Hello, Python enthusiasts! Today we're going to talk about containerizing Python applications. With the popularization of container technology, more and more developers are starting to package Python applications into containers for deployment and running. So, what benefits can containerization bring to our Python development? And what are some things we need to pay attention to? Let's explore together!
Containerization
First, let's briefly review what containerization is. Containerization technology can package an application and all its dependencies into an independent unit. This unit is what we commonly refer to as a container. Containers can run on any system that supports container runtime, without worrying about problems caused by environmental differences.
For Python developers, using containers has many benefits:
-
Environmental consistency: Are you still troubled by "it works on my computer"? With containers, you can ensure consistency across development, testing, and production environments.
-
Rapid deployment: Containers can be quickly started and stopped, greatly improving the efficiency of application deployment.
-
Resource isolation: Containers are isolated from each other and do not affect each other, which is especially useful for microservice architectures.
-
Version control: Container images can be versioned, making it easy to rollback and manage different versions of applications.
So, how should we begin our journey of containerizing Python applications? Let's look at it step by step.
Environment Configuration
Python Environment Setup in Docker Containers
First, we need to set up the Python environment in Docker containers. Here's a little tip: choosing the right base image is very important. I usually choose the official Python image, such as python:3.9-slim
. This image contains the Python runtime while being relatively small in size, making it very suitable as a base image.
Let's look at a simple Dockerfile example:
FROM python:3.9-slim
WORKDIR /app
COPY requirements.txt .
RUN pip install --no-cache-dir -r requirements.txt
COPY . .
CMD ["python", "app.py"]
This Dockerfile does the following:
- Uses Python 3.9 slim version as the base image
- Sets the working directory to /app
- Copies the requirements.txt file and installs dependencies
- Copies all source code
- Sets the startup command
What do you think of this Dockerfile? Isn't it concise and clear? However, this is just a basic version. In actual development, we may need to adjust according to the specific needs of the project. For example, if your application needs some system-level dependencies, you may need to add apt-get install
commands in the Dockerfile to install these dependencies.
Poetry Environment Management and Dependency Isolation
Speaking of dependency management, have you used Poetry? I personally think Poetry is a great tool that can not only help us manage project dependencies but also create and manage virtual environments. In the context of containerization, Poetry can also play its role.
One advantage of using Poetry is that it can help us generate an accurate dependency lock file (poetry.lock), which ensures that exactly the same versions of dependencies are installed in different environments. In Dockerfile, we can use Poetry like this:
FROM python:3.9-slim
WORKDIR /app
RUN pip install poetry
COPY pyproject.toml poetry.lock ./
RUN poetry config virtualenvs.create false \
&& poetry install --no-dev --no-interaction --no-ansi
COPY . .
CMD ["python", "app.py"]
This Dockerfile first installs Poetry, then uses Poetry to install project dependencies. Note that we set virtualenvs.create false
because in containers, we usually don't need to create virtual environments.
You might ask, why use Poetry instead of directly using pip? I think Poetry's advantage lies in providing better dependency resolution and management functions. For example, it can automatically handle dependency conflicts and distinguish between development dependencies and production dependencies. In collaborative projects, these features can save us a lot of trouble.
Containerization of Common Frameworks and Tools
Containerized Deployment of FastAPI Applications
Speaking of Python web frameworks, FastAPI has been very popular recently! It's not only fast but also comes with API documentation, making it very convenient to use. So, how do we containerize FastAPI applications?
Here's a simple example:
FROM python:3.9-slim
WORKDIR /app
COPY requirements.txt .
RUN pip install --no-cache-dir -r requirements.txt
COPY . .
CMD ["uvicorn", "main:app", "--host", "0.0.0.0", "--port", "8000"]
This Dockerfile looks similar to what we've seen before, but notice the CMD line. We use uvicorn to run the FastAPI application and set the host and port.
However, in actual deployment, we may need to consider more factors. For example, if your FastAPI application needs to connect to a database, you may need to set up database connection information in the container. Or, if your application needs to handle a large number of concurrent requests, you may need to adjust the number of uvicorn workers.
Using Selenium in Docker
When it comes to automated testing, Selenium is an indispensable tool. However, using Selenium in Docker presents some special challenges. The most common problem is that when running Selenium scripts in Docker containers, you may get different results from the local environment.
This problem is usually caused by the network settings or browser configuration of Docker containers. One solution to this problem is to use Selenium Grid. Selenium Grid allows you to run your tests in parallel on multiple machines, which is very suitable for use in containerized environments.
Here's an example docker-compose.yml using Selenium Grid:
version: '3'
services:
chrome:
image: selenium/node-chrome:4.0
shm_size: 2gb
depends_on:
- selenium-hub
environment:
- SE_EVENT_BUS_HOST=selenium-hub
- SE_EVENT_BUS_PUBLISH_PORT=4442
- SE_EVENT_BUS_SUBSCRIBE_PORT=4443
selenium-hub:
image: selenium/hub:4.0
container_name: selenium-hub
ports:
- "4442:4442"
- "4443:4443"
- "4444:4444"
This configuration file defines two services: a Chrome node and a Selenium Hub. The Chrome node is used to actually run tests, while the Selenium Hub is responsible for coordinating test tasks.
