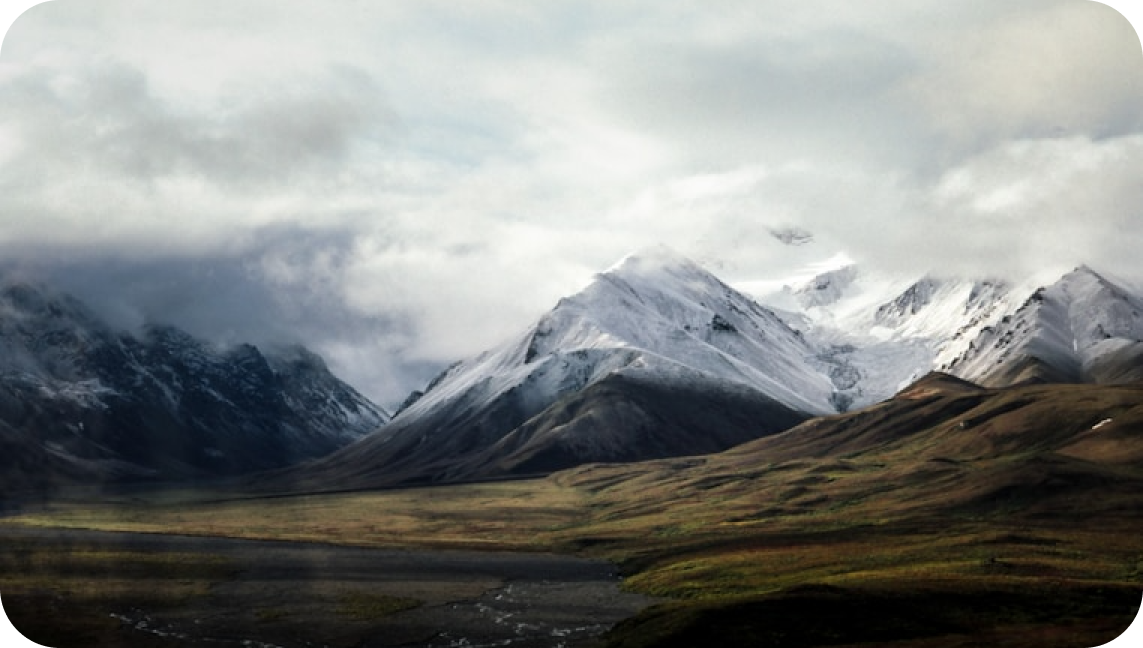
Introduction
Hey, folks! Data visualization is truly an endless source of fun! By transforming dry data into vivid and visual charts, we can not only better understand the information contained in the data but also present analysis results in a clear and easy-to-understand way. With the power of Python, this powerful programming language, the process of data visualization has become unprecedentedly simple and efficient. So, let's explore the mysteries of Python data visualization together today!
Overview of Python Data Visualization
Data visualization plays an increasingly important role in today's big data era. Whether in scientific research, business, or daily life, processing and presenting data is an indispensable basic skill. Python, as a simple to learn yet powerful programming language, occupies a prominent place in the field of data visualization.
In Python, there are many excellent data visualization libraries to choose from, such as:
- Matplotlib: Comprehensive functionality, wide coverage, capable of generating publication-quality charts.
- Seaborn: Built on top of Matplotlib, provides a more advanced interface, commonly used for statistical data visualization.
- Plotly: Can create beautiful dynamic interactive charts, supports online publishing and collaboration.
- Bokeh: Also focuses on web interactive visualization, with excellent rendering performance for large datasets.
In addition, there are many other excellent visualization libraries in the Python ecosystem, such as Folium for geographic data visualization, Mayavi for 3D data visualization, and so on. We can choose the appropriate tools based on specific application scenarios and requirements.
Exploring the Matplotlib Library
Since Matplotlib is comprehensive, widely applied, and serves as the underlying plotting library for other visualization libraries, let's start our exploration with this library.
Basic Usage
Let's look at a simple example where we want to plot a basic linear function y=x:
import matplotlib.pyplot as plt
import numpy as np
x = np.linspace(-10, 10, 100) # Create x values
y = x # Calculate y values
plt.figure() # Create a figure instance
plt.plot(x, y) # Plot (x, y) points
plt.show() # Display the image
Look, with just a few simple lines of code, a clean and crisp linear function graph is drawn! Isn't that cool?
