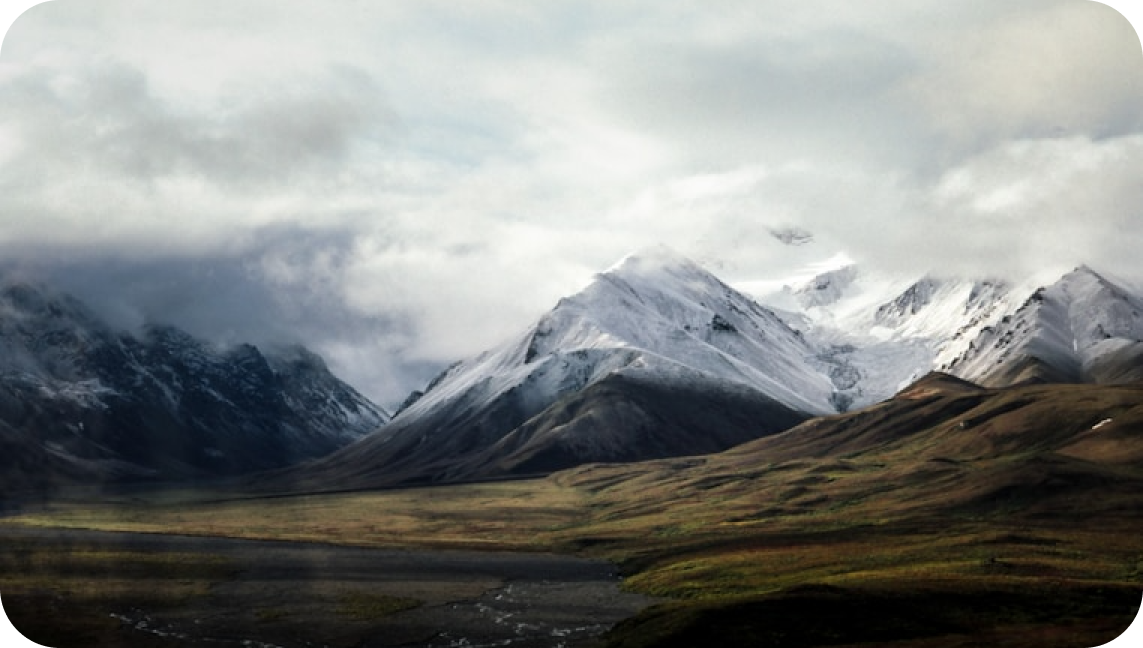
Introduction
Have you ever encountered situations where your Python program runs perfectly on your computer but throws various errors when deployed to a server? Or perhaps you developed a great application, but when sharing it with colleagues, they always complain about complex environment configuration? These issues can actually be solved through containerization technology.
As a Python developer, I deeply appreciate the convenience that containerization brings to development. Today, I'll share five key tips I've summarized from practice to help you better containerize your Python applications.
Basics
Before diving into specific tips, let's understand what containerization is. Simply put, a container is like a standardized package that bundles your application with all its dependencies. This ensures consistent behavior regardless of the environment where it runs.
You can think of a container as a "mini" operating system, but it's much lighter than traditional virtual machines. Why? Because containers directly share the host machine's operating system kernel, rather than running a complete operating system like virtual machines do. This makes containers start faster and consume fewer resources.
Tips
Image Optimization
The first tip I want to share is how to build optimized images. I've seen too many developers directly using the official full Python image as a base, which results in particularly large final images. We can actually use the alpine version of the base image, which is less than 100MB.
FROM python:3.9
FROM python:3.9-alpine
Using the alpine version not only saves storage space but also improves image download and deployment speed. However, note that alpine images lack some common system libraries, so you'll need to install them yourself if your application depends on them.
Dependency Management
The second tip is about dependency management. I recommend using multi-stage builds in Dockerfile to separate dependency installation from application building:
FROM python:3.9-alpine as builder
WORKDIR /install
COPY requirements.txt .
RUN pip install --prefix=/install -r requirements.txt
FROM python:3.9-alpine
COPY --from=builder /install /usr/local
COPY . .
