Hey, Python enthusiasts! Today let's talk about data visualization in Python. Are you often overwhelmed by large amounts of numbers and tables? Don't worry, data visualization was born to solve this problem! It can make boring data lively and interesting, helping you spot hidden patterns in your data at a glance. Let's explore the world of data visualization in Python together!
What is Visualization
First, we need to understand what data visualization is. Simply put, it's the process of turning numbers into charts. Imagine you have a long string of sales data - looking at the numbers alone might not tell you much. But if you turn it into a beautiful line chart, you can immediately see whether the sales trend is going up or down. That's the magic of data visualization!
Why is data visualization so important in Python programming? Because it helps us: 1. Quickly understand data trends 2. Discover anomalies in data 3. Visually present analysis results 4. Make complex data simple and easy to understand
Imagine you're a data scientist who just finished analyzing a large amount of user behavior data. You need to report the results to your boss - are you going to make your boss stare at Excel spreadsheets for hours? Of course not! You just need a few beautiful charts to help your boss understand your analysis results at a glance. That's the power of data visualization!
Toolbox
When it comes to data visualization in Python, we must mention several powerful libraries. They're like your magical toolbox, each with its unique powers.
Matplotlib: The Swiss Army Knife of Plotting
Matplotlib is arguably the big brother in Python's plotting world. It's powerful and can create almost any type of chart you can think of. From simple line charts to complex 3D graphics, Matplotlib can handle it all.
The first time I used Matplotlib, I was amazed by its flexibility. You can precisely control every detail of your charts, from axis ticks to legend placement. However, this flexibility also means the learning curve might be a bit steep. But don't worry, once you master the basics, you can create stunning visualizations.
Seaborn: The Beauty Expert of Statistical Charts
If Matplotlib is the Swiss Army knife of plotting, then Seaborn is an artist who masters aesthetics. It's built on top of Matplotlib but provides a higher-level interface and more beautiful default styles.
I particularly love using Seaborn for statistical charts. For example, when I need to quickly check data distribution, Seaborn's distplot function is a lifesaver. With just a few lines of code, you get a beautiful histogram with kernel density estimation. Plus, Seaborn's color schemes are carefully designed to make your charts look both professional and pleasing to the eye.
Pandas: The Perfect Partner for Data Processing and Visualization
When it comes to data processing, Pandas is definitely a superstar in the Python world. But did you know? Pandas also has some simple but useful built-in plotting functions.
I often use Pandas' plotting functions during the data exploration phase. For example, when I want to quickly check the distribution of a column, I just need one line of code: df['column_name'].hist()
to get a histogram. This convenience is very useful when initially understanding your data.
Plotly: The Magician of Interactive Visualization
If you want your charts to be not just beautiful but also interactive, then Plotly is your best choice. Plotly can create various beautiful interactive charts, perfect for web applications or Jupyter Notebooks.
I was amazed the first time I used Plotly to create an interactive scatter plot. You can zoom in and out, hover to see detailed information about data points, and even selectively show or hide certain data series. This interactivity makes data exploration more fun and intuitive.
Hands-on Practice
Alright, we've covered enough theory. Now, let's get hands-on and see how these tools work in practice.
Matplotlib: Creating Sales Trend Charts
Let's say you're a company's data analyst, and your boss asks you to analyze the past week's sales data. You have the following data:
import matplotlib.pyplot as plt
days = ['Monday', 'Tuesday', 'Wednesday', 'Thursday', 'Friday', 'Saturday', 'Sunday']
sales = [1000, 1200, 1100, 1500, 2000, 2500, 2200]
plt.figure(figsize=(10, 6))
plt.plot(days, sales, marker='o')
plt.title('Last Week\'s Sales Trend')
plt.xlabel('Date')
plt.ylabel('Sales (Yuan)')
plt.grid(True)
plt.show()
This code will generate a beautiful line chart clearly showing the sales trend over a week. You'll notice that weekend sales are significantly higher than weekdays. This finding might lead your boss to adjust marketing strategies, like increasing promotions during weekends.
