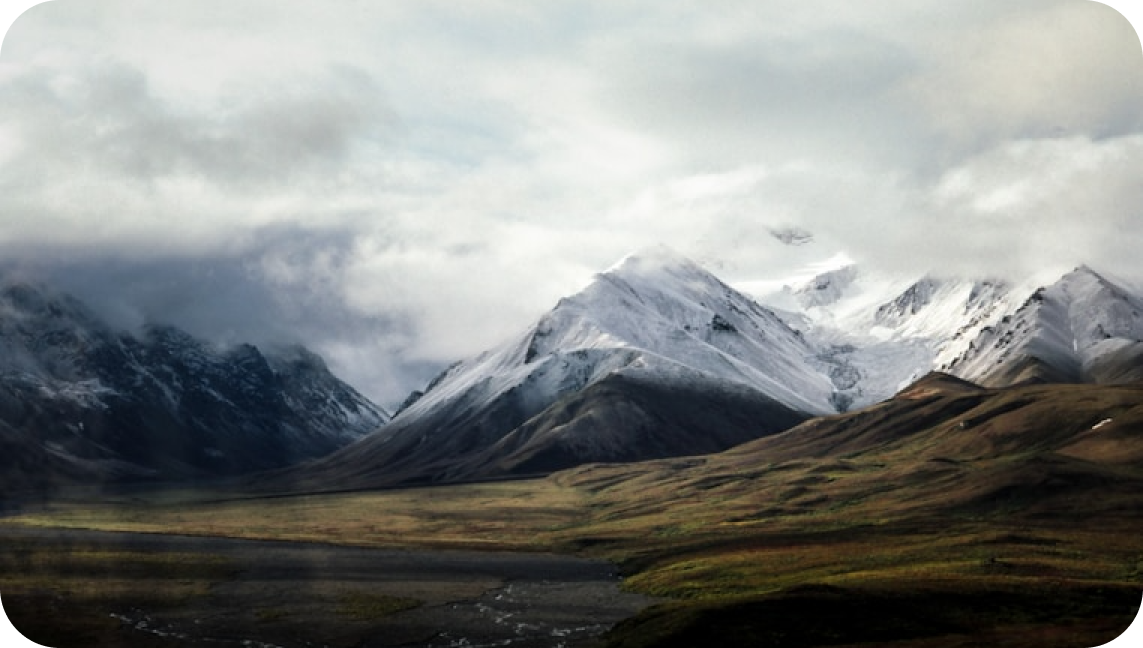
Today I'd like to share a skill I frequently use in Python data analysis - data visualization. Have you ever found yourself with a pile of data but unsure how to make it "speak"? Or perhaps your charts aren't professional enough to effectively convey your ideas? Let's explore the mysteries of Python data visualization together.
First Encounter
I remember my feelings when I first encountered data visualization. At that time, I had a sales dataset that needed to be analyzed for management. Looking at the dense numbers in Excel spreadsheets, I didn't know where to begin. Later, after discovering Python visualization, the data seemed to come alive, with trends and patterns becoming clearly visible.
What exactly is data visualization? In my understanding, it's the transformation of abstract numbers into intuitive graphics. Just like when we learned math as children, teachers always used diagrams to help us understand concepts. Data visualization works the same way, using visual methods to help us better understand and communicate information.
Basics
When it comes to Python data visualization, we must mention Matplotlib, the fundamental library. It's like the "building blocks" of visualization - though basic, it can construct all kinds of beautiful charts.
Let me share a simple but practical example:
import matplotlib.pyplot as plt
import numpy as np
months = ['Jan', 'Feb', 'Mar', 'Apr', 'May', 'Jun']
sales_2022 = [1000, 1200, 900, 1500, 1800, 1300]
sales_2023 = [1200, 1400, 1100, 1700, 2000, 1600]
plt.figure(figsize=(10, 6))
x = np.arange(len(months))
width = 0.35
plt.bar(x - width/2, sales_2022, width, label='2022')
plt.bar(x + width/2, sales_2023, width, label='2023')
plt.xlabel('Month')
plt.ylabel('Sales (10,000 Yuan)')
plt.title('Sales Comparison for First Half of 2022-2023')
plt.xticks(x, months)
plt.legend()
plt.grid(True, linestyle='--', alpha=0.7)
plt.show()
See, just a few lines of code can generate a professional sales comparison chart. Here I used a double bar chart to compare two years of sales data, added grid lines for readability, and set appropriate chart dimensions. These are all tips I've gathered from practice.