The basic process of using Matplotlib is: first import the relevant modules, create data, then call the plot() function to draw, and finally show() to display the image.
Customization
Of course, if it were just these few features, Matplotlib would be too thin. It provides a large number of customization options, allowing us to optimize the chart step by step until it shines. For example, we can:
- Add titles, axis labels, etc.:
plt.title('Linear Function') # Set title
plt.xlabel('X') # Set x-axis label
plt.ylabel('Y') # Set y-axis label
- Adjust line style and color:
plt.plot(x, y, '--r') # Dashed line, red
- Add legend, grid lines, etc.:
plt.legend(['Linear']) # Add legend
plt.grid(True) # Add grid lines
- Set axis range, tick values, etc.:
plt.xlim(-5, 5) # Set x-axis range
plt.xticks(np.arange(-5, 6)) # Set x-axis tick values
After a series of adjustments, this simple linear function graph becomes colorful and information-rich! Have you come up with some of your own ideas? Come on, give it a try!
Common Chart Types
A single line is not enough, Matplotlib has prepared a variety of common chart types for us, allowing us to choose the most suitable visualization form based on data characteristics.
Scatter Plot
Scatter plots are used to show the relationship between two data dimensions and are particularly suitable for observing data distribution patterns. Let's use a scatter plot to show Annie's data on feeding squirrels in the park:
food_counts = [34, 25, 46, 25, 27, 62, 54, 31] # Daily feeding amount
squirrel_counts = [53, 49, 57, 48, 59, 72, 65, 51] # Daily number of squirrels appearing
plt.figure()
plt.scatter(food_counts, squirrel_counts) # Draw scatter plot
plt.title("Park Squirrel Data")
plt.xlabel("Food Count")
plt.ylabel("Squirrel Count")
plt.show()
From the graph, we can clearly see that the feeding amount and the number of squirrels appearing are roughly positively correlated. Well done, Annie!
Bar Chart
Bar charts are good at showing data for different categories. Let's look at Jimmy's weekly exercise data:
days = ['Mon', 'Tue', 'Wed', 'Thu', 'Fri', 'Sat', 'Sun']
exercise_times = [25, 32, 0, 45, 18, 67, 52]
plt.figure(figsize=(8, 5)) # Set canvas size
plt.bar(days, exercise_times) # Draw bar chart
plt.title("Jimmy's Weekly Exercise")
plt.xlabel('Day')
plt.ylabel('Exercise Time (min)')
plt.show()
At a glance, we can see that Jimmy exercises longer on weekends, but didn't exercise on Wednesday. If we presented this data in a table, it would be hard to see the pattern at a glance.
Pie Chart
Pie charts are useful for showing the proportions of different parts that make up a whole. For example, let's analyze Clark's calorie distribution for three meals a day:
meals = ['Breakfast', 'Lunch', 'Dinner']
calories = [420, 670, 1280]
plt.figure(figsize=(6, 6)) # Set canvas size
plt.pie(calories, labels=meals, autopct='%.1f%%') # Draw pie chart, show percentages
plt.title("Clark's Daily Calorie Intake")
plt.axis('equal') # Set aspect ratio equal to ensure circular shape
plt.show()
It's clear at a glance that Clark's dinner calories account for more than half of the total daily calories. It looks like he needs to watch his intake.
Combining with Pandas
In practical applications, we often need to deal with structured tabular data. The powerful data analysis library Pandas can work well with Matplotlib, greatly simplifying the process of data processing and visualization.
Time Series Data
Let's take the average monthly temperature data for New York City in 2022 as an example to see how to visualize time series data:
import pandas as pd
temperatures = [32.6, 37.2, 46.3, 55.1, 66.2, 73.8, 79.1, 77.4, 68.5, 58.6, 48.2, 36.1]
months = pd.date_range(start='2022-01-01', end='2022-12-01', freq='M')
ts = pd.Series(temperatures, index=months)
plt.figure(figsize=(10, 6))
plt.plot(ts)
plt.xlabel('Month')
plt.ylabel('Temperature (°F)')
plt.title('Average Monthly Temperatures - NYC 2022')
plt.show()
First, we use Pandas to create a time series object ts
. Then by directly calling its plot
method, we can generate a beautiful line chart!