I think one clever feature of Matplotlib is the marker='o'
parameter. It adds a dot at each data point, making the trend clearer. You can try changing this parameter to see different effects. For example, using marker='*'
will show a star at each data point.
Seaborn: Analyzing Customer Age Distribution
Now, let's say you want to understand your company's customer age distribution. Seaborn can help you easily create a beautiful histogram:
import seaborn as sns
import matplotlib.pyplot as plt
import numpy as np
np.random.seed(0)
ages = np.random.normal(35, 10, 1000).astype(int)
plt.figure(figsize=(10, 6))
sns.histplot(ages, bins=30, kde=True)
plt.title('Customer Age Distribution')
plt.xlabel('Age')
plt.ylabel('Frequency')
plt.show()
This code will generate a histogram of age distribution with a smooth density curve. From the chart, you might find that customer ages are mainly concentrated between 25-45 years old. This information is very valuable for developing marketing strategies.
I particularly like Seaborn's kde=True
parameter. It adds a kernel density estimation curve, making it easier to see the overall distribution trend. You can try removing this parameter to see how the effect differs.
Plotly: Creating Interactive Scatter Plots
Finally, let's use Plotly to create an interactive scatter plot to analyze the relationship between product prices and sales:
import plotly.express as px
import pandas as pd
import numpy as np
np.random.seed(0)
df = pd.DataFrame({
'Product': ['A', 'B', 'C', 'D'] * 25,
'Price': np.random.uniform(10, 100, 100),
'Sales': np.random.uniform(50, 500, 100)
})
fig = px.scatter(df, x='Price', y='Sales', color='Product',
title='Product Price vs Sales Relationship')
fig.show()
This code will generate an interactive scatter plot where you can zoom in and out, hover to see detailed information for each point. You might discover that some products show a clear negative correlation between price and sales.
I think the best thing about Plotly is its interactivity. You can try adding the size='Sales'
parameter, which will make the point size vary with sales, adding a new dimension to your chart.
Learning Path
Learning data visualization is a gradual process. I suggest you start with these aspects:
-
Master the basics: Start by diving deep into Matplotlib. Although its learning curve might be steep, it's the foundation for many other libraries.
-
Explore advanced libraries: Then you can learn Seaborn and Plotly, which help you create more complex and beautiful charts.
-
Practice, practice, and more practice: Find some real datasets and try visualizing them in different ways. You'll discover that the same data visualized differently might lead to completely different insights.
-
Pay attention to details: A good data visualization not only shows data but also pays attention to details like titles, labels, and colors. These details often make your charts more professional and persuasive.
-
Learn design principles: Understand some basic design principles, like how to choose appropriate chart types and how to use colors. This knowledge will make your visualizations more outstanding.
Remember, data visualization is not just a science but also an art. It requires both data processing and programming skills, as well as aesthetics and creativity. So, maintain your curiosity and creativity during the learning process.
Have you noticed that when you visualize the same data in different ways, you might get completely different insights? That's the charm of data visualization. It not only helps us understand data but also inspires us to think.
So, are you ready to start your Python data visualization journey? Remember to experiment and practice a lot. Trust me, when you create your first stunning data visualization, that sense of achievement is incomparable.
Finally, I want to say that data visualization is not just a skill, it's a way of thinking. It teaches us how to look at data from different angles and how to simplify complex information into intuitive images. In this data-driven era, mastering data visualization skills is like having a pair of eyes that can see into the future.
Well, that's all for today's sharing. What are your thoughts or experiences with Python data visualization? Feel free to share your ideas in the comments. Let's explore together in the ocean of data and discover those fascinating stories hidden behind the numbers.
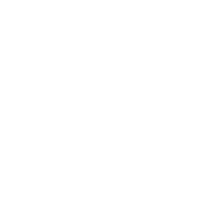
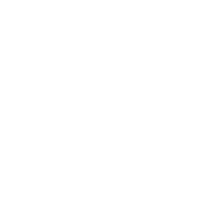