CMD ["python", "app.py"]
The advantage of this approach is that the final image only contains files necessary for runtime, excluding temporary files from the build process.
Cache Optimization
The third tip is about properly utilizing Docker's cache mechanism. I notice many developers like to write all commands together, causing all steps to be re-executed with each build. Actually, we can organize the Dockerfile like this:
FROM python:3.9-alpine
WORKDIR /app
COPY requirements.txt .
RUN pip install -r requirements.txt
COPY . .
CMD ["python", "app.py"]
This way, as long as requirements.txt hasn't changed, the pip install layer will use cache, greatly improving build speed.
Environment Configuration
The fourth tip is about using environment variables. I recommend using environment variables for configuration rather than hardcoding in the code:
import os
DATABASE_URL = os.getenv('DATABASE_URL', 'postgresql://localhost:5432/db')
DEBUG = os.getenv('DEBUG', 'False').lower() == 'true'
Then set default values in Dockerfile:
ENV DATABASE_URL=postgresql://localhost:5432/db
ENV DEBUG=False
This makes the application easier to configure and better aligns with twelve-factor app principles.
Health Checks
The final tip is adding health checks. I often see containerized applications that are running but not actually working properly. Adding health checks helps detect problems early:
HEALTHCHECK --interval=30s --timeout=3s \
CMD curl -f http://localhost:8000/health || exit 1
Practice
Let's look at a complete example. Suppose we have a simple Flask application:
from flask import Flask
import os
app = Flask(__name__)
@app.route('/')
def hello():
return 'Hello, Docker!'
@app.route('/health')
def health():
return 'OK'
if __name__ == '__main__':
port = int(os.getenv('PORT', 8000))
app.run(host='0.0.0.0', port=port)
The corresponding Dockerfile can be written as:
FROM python:3.9-alpine as builder
WORKDIR /install
COPY requirements.txt .
RUN pip install --prefix=/install -r requirements.txt
FROM python:3.9-alpine
WORKDIR /app
COPY --from=builder /install /usr/local
COPY . .
ENV PORT=8000
EXPOSE 8000
HEALTHCHECK --interval=30s --timeout=3s \
CMD wget --no-verbose --tries=1 --spider http://localhost:8000/health || exit 1
CMD ["python", "app.py"]
This Dockerfile incorporates all the tips we discussed earlier.
Summary
Containerization technology greatly simplifies the deployment and distribution of Python applications. By properly using these tips, you can build more lightweight, reliable, and maintainable containerized applications.
Have you encountered any interesting problems when containerizing Python applications? Or do you have other useful tips to share? Feel free to discuss in the comments.
Extension
Speaking of containerization, we can discuss more related topics:
- How to use Docker Compose to manage multi-container applications?
- How to implement container monitoring and log collection in production environments?
- What are the best practices for CI/CD of containerized applications?
These topics are all very interesting. Which one interests you the most? We can continue to explore in future articles.
