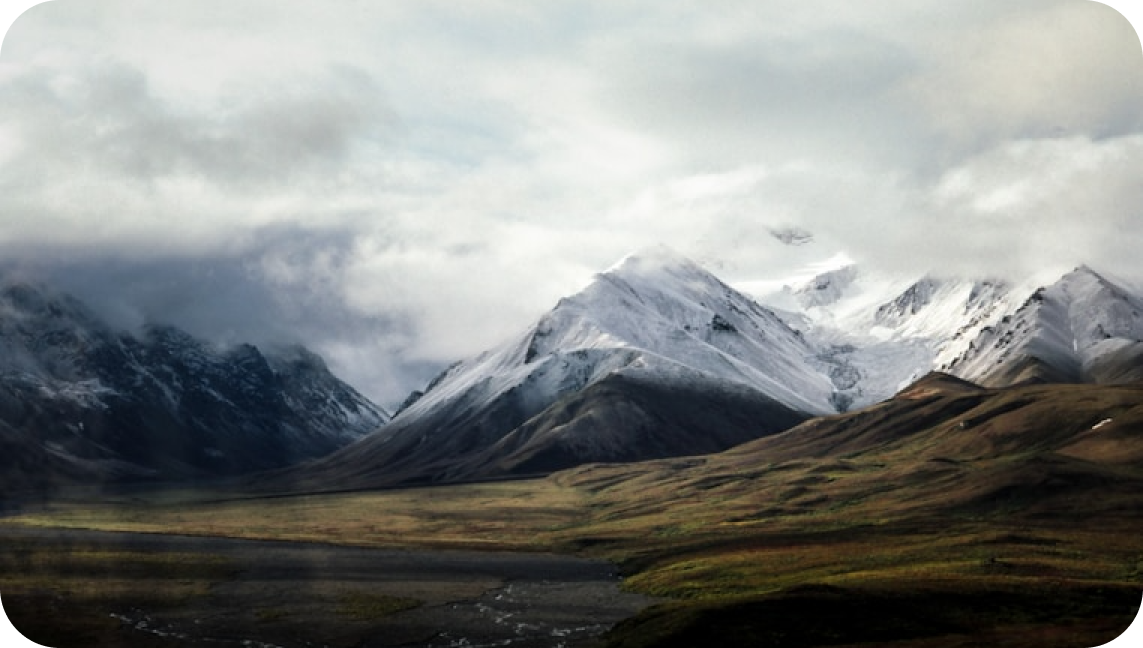
Opening Thoughts
Have you ever been in this situation? You have a pile of data but don't know how to present it in a way your boss can understand at a glance. Or you're working on your thesis, you've done the statistical analysis, but you're unsure which charts would perfectly present your research findings.
As a Python enthusiast, I deeply understand the importance of data visualization. I remember when I first started learning data analysis, I often struggled with making data "speak human." Until I met Matplotlib, this magical tool that makes data "speak."
Basics
Before we start hands-on work, we need to prepare our "weapons." The main tool we'll use is the Matplotlib library, which is like the Swiss Army knife of data visualization - simple yet incredibly powerful.
from numpy import sin
import matplotlib.pyplot as plt
import numpy as np
from random import seed, randint
Did you know? Matplotlib's design was inspired by MATLAB's plotting system. That explains why much of its syntax looks so familiar.
Getting Started
Line Plots
Line plots are the most basic type of chart, particularly suitable for showing how data changes over time. Let's start with a simple sine wave:
x = np.linspace(0, 10, 100)
y = sin(x)
plt.plot(x, y)
plt.title('Elegant Sine Wave')
plt.xlabel('Time')
plt.ylabel('Amplitude')
plt.show()
Would you like me to explain or break down this code?
