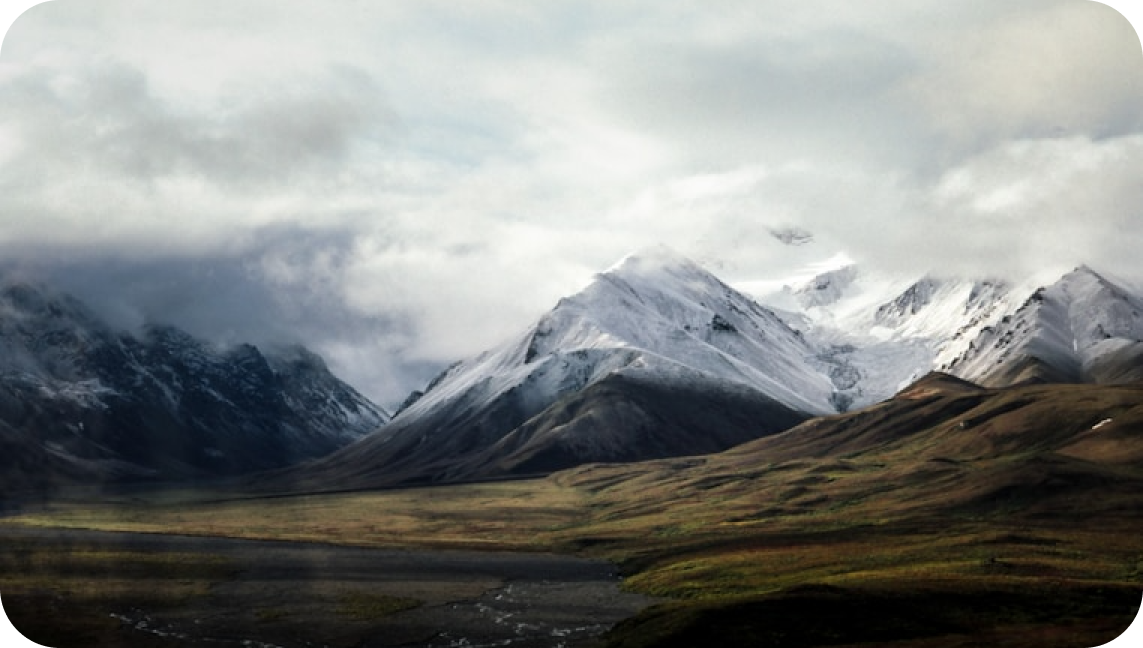
Introduction
Hello, Python enthusiasts! Today, let's talk about the hot topic of data visualization. I'm sure many of you have had this experience: faced with piles of data, looking at dense numbers and text, your head becomes a jumble. However, as soon as you transform this dry data into intuitive charts, it immediately becomes clear, letting the data "speak" for you.
Data visualization not only helps us better understand data but also has wide applications in many fields, such as scientific research, business analysis, news reporting, and more. Python, as an expressive programming language, provides powerful support for data visualization. Today, let's explore the mysteries of Python data visualization together!
Matplotlib
When it comes to Python data visualization, we can't ignore Matplotlib, a time-tested helper. Matplotlib is the most famous plotting library in Python, capable of generating publication-quality graphics. However, Matplotlib's learning curve is quite steep and might be intimidating for beginners. Don't worry, with my step-by-step explanation, you'll soon master the essence of Matplotlib.
Appetizers
Come on, let's start with some simple examples. Have you ever thought about creating beautiful line charts, scatter plots, and histograms with just a few lines of code? Follow me!
import matplotlib.pyplot as plt
import numpy as np
x = np.linspace(0, 10, 100)
y = np.sin(x)
plt.plot(x, y)
plt.show()
x = np.random.rand(100)
y = np.random.rand(100)
plt.scatter(x, y)
plt.show()
a = np.random.randn(1000)
plt.hist(a, bins=20)
plt.show()
Isn't it simple? Just import the Matplotlib and NumPy libraries, call a few functions, and you can generate various common statistical graphs. However, this is just the tip of the iceberg; Matplotlib's capabilities are incredibly powerful.