Advanced
As I delved deeper into visualization, I discovered many advanced features in Matplotlib. For example, custom styles, multiple subplot layouts, dynamic charts, etc. Let me share a slightly more complex example:
import matplotlib.pyplot as plt
import numpy as np
np.random.seed(42)
data = np.random.normal(100, 15, 1000)
sales_trend = np.linspace(80, 120, 100) + np.random.normal(0, 5, 100)
fig, (ax1, ax2) = plt.subplots(1, 2, figsize=(15, 6))
ax1.hist(data, bins=30, color='skyblue', alpha=0.7)
ax1.set_title('Sales Distribution')
ax1.set_xlabel('Sales (10,000 Yuan)')
ax1.set_ylabel('Frequency')
ax1.grid(True, linestyle='--', alpha=0.5)
ax2.plot(sales_trend, color='red', linewidth=2)
ax2.set_title('Sales Trend')
ax2.set_xlabel('Time (Days)')
ax2.set_ylabel('Sales (10,000 Yuan)')
ax2.grid(True, linestyle='--', alpha=0.5)
plt.tight_layout()
plt.show()
This example shows how to display multi-dimensional data analysis results in one chart. The left side uses a histogram to show sales distribution, while the right side uses a line chart to show sales trends. Such composite charts are particularly useful in real work, allowing audiences to quickly understand multi-dimensional information.
Practical Application
In real work, data visualization is far more than just making pretty charts. Here are several experiences I'd like to share:
- Data cleaning is important I remember once when I used raw data for visualization, the chart came out distorted. Later I discovered it was due to anomalies and missing values in the data. So now I always do data cleaning first:
import pandas as pd
import matplotlib.pyplot as plt
import seaborn as sns
def clean_and_visualize(data):
# Handle missing values
data = data.dropna()
# Handle outliers
Q1 = data['value'].quantile(0.25)
Q3 = data['value'].quantile(0.75)
IQR = Q3 - Q1
lower_bound = Q1 - 1.5 * IQR
upper_bound = Q3 + 1.5 * IQR
data_cleaned = data[(data['value'] >= lower_bound) &
(data['value'] <= upper_bound)]
# Visualize
plt.figure(figsize=(10, 6))
sns.boxplot(data=data_cleaned, x='category', y='value')
plt.title('Data Distribution by Category')
plt.show()
return data_cleaned
- Color schemes are crucial Good color schemes can make charts more professional and better at conveying information. I often use color schemes like this:
import matplotlib.pyplot as plt
import numpy as np
def plot_with_custom_colors():
# Define professional color scheme
colors = ['#2878B5', '#9AC9DB', '#C82423', '#F8AC8C', '#1B8A6B']
# Sample data
categories = ['A', 'B', 'C', 'D', 'E']
values = np.random.randint(50, 100, 5)
plt.figure(figsize=(10, 6))
plt.bar(categories, values, color=colors)
plt.title('Chart with Professional Color Scheme')
plt.show()
- Interactivity is important When presenting data, I've found that adding interactive elements greatly enhances user experience:
import plotly.express as px
import pandas as pd
import numpy as np
def create_interactive_plot():
# Create sample data
dates = pd.date_range(start='2023-01-01', end='2023-12-31', freq='D')
values = np.random.normal(100, 15, len(dates))
trend = np.linspace(80, 120, len(dates))
df = pd.DataFrame({
'date': dates,
'value': values,
'trend': trend
})
# Create interactive chart
fig = px.line(df, x='date', y=['value', 'trend'],
title='Interactive Sales Trend Chart')
fig.show()
Insights
Through years of practice, I've gained a deeper understanding of data visualization. It's not just a technology, but an art. Good data visualization should be like storytelling, able to attract audiences, convey information, and provoke thought.
Did you know? Research shows that the human brain processes visual information 60,000 times faster than text. That's why a good chart is worth a thousand words. In my work, whenever I need to present analysis results to colleagues with non-technical backgrounds, data visualization always helps me achieve twice the result with half the effort.
Finally, I want to say that data visualization is a field that requires continuous learning and practice. Technology advances, aesthetics improve, and user needs change. As data analysts, we need to constantly update our knowledge base and improve our skills. What do you think? Feel free to share your experiences and thoughts in the comments.
Next time, I plan to share how to perform advanced geographic data visualization with Python. Stay tuned.
Next
Mastering Data Visualization in Python: A Complete Guide to Matplotlib
A comprehensive guide exploring data visualization fundamentals in Python, covering core concepts, visualization types, and practical implementations using popular libraries like Matplotlib, Seaborn, and Plotly, with detailed examples and use cases
Overview of Python Data Visualization
Explore the field of Python data visualization, introducing the characteristics and applications of mainstream libraries such as Matplotlib, Bokeh, and Holoviz.