Scatter Plots
When we want to explore relationships between two variables, scatter plots come in handy:
x = np.random.rand(50)
y = x + np.random.normal(0, 0.1, 50)
plt.scatter(x, y, alpha=0.5)
plt.title('Scatter Plot: Exploring Variable Relationships')
plt.xlabel('X Variable')
plt.ylabel('Y Variable')
plt.show()
Would you like me to explain or break down this code?
Advanced Topics
Histograms
Histograms are one of my favorite statistical charts. They can visually display data distribution characteristics:
data = np.random.normal(0, 1, 1000)
plt.hist(data, bins=30, alpha=0.7, color='skyblue')
plt.title('Data Distribution at a Glance')
plt.xlabel('Value Range')
plt.ylabel('Frequency')
plt.show()
Would you like me to explain or break down this code?
Box Plots
Speaking of data distribution, we must mention box plots. They help us quickly identify outliers:
data = [np.random.normal(0, std, 100) for std in range(1, 4)]
plt.boxplot(data, patch_artist=True)
plt.title('Box Plot: The Art of Data Distribution')
plt.show()
Would you like me to explain or break down this code?
Practical Tips
In actual work, I've summarized some useful visualization tips:
-
Color schemes matter: I often use
plt.style.use('seaborn')
for a more professional look. -
Charts should tell stories: Pure data display isn't enough; use titles and labels to clearly convey information.
-
Keep it simple: Sometimes, removing unnecessary elements makes important information stand out more.
Let's look at a comprehensive example:
plt.style.use('seaborn')
fig, axes = plt.subplots(2, 2, figsize=(12, 8))
x = np.linspace(0, 10, 100)
axes[0,0].plot(x, sin(x))
axes[0,0].set_title('Line Plot')
x = np.random.rand(50)
y = np.random.rand(50)
axes[0,1].scatter(x, y)
axes[0,1].set_title('Scatter Plot')
data = np.random.normal(0, 1, 1000)
axes[1,0].hist(data, bins=30)
axes[1,0].set_title('Histogram')
data = [np.random.normal(0, std, 100) for std in range(1, 4)]
axes[1,1].boxplot(data)
axes[1,1].set_title('Box Plot')
plt.tight_layout()
plt.show()
Would you like me to explain or break down this code?
Conclusion
Data visualization isn't just technology; it's an art. Through appropriate charts, we can make dry data come alive and become interesting. Have you noticed how data analysis becomes more engaging once you master these visualization techniques?
By the way, do you know what other interesting chart types Matplotlib has? Feel free to share your discoveries in the comments. Let's explore the infinite possibilities of data visualization together.
Next
Mastering Data Visualization in Python: A Complete Guide to Matplotlib
A comprehensive guide exploring data visualization fundamentals in Python, covering core concepts, visualization types, and practical implementations using popular libraries like Matplotlib, Seaborn, and Plotly, with detailed examples and use cases
Overview of Python Data Visualization
Explore the field of Python data visualization, introducing the characteristics and applications of mainstream libraries such as Matplotlib, Bokeh, and Holoviz.
Unleashing the Infinite Possibilities of Data Visualization with Python
This article introduces the application of Python in the field of data visualization, discusses the basic usage of the Matplotlib library and common chart types, as well as how to combine Pandas for data processing and visualization. It also provides tips for chart beautification, helping readers create professional-level data visualization works.