Customization
Everyone may have different aesthetic requirements for charts. Fortunately, Matplotlib provides countless customization options to make your charts more vivid. For example, we can customize line colors, styles, adjust axis ranges, labels, and so on.
plt.figure(figsize=(8, 6)) # Set canvas size
plt.plot(x, y, 'r--', linewidth=2, label='Sine Wave') # Red dashed line, line width 2
plt.xlabel('X')
plt.ylabel('Sine of X')
plt.title('A Sine Wave')
plt.axis([-1, 11, -1.5, 1.5]) # x-axis range [-1, 11], y-axis range [-1.5, 1.5]
plt.legend()
plt.show()
Don't you feel this chart has more quality now? Matplotlib's customization capabilities are so powerful, you can play with it however you want!
Time Handling
In data visualization, we often encounter time series data. Matplotlib also provides good support for this type of data. However, it's worth noting that when dealing with individual time values, we must first convert them to Python's datetime
objects.
import datetime
dates = [datetime.datetime(2023, 5, 1),
datetime.datetime(2023, 5, 2),
datetime.datetime(2023, 5, 3)]
values = [20, 18, 22]
plt.plot_date(dates, values)
plt.gcf().autofmt_xdate() # Automatically rotate date labels to avoid overlap
plt.show()
Isn't it cool? Matplotlib automatically recognized the time data and correctly labeled the dates on the x-axis. Of course, if your time series data has large intervals, you might need to do some additional date formatting. But don't worry, with Matplotlib, it's all so easy!
Pandas Support
During the data visualization process, we usually need to clean, transform, and process the data first. As a powerful tool for Python data analysis, Pandas is excellent in this regard. Perfectly combining Pandas with Matplotlib can unleash unlimited possibilities for data visualization!
Data Processing
Suppose we have a dataset that includes vehicle speeds and congestion situations on different streets at different times:
import pandas as pd
data = {'Street': ['Main St', 'Main St', 'Maple Ave', 'Maple Ave'],
'Time': ['09:00', '10:00', '09:00', '10:00'],
'Speed': [30, 35, 40, 30],
'Congestion': [2, 1, 1, 3]}
traffic = pd.DataFrame(data)
With Pandas, operations like grouping, filtering, and sorting on this data become a piece of cake. For example, we can group the data by street name like this:
grouped = traffic.groupby('Street')
Visualizing Grouped Data
Now, let's visualize this grouped data! We can plot the speed changes over time for each street on the same subplot:
import matplotlib.pyplot as plt
fig, ax = plt.subplots(figsize=(8, 6))
for street, group in grouped:
group.plot(x='Time', y='Speed', label=street, ax=ax)
ax.set_xlabel('Time')
ax.set_ylabel('Speed (mph)')
ax.set_title('Traffic Speed by Street')
ax.legend()
plt.show()
Look, isn't it clear at a glance? From the graph, we can clearly see that at 10 AM, the speed on Main Street is faster than on Maple Avenue. If you want to analyze the congestion situation in depth, just replace y='Speed'
with y='Congestion'
.
Advanced Techniques
After mastering the basics, it's time to upgrade our skills. Let's explore some more advanced data visualization techniques to make your charts even more impressive!
Multi-dimensional Visualization
Sometimes, we need to display multiple data series on the same chart for comparative analysis. In this case, we can use Matplotlib's subplot functionality.
fig, axs = plt.subplots(2, 1, figsize=(8, 6))
traffic.plot(x='Time', y='Speed', label='Speed', ax=axs[0])
axs[0].set_title('Traffic Speed')
axs[0].legend()
traffic.plot(x='Time', y='Congestion', label='Congestion', ax=axs[1], kind='bar')
axs[1].set_title('Traffic Congestion')
axs[1].legend()
plt.tight_layout()
plt.show()
Amazing, right? We've displayed data from two dimensions - speed and congestion level - on one chart. The upper half is a line chart, and the lower half is a bar chart. This way, we can analyze traffic conditions more comprehensively.