The seamless integration of Pandas and Matplotlib makes the visualization of time series data incredibly simple.
Grouped Data
Let's look at a more complex example - analyzing a company's 2022 revenue data by department:
dept_revenue = pd.DataFrame({
'Department': ['Sales', 'Marketing', 'R&D', 'IT', 'HR'],
'Revenue': [825, 612, 479, 352, 119]
})
plt.figure(figsize=(8, 6))
dept_revenue.set_index('Department').plot(kind='bar')
plt.xlabel('Department')
plt.ylabel('Revenue (Million USD)')
plt.title('Company Revenue by Department - 2022')
plt.show()
Here we created a DataFrame object to store department and revenue data. Then, with just one line of plot
code, we can generate an intuitive bar chart!
By using Pandas to process data and combining it with Matplotlib for plotting, we can quickly analyze and visualize various complex data. The powerful combination of the two greatly improves the efficiency of data analysis.
Beautifying Charts
Generating charts is just the first step. Next, we need to beautify the charts to make them more attractive and readable. Matplotlib also provides us with a lot of practical functions in this regard.
Choosing the Right Chart Type
Different data characteristics are suitable for different types of charts. For example, for categorical data, we can use bar charts or pie charts; for data showing trends over time, line charts or area charts would be more appropriate; while scatter plots are good at showing the relationship between two variables, and so on.
Choosing the right chart type not only presents the data more clearly but also makes the chart look more aesthetically pleasing and professional.
Optimizing Chart Layout
The layout of a chart directly affects its readability. We can adjust the aspect ratio of the chart, the spacing and arrangement of various components to make the chart look more harmonious and beautiful.
plt.figure(figsize=(10, 6)) # Set chart size
plt.subplots_adjust(left=0.1, right=0.9, bottom=0.15, top=0.9) # Adjust chart layout
In addition, we can adjust the axis range, tick values, etc., to ensure that the data can be fully displayed in the chart area.
Using Legends and Annotations
Legends and annotations can provide sufficient context information for the chart, allowing readers to understand the chart content at a glance. We can customize the position, font size, etc. of the legend so that it doesn't affect the aesthetics of the chart.
plt.legend(fontsize=12, loc='upper left') # Set legend font size and position
For some special data points, we can use the annotation feature to make them more prominent.
plt.annotate('Peak Value', xy=(2, 1000), xytext=(3, 800),
arrowprops=dict(arrowstyle='->')) # Add an arrow annotation
Customizing Colors and Styles
Using appropriate colors and styles can make the chart look more vivid and attractive. We can customize the color and style of lines and data points, and even set different color schemes for different data series.
plt.plot(x, y, 'r--', linewidth=2) # Red dashed line, line width 2
plt.scatter(x, y, c='b', marker='*', s=80) # Blue star-shaped data points, size 80
In addition, Matplotlib also provides some built-in style sets that can give our charts a unique visual style.
plt.style.use('dark_background') # Use dark background style
After careful design and adjustment in various aspects, we can generate professional-level charts that are colorful and well-organized!
Conclusion
Through the sharing above, I believe you have gained an initial understanding of Python's powerful capabilities in the field of data visualization. Whether it's basic statistical chart drawing or complex data organization and visual analysis, Python can provide us with efficient, flexible, and beautiful solutions.
Of course, this article has only scratched the surface of data visualization. In practice, we still need to choose appropriate tools and technical routes based on specific data characteristics and visualization requirements. However, with Python as our capable assistant, we believe we can master various data visualization challenges!
So, what kind of brilliant data visualization effects are you most looking forward to achieving with Python? Now, let your imagination run wild and navigate freely in the ocean of data! If you have any questions, feel free to give me feedback at any time.
Next
Mastering Data Visualization in Python: A Complete Guide to Matplotlib
A comprehensive guide exploring data visualization fundamentals in Python, covering core concepts, visualization types, and practical implementations using popular libraries like Matplotlib, Seaborn, and Plotly, with detailed examples and use cases
Overview of Python Data Visualization
Explore the field of Python data visualization, introducing the characteristics and applications of mainstream libraries such as Matplotlib, Bokeh, and Holoviz.
Unleashing the Infinite Possibilities of Data Visualization with Python
This article introduces the application of Python in the field of data visualization, discusses the basic usage of the Matplotlib library and common chart types, as well as how to combine Pandas for data processing and visualization. It also provides tips for chart beautification, helping readers create professional-level data visualization works.