Next
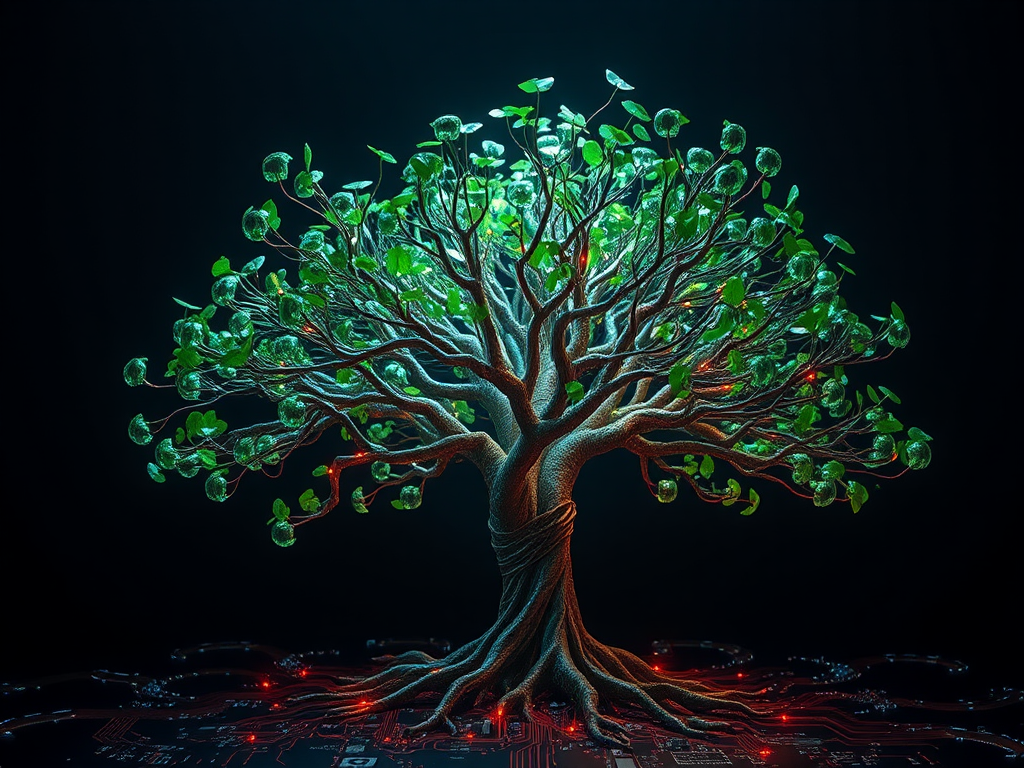
Mastering Data Visualization in Python: A Complete Guide to Matplotlib
A comprehensive guide exploring data visualization fundamentals in Python, covering core concepts, visualization types, and practical implementations using popular libraries like Matplotlib, Seaborn, and Plotly, with detailed examples and use cases
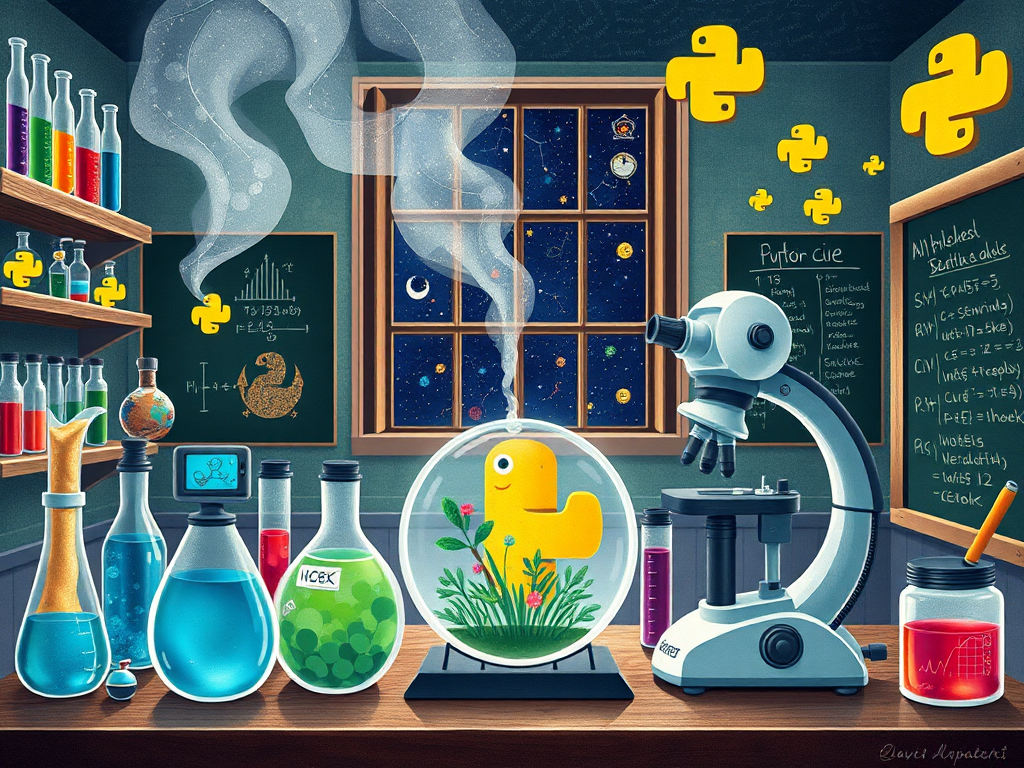
Overview of Python Data Visualization
Explore the field of Python data visualization, introducing the characteristics and applications of mainstream libraries such as Matplotlib, Bokeh, and Holoviz.