Unleashing the Infinite Possibilities of Data Visualization with Python
This article introduces the application of Python in the field of data visualization, discusses the basic usage of the Matplotlib library and common chart types, as well as how to combine Pandas for data processing and visualization. It also provides tips for chart beautification, helping readers create professional-level data visualization works.
Next
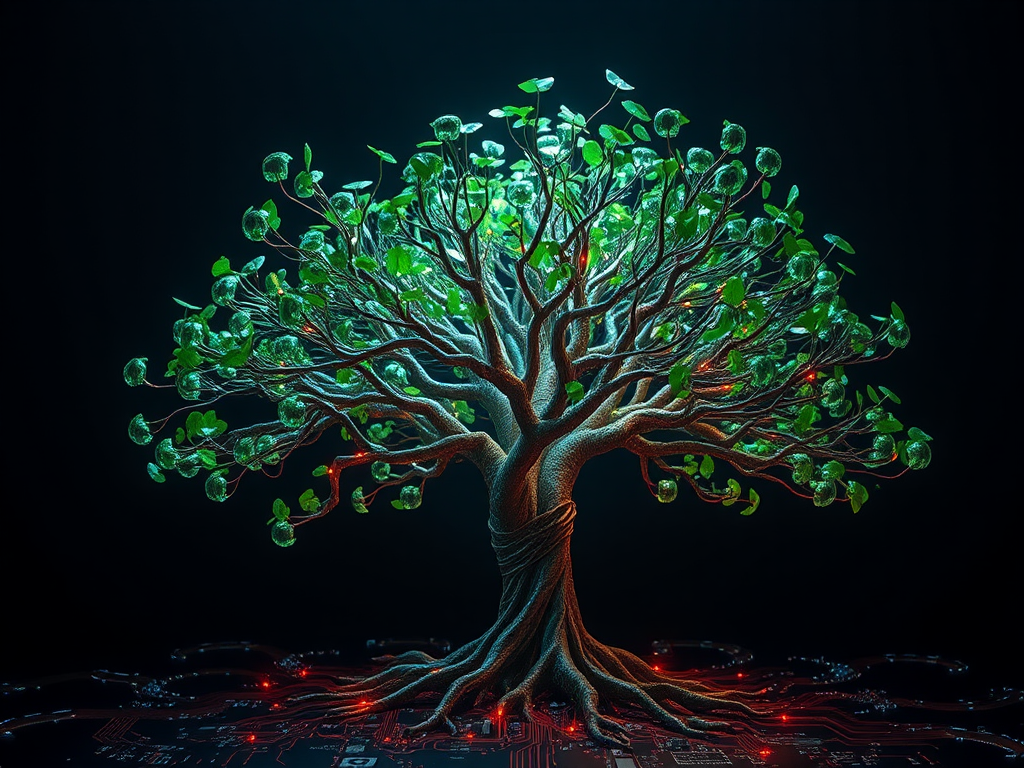
Mastering Data Visualization in Python: A Complete Guide to Matplotlib
A comprehensive guide exploring data visualization fundamentals in Python, covering core concepts, visualization types, and practical implementations using popular libraries like Matplotlib, Seaborn, and Plotly, with detailed examples and use cases