Adding Annotations
Sometimes, adding some annotations to the chart can make data visualization more vivid. For example, we can use arrows to point out a certain value, or use text to mark a special data point.
fig, ax = plt.subplots(figsize=(8, 6))
ax.plot(traffic['Time'], traffic['Speed'])
ax.annotate('Peak Speed', xy=('10:00', 35), xytext=(9.7, 38),
arrowprops=dict(facecolor='black', shrink=0.05))
ax.text('09:30', 32, 'Slow Speed', fontsize=12,
bbox=dict(boxstyle='round', facecolor='wheat', alpha=0.5))
plt.show()
Look, I used an arrow to point out the peak speed point, and used a text annotation box to mark a slower speed. Doesn't it feel like the chart has come "alive"?
Performance Optimization
After discussing so many data visualization techniques, we shouldn't ignore performance issues. Especially when dealing with large-scale datasets, memory management and performance optimization become particularly important.
Memory Management
Python has an automatic memory management mechanism, so most of the time we don't need to worry too much. But if you find your program running slowly or consuming too much memory, you need to check if there are memory leak issues.
Python provides the gc
module to help us manually manage memory. We can use gc.get_objects()
to get all objects tracked by Python, and use the getsizeof
function to check the memory size occupied by objects.
import gc
import sys
big_object = ['Hello' for _ in range(1000000)]
found_objects = gc.get_objects()
print(f"Found {len(found_objects)} objects")
for obj in found_objects[:10]:
try:
if sys.getsizeof(obj) >= 1000:
print(f"Object of type {type(obj)} has size {sys.getsizeof(obj)}")
except:
pass
The above code creates a large list, then iterates through all objects tracked internally by Python, printing information about objects larger than 1000 bytes. In this way, we can discover and deal with those "memory black holes" that occupy too much memory.
Using gc
In addition to viewing object information, we can also manually call the garbage collector to free up memory space occupied by objects that are no longer in use.
import gc
del_objs = [obj for obj in gc.get_objects() if type(obj) == list]
collected = gc.collect()
print(f"Collected {collected} objects")
In the above code, we first get all list-type objects, then manually call the gc.collect()
function for garbage collection. Finally, we print the number of collected objects.
By properly using the gc
module, we can minimize memory leaks and improve the running efficiency of our program.
Summary
Alright, our journey into Python data visualization ends here. We started from the basics of Matplotlib, explored advanced techniques, and finally touched on performance optimization.
I believe that through today's explanation, you now have a preliminary understanding of Python data visualization. However, this is just the beginning; the ocean of data visualization is vast, and we've only explored a small bay. The road ahead is still long, but as long as you persevere, you'll be able to navigate it with ease!
Are you ready to continue learning in depth? If there's anything you don't understand, feel free to ask me at any time! I look forward to sailing with you in the wave of data visualization!
Next
Mastering Data Visualization in Python: A Complete Guide to Matplotlib
A comprehensive guide exploring data visualization fundamentals in Python, covering core concepts, visualization types, and practical implementations using popular libraries like Matplotlib, Seaborn, and Plotly, with detailed examples and use cases
Overview of Python Data Visualization
Explore the field of Python data visualization, introducing the characteristics and applications of mainstream libraries such as Matplotlib, Bokeh, and Holoviz.
