Origin
Have you ever felt overwhelmed by tedious data? Ever wondered how to make these cold numbers come alive? As a Python developer, I deeply understand the importance of data visualization. Today, let me take you on a deep dive into Matplotlib, this powerful visualization tool, and see how it makes data "speak."
Basics
I remember when I first encountered Matplotlib, I was like a child holding a paintbrush for the first time, full of curiosity and anticipation. Matplotlib is indeed the "universal paintbrush" of Python data visualization. It's not just a standalone plotting library but also the foundation for other advanced visualization libraries like Seaborn.
Let's start with a simple example:
import matplotlib.pyplot as plt
import numpy as np
x = np.linspace(0, 10, 100)
y = np.sin(x)
plt.figure(figsize=(10, 6))
plt.plot(x, y, 'b-', label='sin(x)')
plt.title('Sine Wave')
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.legend()
plt.grid(True)
plt.show()
Advanced Level
After mastering basic plotting, I discovered that Matplotlib's charm extends far beyond. It's like a treasure chest, revealing new surprises with each deeper exploration.
For instance, when we need to display multiple subplots in the same figure, subplot becomes very useful:
import matplotlib.pyplot as plt
import numpy as np
fig, axes = plt.subplots(2, 2, figsize=(12, 10))
x1 = np.linspace(0, 5, 100)
axes[0, 0].plot(x1, x1**2)
axes[0, 0].set_title('Quadratic Function')
x2 = np.random.rand(50)
y2 = np.random.rand(50)
axes[0, 1].scatter(x2, y2)
axes[0, 1].set_title('Random Scatter Plot')
x3 = ['A', 'B', 'C', 'D']
y3 = [3, 7, 2, 5]
axes[1, 0].bar(x3, y3)
axes[1, 0].set_title('Bar Chart')
sizes = [30, 20, 25, 15, 10]
labels = ['A', 'B', 'C', 'D', 'E']
axes[1, 1].pie(sizes, labels=labels, autopct='%1.1f%%')
axes[1, 1].set_title('Pie Chart')
plt.tight_layout()
plt.show()
Practical Applications
In real work, data visualization is much more complex than simple examples. I once participated in a financial data analysis project that needed to show the relationship between stock price trends and trading volume. Let me share a real case:
import matplotlib.pyplot as plt
import numpy as np
import pandas as pd
dates = pd.date_range(start='2023-01-01', end='2023-12-31', freq='D')
price = np.random.normal(100, 10, len(dates)).cumsum() + 1000
volume = np.random.normal(1000000, 200000, len(dates))
fig, (ax1, ax2) = plt.subplots(2, 1, figsize=(12, 8), height_ratios=[3, 1])
ax1.plot(dates, price, 'b-', linewidth=1)
ax1.set_title('Stock Price and Volume Analysis')
ax1.set_ylabel('Price')
ax1.grid(True)
ax2.bar(dates, volume, color='g', alpha=0.3)
ax2.set_ylabel('Volume')
ax2.grid(True)
plt.tight_layout()
plt.show()
Insights
Over the years of using Matplotlib, I've summarized several important experiences:
-
Data cleaning is crucial. Even the most powerful visualization tools cannot compensate for problems caused by dirty data. I suggest spending time checking and processing data quality before plotting.
-
Color schemes need attention. In a good visualization work, color schemes often play a crucial role. I often use Matplotlib's colormap to get professional color schemes.
-
Charts should be concise. Sometimes we want to show all information in one chart, but this often backfires. Maintaining chart simplicity for quick reader understanding is key.
-
Focus on interactivity. Modern data visualization requires not only beautiful charts but also user interaction. Matplotlib provides rich interactive features, such as tooltips and zoom functionality.
Looking Forward
The future of data visualization is full of infinite possibilities. With growing data volumes and analysis needs, I believe Matplotlib will continue to evolve and provide us with more powerful features.
Did you know? Matplotlib's name comes from MATLAB, an important tool in scientific computing. But now, Python's visualization ecosystem has far surpassed this starting point. From simple line charts to complex 3D visualizations, from static charts to interactive dashboards, Python's visualization toolkit continues to evolve.
In this data-driven era, mastering data visualization skills is becoming increasingly important. If you're learning Python data visualization, I suggest starting with Matplotlib, building a solid foundation, then gradually exploring other tools like Seaborn, Plotly, etc.
Remember, data visualization is not just technology, but also an art. It requires us to find balance between technology and aesthetics, seeking resonance between accuracy and beauty.
What do you think is the most challenging part of data visualization? Feel free to share your thoughts and experiences in the comments.
Conclusion
Writing this, I recall what one of my mentors said: "Data visualization is like storytelling; good charts let the data speak for itself." Indeed, when we can skillfully use tools like Matplotlib, we can present data more vividly to people.
I hope this article helps you better understand and use Matplotlib. The road of data visualization is long; let's continue to explore and improve together on this journey. Do you have any unique data visualization experiences to share?
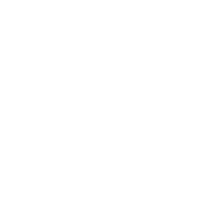
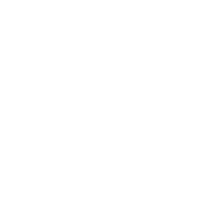