Next
Python Containerization Deployment in Practice: A Complete Guide to Dockerfile Writing from Beginner to Expert
A comprehensive guide on containerizing Python applications with Docker, covering infrastructure setup, dependency management strategies, and multi-stage build implementations, with focus on handling complex dependencies
Five Key Tips for Containerizing Python Applications to Make Your Code Run Anywhere
An in-depth exploration of integrating Python applications with containerization technology, covering container fundamentals, working principles, and practical implementation methods in Python projects for efficient application deployment
Python Containerization in Practice: Best Practices Guide from Development to Production
A comprehensive guide to Python application containerization, covering Docker configuration, Kubernetes deployment, image optimization, security policies, monitoring systems, and log management, providing development teams with complete containerization practices
Next
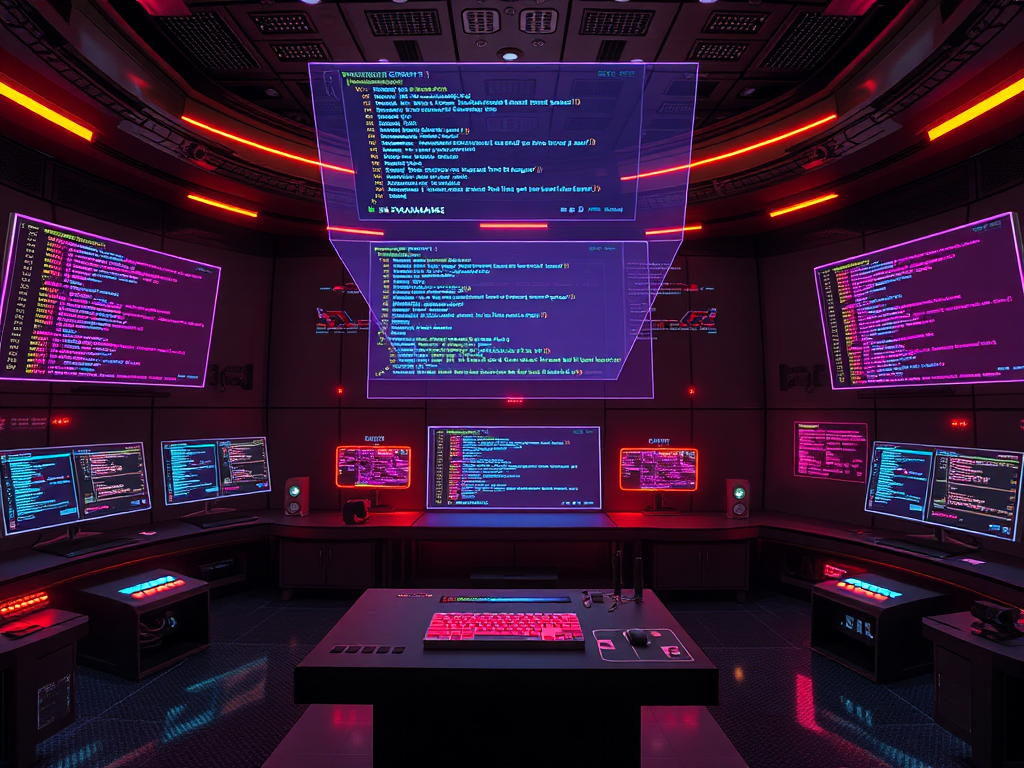