Data Visualization: Making Your Data "Speak"
This article delves into Python data visualization techniques, including basic usage of the Matplotlib library, Pandas data processing, advanced plotting techniques, and performance optimization methods, providing readers with a comprehensive introduction to Python data visualization
Next
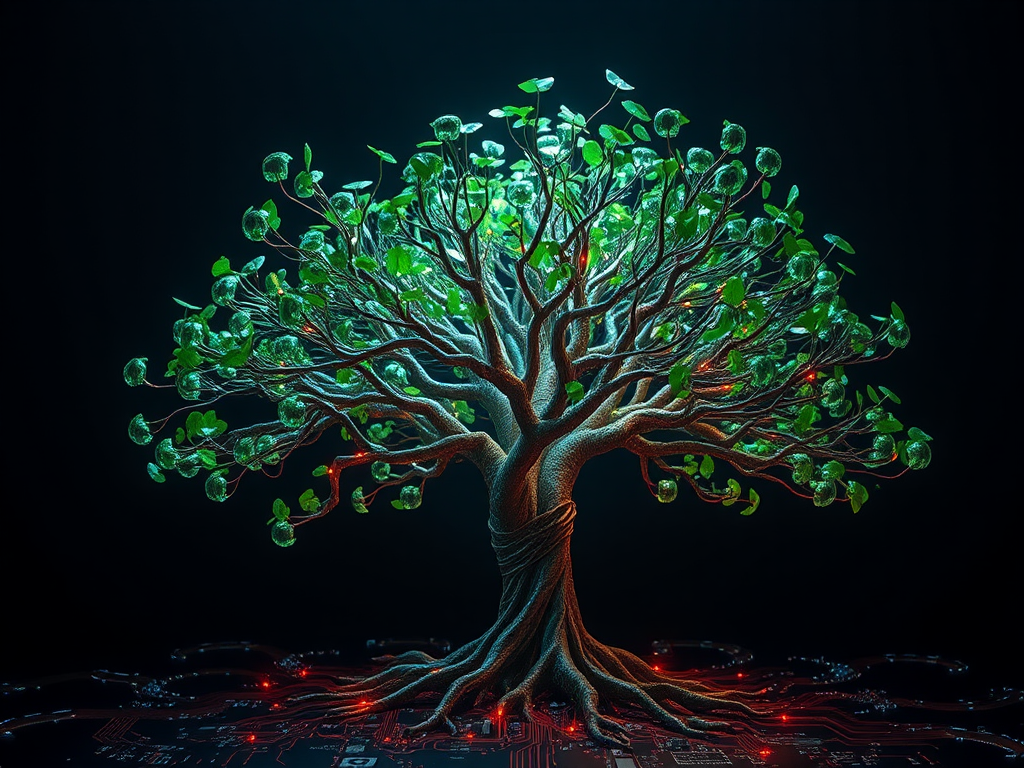
Mastering Data Visualization in Python: A Complete Guide to Matplotlib
A comprehensive guide exploring data visualization fundamentals in Python, covering core concepts, visualization types, and practical implementations using popular libraries like Matplotlib, Seaborn, and Plotly, with detailed examples and use cases
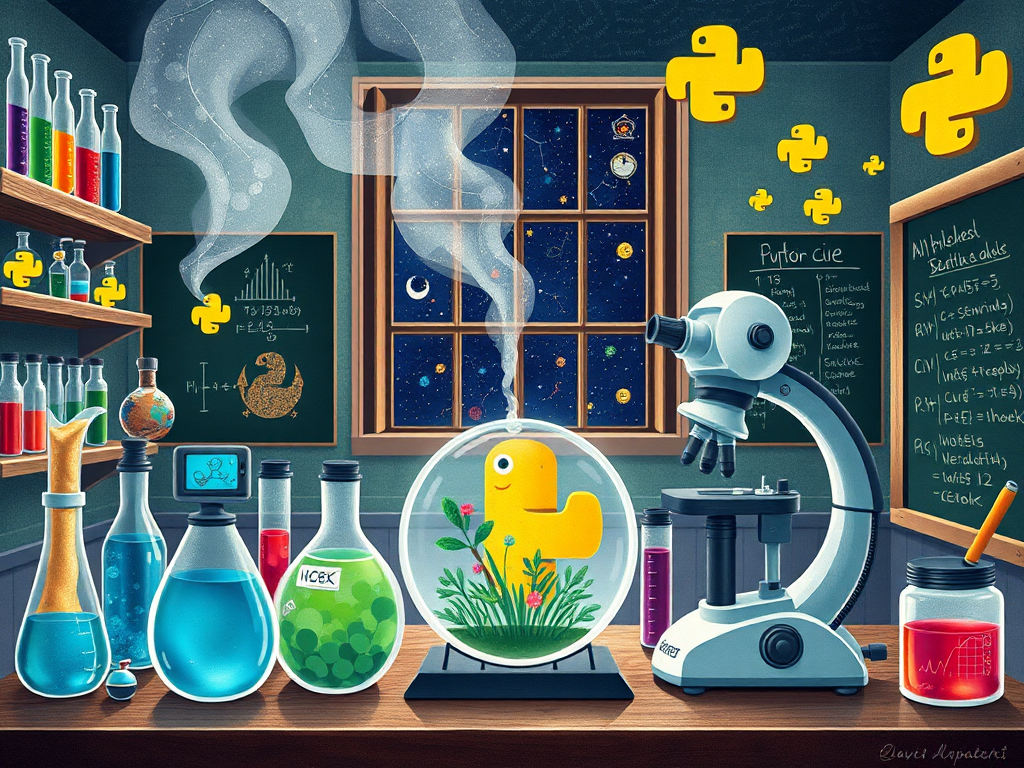
Overview of Python Data Visualization
Explore the field of Python data visualization, introducing the characteristics and applications of mainstream libraries such as Matplotlib, Bokeh, and Holoviz.