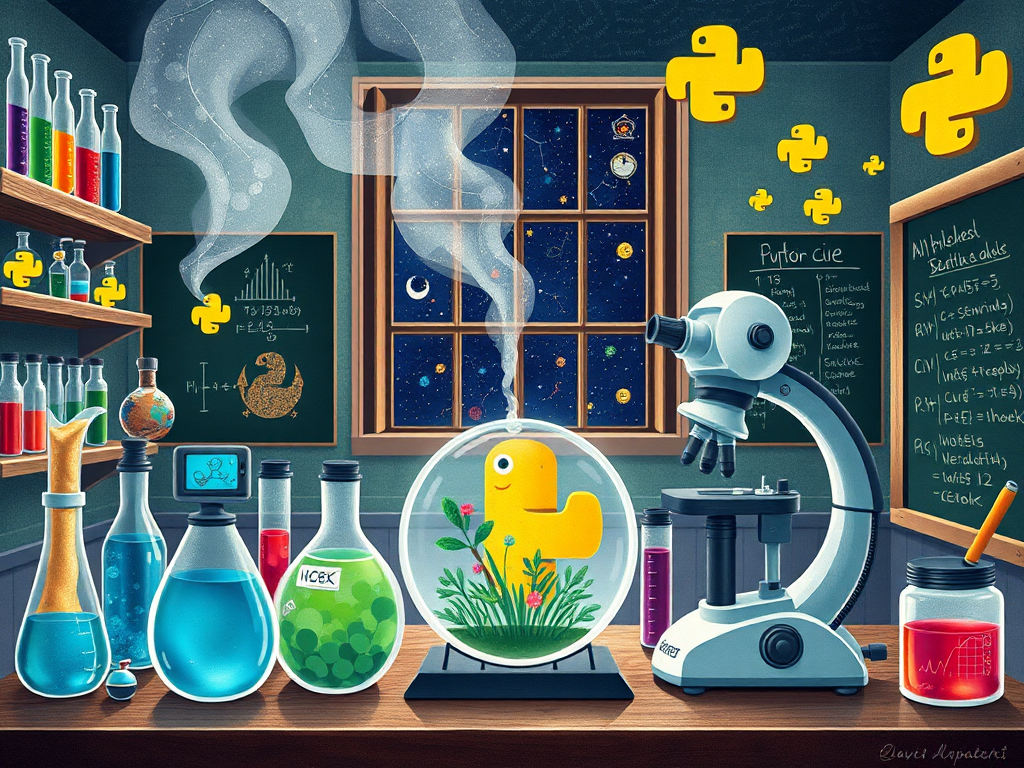
Overview of Python Data Visualization
Explore the field of Python data visualization, introducing the characteristics and applications of mainstream libraries such as Matplotlib, Bokeh, and Holoviz.
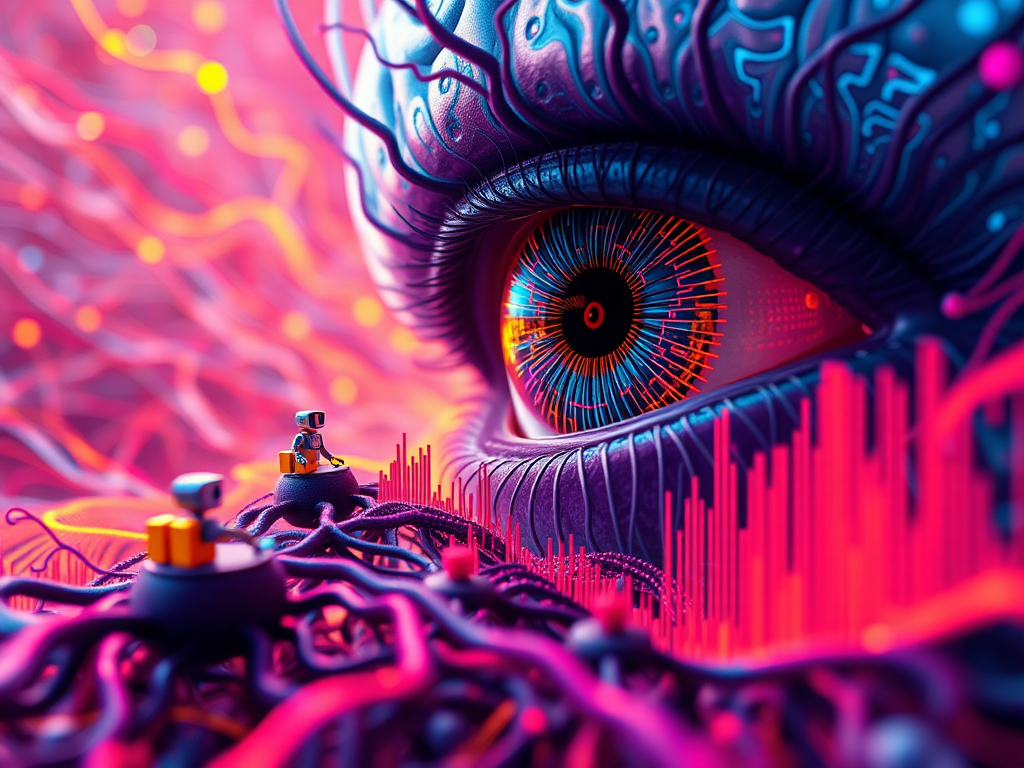
Unleashing the Infinite Possibilities of Data Visualization with Python
This article introduces the application of Python in the field of data visualization, discusses the basic usage of the Matplotlib library and common chart types, as well as how to combine Pandas for data processing and visualization. It also provides tips for chart beautification, helping readers create professional-level data visualization works.