Python Containerization Deployment in Practice: A Complete Guide to Dockerfile Writing from Beginner to Expert
A comprehensive guide on containerizing Python applications with Docker, covering infrastructure setup, dependency management strategies, and multi-stage build implementations, with focus on handling complex dependencies
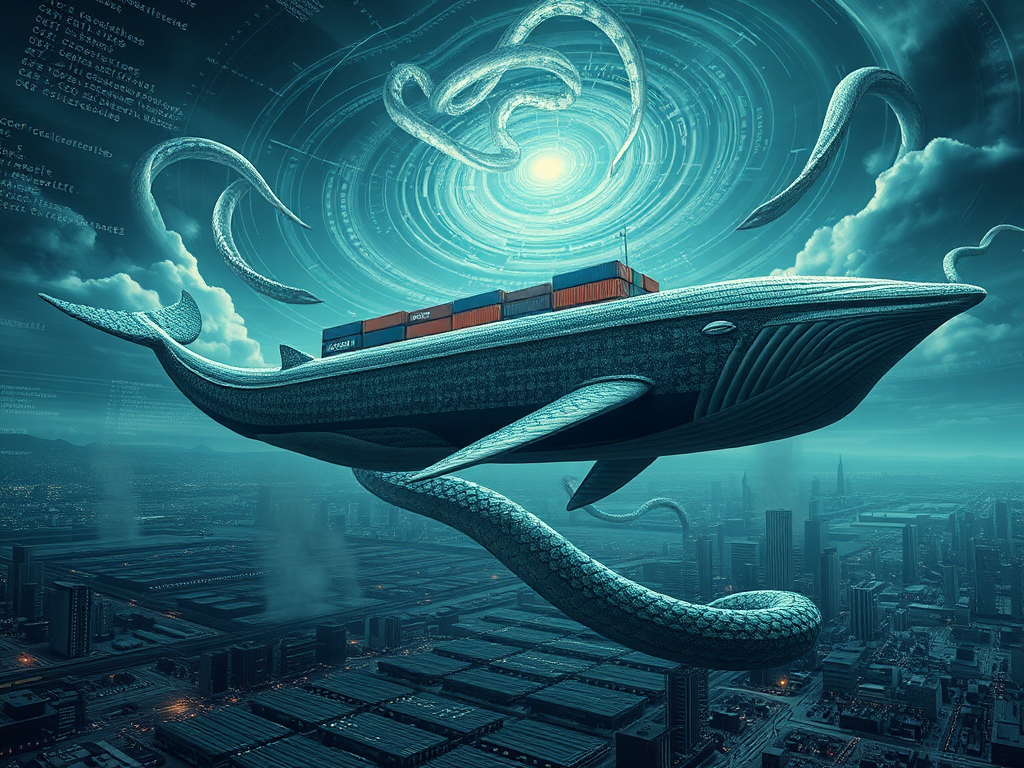
Five Key Tips for Containerizing Python Applications to Make Your Code Run Anywhere
An in-depth exploration of integrating Python applications with containerization technology, covering container fundamentals, working principles, and practical implementation methods in Python projects for efficient application deployment
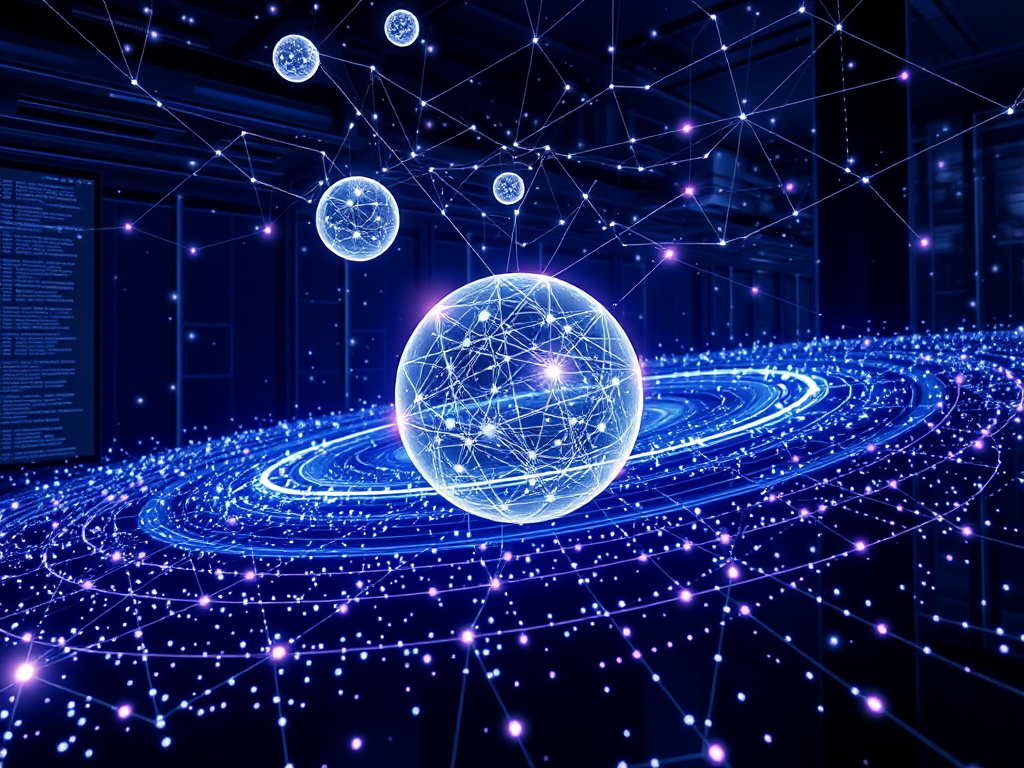
Python Containerization in Practice: Best Practices Guide from Development to Production
A comprehensive guide to Python application containerization, covering Docker configuration, Kubernetes deployment, image optimization, security policies, monitoring systems, and log management, providing development teams with complete containerization practices