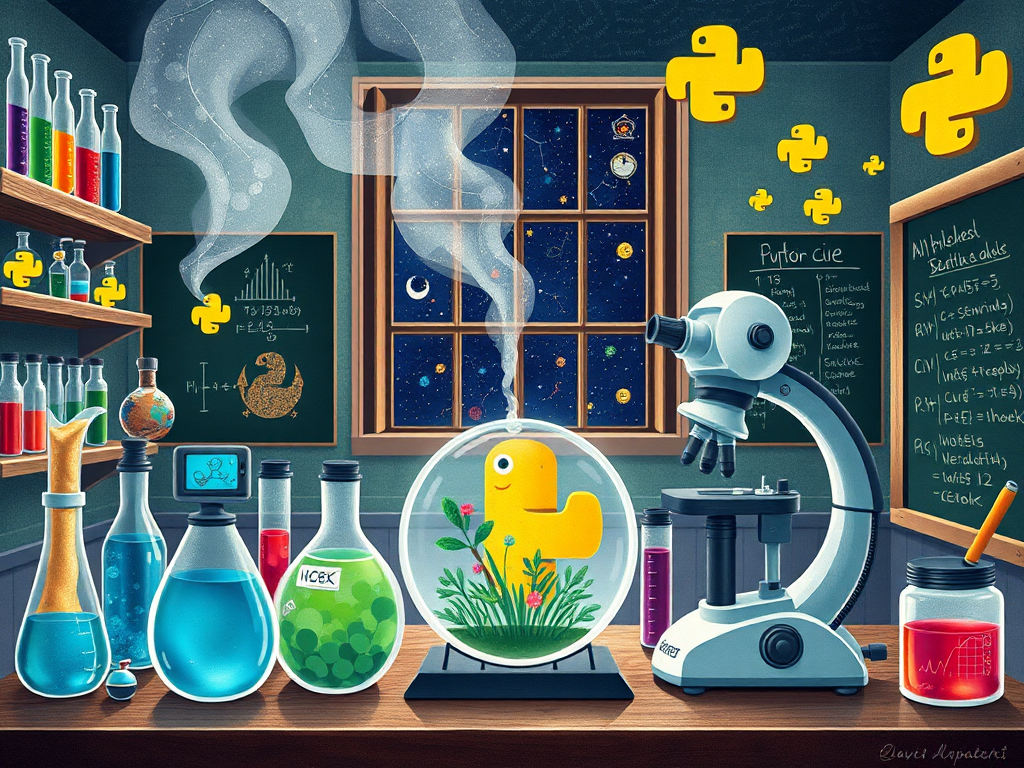
Overview of Python Data Visualization
Explore the field of Python data visualization, introducing the characteristics and applications of mainstream libraries such as Matplotlib, Bokeh, and Holoviz.
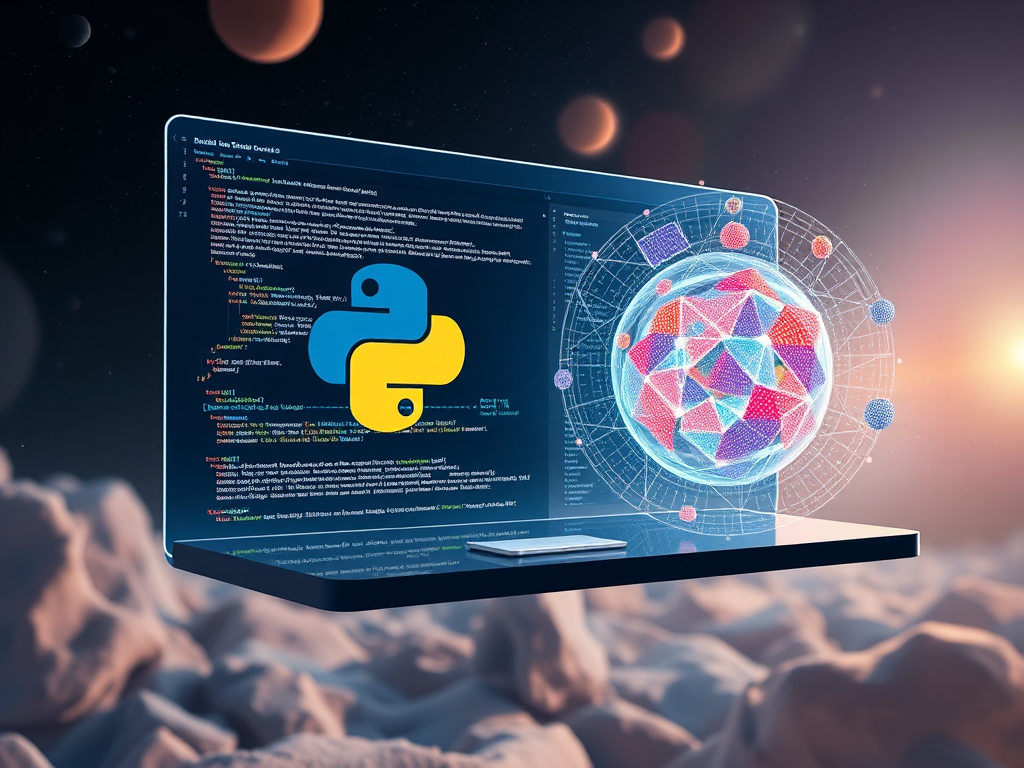
Advanced Python Data Visualization: How to Create Professional Visualizations with Matplotlib
An in-depth exploration of data visualization and Python programming, covering fundamental concepts, chart types, Python visualization ecosystem, and its practical applications in business analysis and scientific research