Next
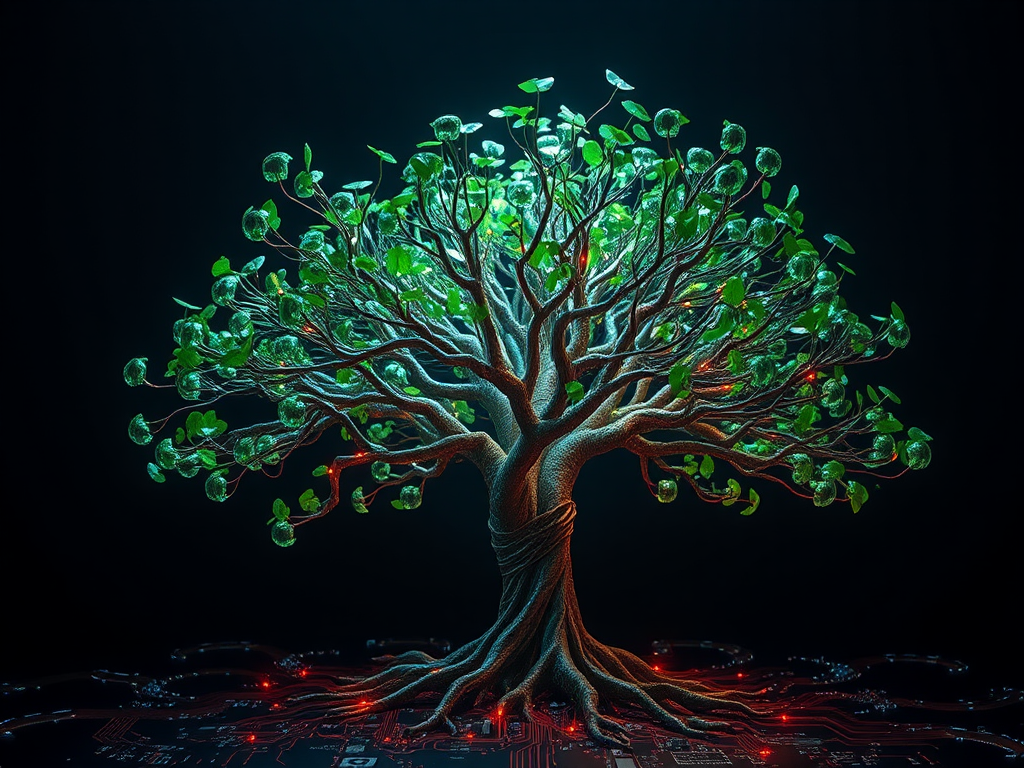
Mastering Data Visualization in Python: A Complete Guide to Matplotlib
A comprehensive guide exploring data visualization fundamentals in Python, covering core concepts, visualization types, and practical implementations using popular libraries like Matplotlib, Seaborn, and Plotly, with detailed examples and use cases
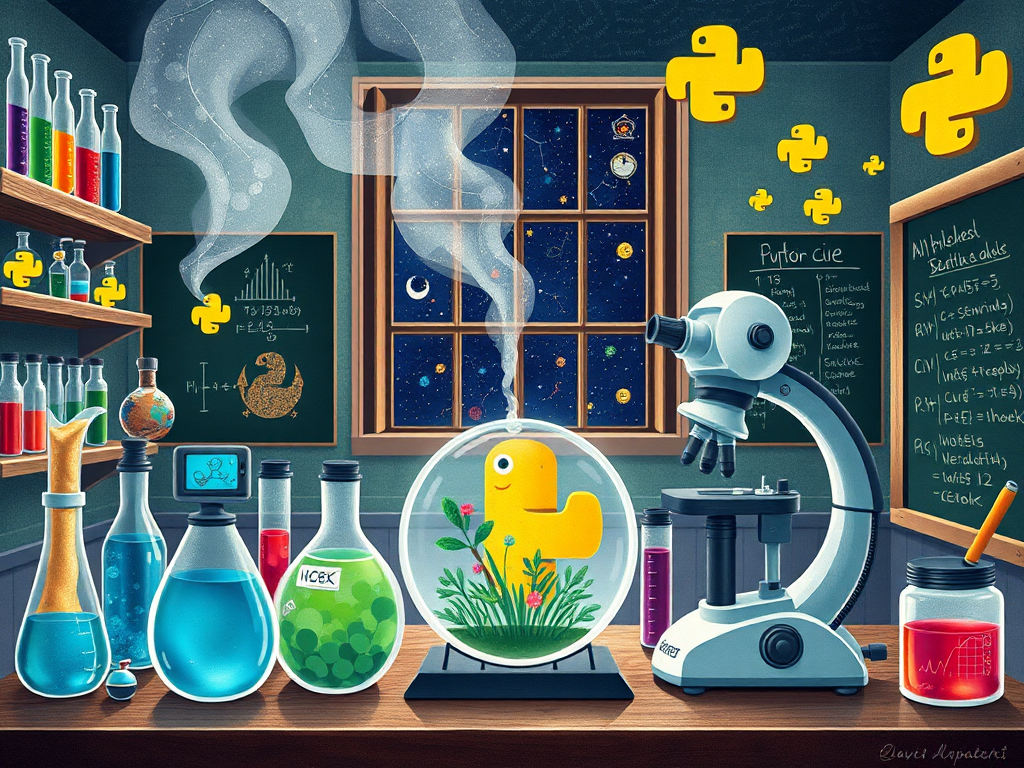
Overview of Python Data Visualization
Explore the field of Python data visualization, introducing the characteristics and applications of mainstream libraries such as Matplotlib, Bokeh, and Holoviz.