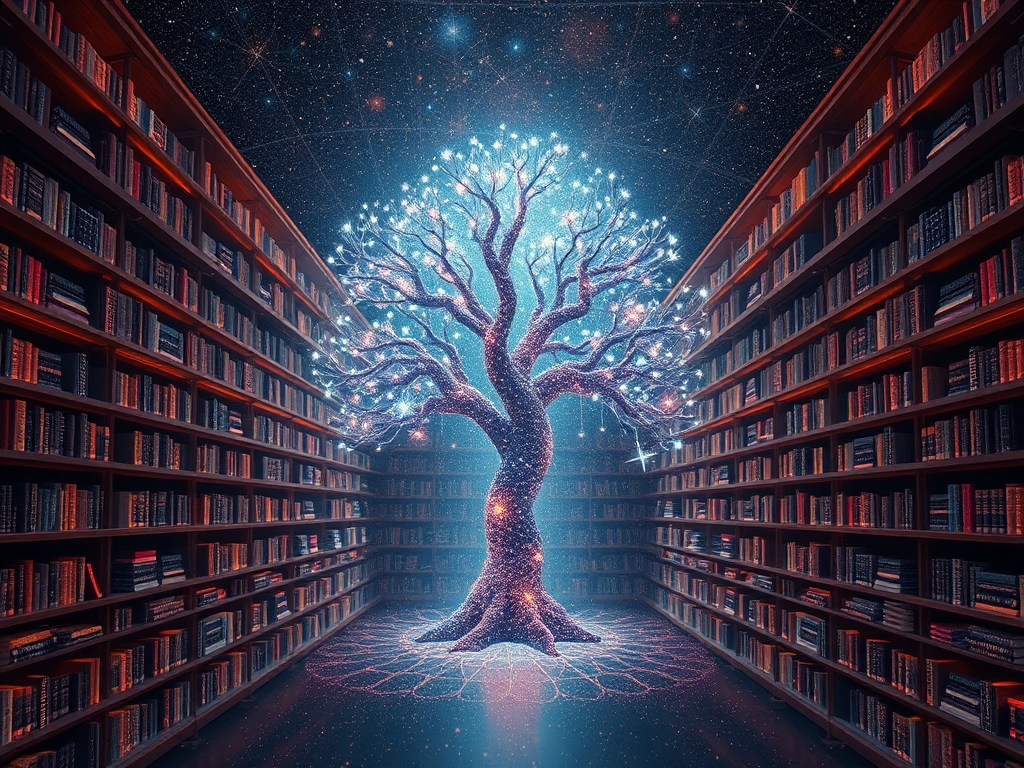
Data Visualization: Making Your Data "Speak"
This article delves into Python data visualization techniques, including basic usage of the Matplotlib library, Pandas data processing, advanced plotting techniques, and performance optimization methods, providing readers with a comprehensive introduction to Python data visualization