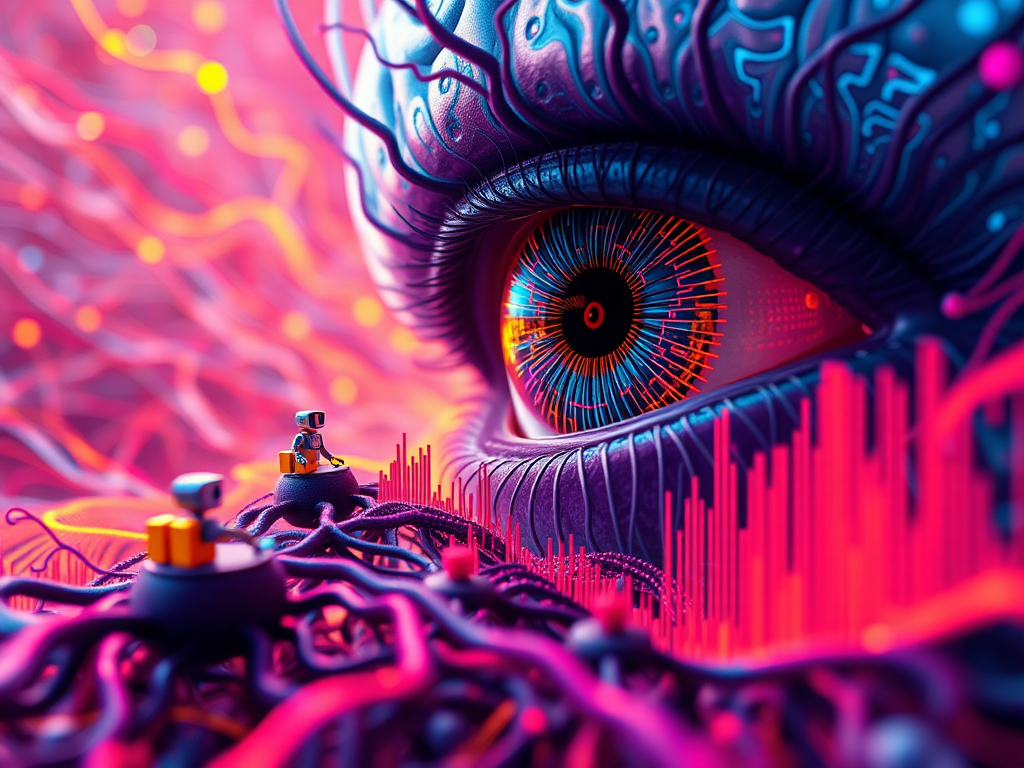
Unleashing the Infinite Possibilities of Data Visualization with Python
This article introduces the application of Python in the field of data visualization, discusses the basic usage of the Matplotlib library and common chart types, as well as how to combine Pandas for data processing and visualization. It also provides tips for chart beautification, helping readers create professional-level data visualization works.