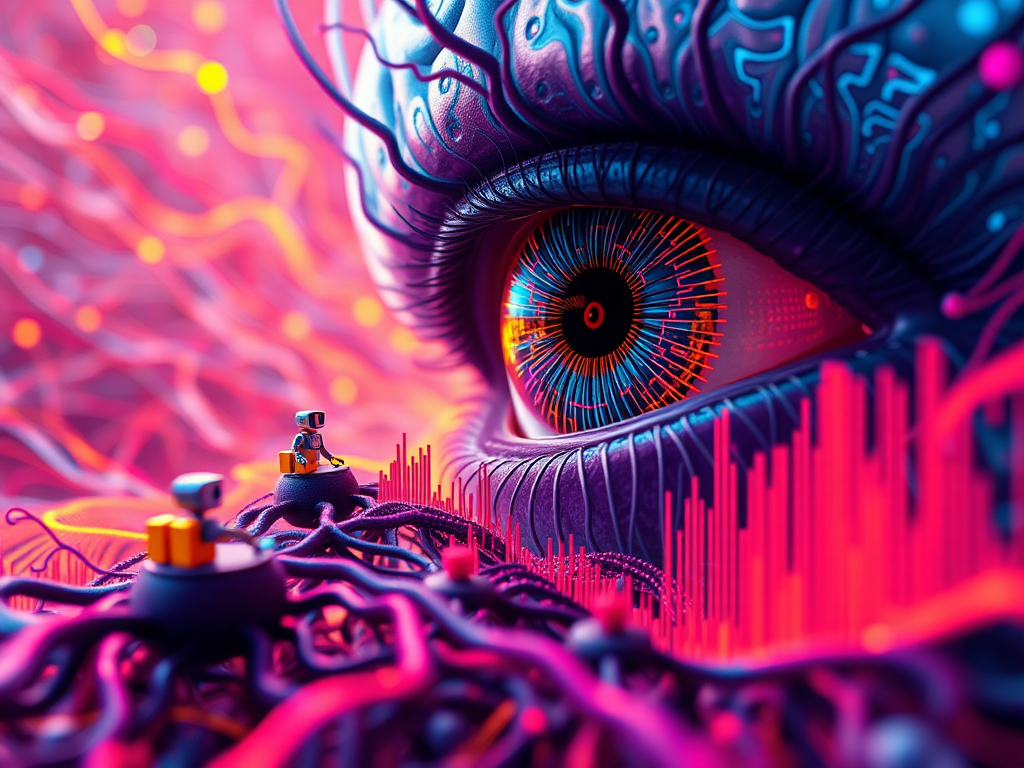
Unleashing the Infinite Possibilities of Data Visualization with Python
This article introduces the application of Python in the field of data visualization, discusses the basic usage of the Matplotlib library and common chart types, as well as how to combine Pandas for data processing and visualization. It also provides tips for chart beautification, helping readers create professional-level data visualization works.