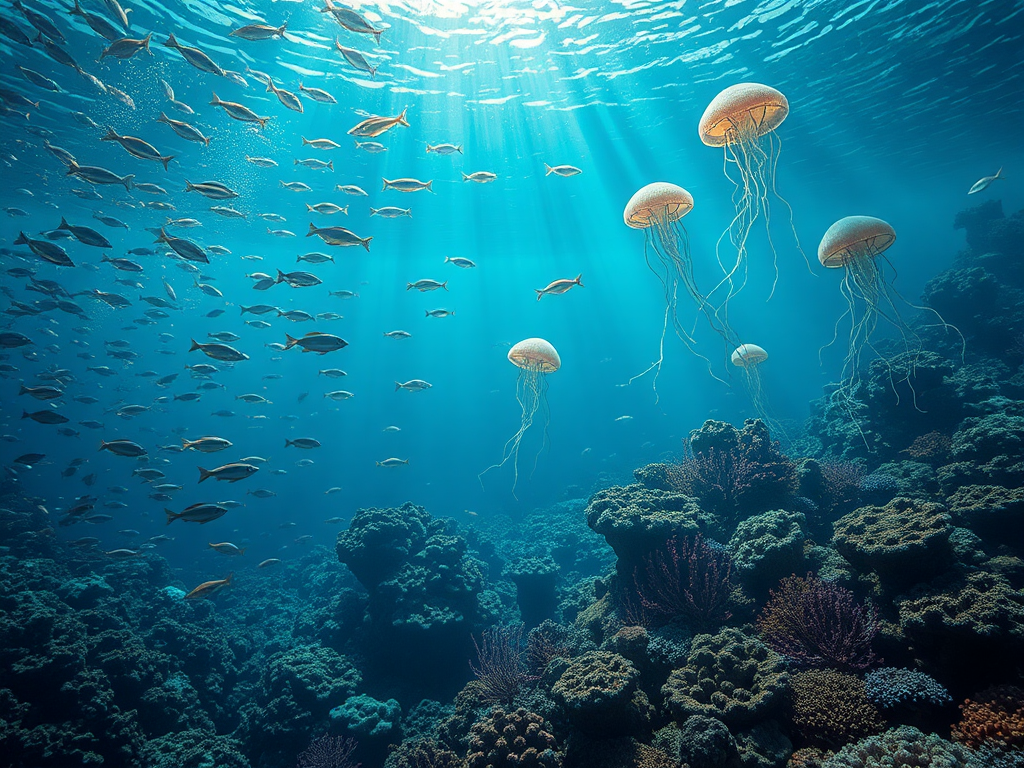
The Art and Practice of Python Data Visualization
Discuss the importance and practical methods of Python data visualization, introduce common libraries such as Matplotlib and Plotnine, and use StackOverflow data as an example to explain in detail the steps of data acquisition, preprocessing, basic statistics, correlation analysis, and time series analysis, demonstrating the powerful role of data visualization in revealing data patterns and insights