In your Python script, you can connect to Selenium Grid like this:
from selenium import webdriver
from selenium.webdriver.common.desired_capabilities import DesiredCapabilities
driver = webdriver.Remote(
command_executor='http://selenium-hub:4444/wd/hub',
desired_capabilities=DesiredCapabilities.CHROME
)
Using this method, you can run Selenium tests in a Docker environment. And because Grid is used, you can easily expand to multiple browser nodes for parallel testing.
Best Practices for Containerizing Python Applications
Docker Configuration Optimization
After using Docker for a while, you may find that some areas can be further optimized. Here are a few Docker configuration optimization tips I often use:
-
Use multi-stage builds: This can help you reduce the size of the final image. For example, you can compile dependencies in one stage and then only copy the compiled files in another stage.
-
Arrange Dockerfile layers reasonably: Put instructions that don't change often (such as installing system dependencies) at the front of the Dockerfile, which can better utilize Docker's caching mechanism.
-
Use .dockerignore file: This can prevent unnecessary files from being copied into the Docker image, thereby reducing the image size.
-
Choose the appropriate base image: For Python applications, the slim version of the image is usually sufficient, and there's no need to use the full version of the image.
-
Run applications as non-root users: This can improve the security of containers.
Here's an example of an optimized Dockerfile:
FROM python:3.9-slim as builder
WORKDIR /app
RUN apt-get update && apt-get install -y --no-install-recommends gcc
COPY requirements.txt .
RUN pip install --user -r requirements.txt
FROM python:3.9-slim
WORKDIR /app
COPY --from=builder /root/.local /root/.local
COPY . .
ENV PATH=/root/.local/bin:$PATH
RUN useradd -m myuser
USER myuser
CMD ["python", "app.py"]
This Dockerfile uses multi-stage builds, installing all dependencies in the build stage, and then only copying the necessary files in the run stage. It also creates a non-root user to run the application.
Managing Multi-Container Applications with Docker Compose
When your application becomes complex and requires multiple services to work together, Docker Compose comes in handy. Docker Compose allows you to define and run multiple Docker containers with a YAML file.
Here's a simple docker-compose.yml example that defines a Python application and a PostgreSQL database:
version: '3'
services:
web:
build: .
ports:
- "5000:5000"
environment:
- DATABASE_URL=postgresql://user:password@db/mydb
depends_on:
- db
db:
image: postgres:13
environment:
- POSTGRES_USER=user
- POSTGRES_PASSWORD=password
- POSTGRES_DB=mydb
With this configuration file, you can start the entire application with one command:
docker-compose up
Docker Compose not only simplifies the management of multi-container applications but also provides some useful features such as inter-service dependency management and network configuration. Using Docker Compose in the development environment can make it easier for you to simulate the production environment.
Debugging and Problem Solving
Solving Network Problems in Containerized Environments
In containerized environments, network problems are one of the most common issues. For example, you may encounter situations where containers cannot communicate with each other, or containers cannot access the external network.
The key to solving these problems is understanding Docker's network model. Docker provides several network drivers, with the bridge network being the most commonly used. In a bridge network, each container has its own IP address and can communicate with each other through this IP address.
If you use Docker Compose, by default all services will be placed in the same network and can access each other through the service name. For example, in the above example, the web service can access the database service through the hostname "db".
For the problem of containers not being able to access the external network, it's usually a DNS configuration issue. You can try adding Google's DNS servers in the Docker daemon's configuration file:
{
"dns": ["8.8.8.8", "8.8.4.4"]
}
Handling Behavioral Differences Inside and Outside Containers
Sometimes, you may find that an application runs fine in the local environment, but problems occur when put into a container. In this case, we need to carefully compare the environmental differences inside and outside the container.
A common reason is different timezone settings. In Dockerfile, you can set the timezone like this:
ENV TZ=Asia/Shanghai
RUN ln -snf /usr/share/zoneinfo/$TZ /etc/localtime && echo $TZ > /etc/timezone
Another possible reason is file permission issues. In containers, applications may run as different users, resulting in inability to access certain files. One way to solve this problem is to explicitly set file permissions in the Dockerfile:
COPY --chown=myuser:myuser . .
Finally, if you really can't find where the problem is, you can try entering the container for debugging. Use the following command to start an interactive shell:
docker exec -it container_name /bin/bash
Inside the container, you can view environment variables, check file permissions, test network connections, etc., all of which can help you find out where the problem lies.
Conclusion
Alright, our journey into Python containerization ends here. We've discussed everything from basic environment configuration to containerization of common frameworks, and then to some best practices and problem-solving techniques. I hope this content has been helpful to you!
Containerization technology is changing the way we develop and deploy applications. As Python developers, mastering this technology can make our work more efficient and make our applications easier to deploy and scale.
Do you have any experiences or questions about Python containerization? Feel free to share your thoughts in the comments section! Let's learn and progress together.
Remember, technology is constantly evolving, so keep your enthusiasm for learning. See you next time!
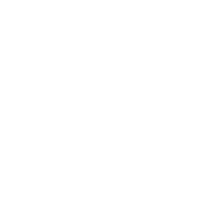
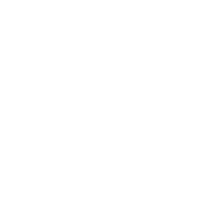