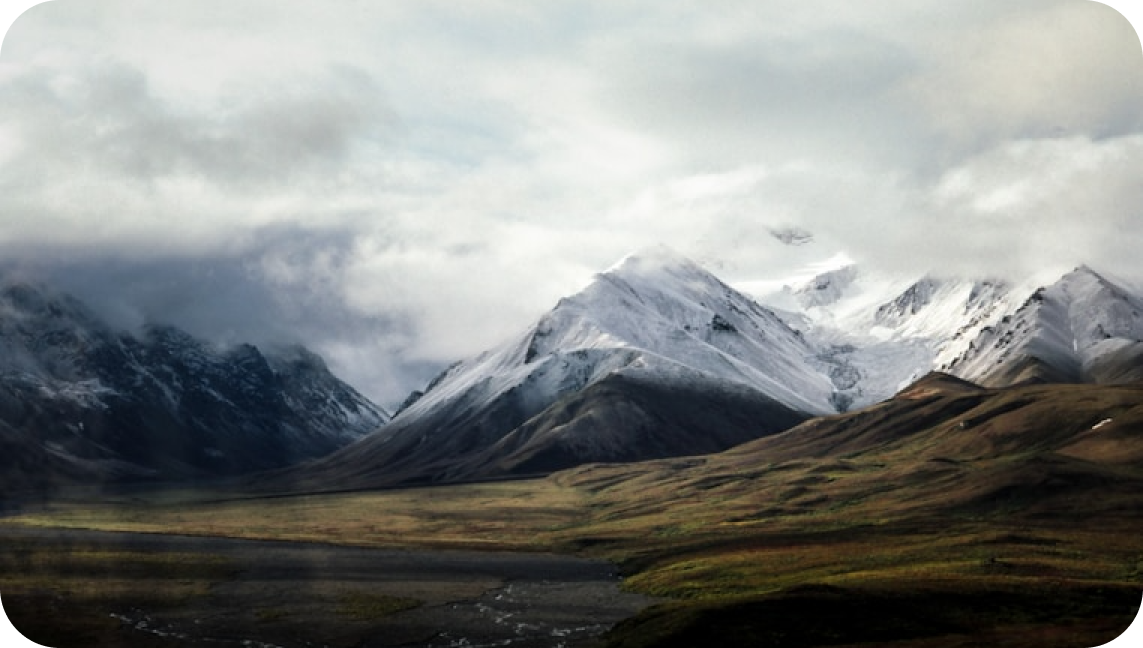
Introduction
Hello! Today I'd like to talk about containerizing Python projects. As a developer who frequently works with Docker, I deeply understand the importance of an excellent containerization solution for projects. Let's explore together how to build an efficient and elegant Python containerized environment.
Basic Selection
When it comes to containerization, the first consideration is choosing the base image. Did you know that Python officially provides multiple versions of base images, such as python:3.9, python:3.9-slim, python:3.9-alpine, etc.? I particularly recommend using the slim version. Why? Because it strikes a great balance between image size and functionality.
Let's look at a specific example:
FROM python:3.9-slim-buster
WORKDIR /app
ENV PYTHONUNBUFFERED=1 \
PYTHONDONTWRITEBYTECODE=1 \
PIP_NO_CACHE_DIR=1
RUN apt-get update && apt-get install -y --no-install-recommends \
build-essential \
&& rm -rf /var/lib/apt/lists/*
This code sets some important environment variables: PYTHONUNBUFFERED ensures real-time Python output, PYTHONDONTWRITEBYTECODE prevents generating pyc files, and PIP_NO_CACHE_DIR avoids pip cache taking up extra space.
Dependency Management
Regarding dependency management, I think there's an important concept to share with you: dependency management isn't just about writing a simple requirements.txt file. We need to consider dependency version locking, separation of build-time and runtime dependencies, and other issues.
Let's look at this more complete example:
FROM python:3.9-slim-buster as builder
WORKDIR /app
COPY requirements.txt requirements-dev.txt ./
RUN python -m venv /opt/venv && \
/opt/venv/bin/pip install --no-cache-dir -r requirements.txt && \
/opt/venv/bin/pip install --no-cache-dir -r requirements-dev.txt
FROM python:3.9-slim-buster as runtime
WORKDIR /app
COPY --from=builder /opt/venv /opt/venv
ENV PATH="/opt/venv/bin:$PATH"
COPY . .
CMD ["python", "app.py"]
I particularly want to emphasize this multi-stage build approach. By separating the build environment from the runtime environment, we can significantly reduce the final image size. In the builder stage, we install all dependencies; in the runtime stage, we only copy the necessary files and virtual environment.
Optimization Strategies
Speaking of optimization, I have some practical experience to share with you. First is the layered installation of dependencies:
FROM python:3.9-slim-buster
WORKDIR /app
COPY requirements-base.txt .
RUN pip install --no-cache-dir -r requirements-base.txt
COPY requirements.txt .
RUN pip install --no-cache-dir -r requirements.txt
COPY . .
This approach utilizes Docker's layer caching mechanism. Think about it - base dependencies like Flask, SQLAlchemy don't change often, so we install them first, allowing these layers to be reused in subsequent builds.
Development Environment Configuration
Development and production environment configurations are often different. Let's see how to handle this:
FROM python:3.9-slim-buster
WORKDIR /app
RUN apt-get update && apt-get install -y --no-install-recommends \
git \
curl \
&& rm -rf /var/lib/apt/lists/*
COPY requirements-dev.txt .
RUN pip install --no-cache-dir -r requirements-dev.txt
ENV FLASK_ENV=development \
FLASK_DEBUG=1
VOLUME ["/app"]
CMD ["flask", "run", "--host=0.0.0.0", "--port=5000"]
This development environment configuration includes debugging tools, hot reload, and other features particularly suitable for local development.
Production Environment Optimization
Production environments have higher requirements for performance and security. Let's look at a production environment configuration example:
FROM python:3.9-slim-buster as builder
WORKDIR /app
RUN apt-get update && apt-get install -y --no-install-recommends \
gcc \
python3-dev \
&& rm -rf /var/lib/apt/lists/*
COPY requirements.txt .
RUN pip wheel --no-cache-dir --no-deps --wheel-dir /app/wheels -r requirements.txt
FROM python:3.9-slim-buster
WORKDIR /app
RUN useradd -m appuser && \
chown -R appuser:appuser /app
COPY --from=builder /app/wheels /wheels
COPY . .
RUN pip install --no-cache /wheels/*
USER appuser
CMD ["gunicorn", "--bind", "0.0.0.0:8000", "app:app"]
This configuration adds several important security features: running the application as a non-root user, minimizing system dependencies, using a production-grade WSGI server, etc.
Debugging Techniques
Debugging Python applications in a containerized environment is also an important topic. Let me share a debugging configuration:
FROM python:3.9-slim-buster
WORKDIR /app
RUN pip install --no-cache-dir debugpy
COPY . .
ENV PYTHONDONTWRITEBYTECODE=1 \
PYTHONUNBUFFERED=1 \
DEBUG=1
EXPOSE 5678
CMD ["python", "-m", "debugpy", "--listen", "0.0.0.0:5678", "--wait-for-client", "app.py"]
This configuration allows us to use VS Code or PyCharm for remote debugging, particularly useful for troubleshooting issues in containers.
Performance Monitoring
For production applications, performance monitoring is essential. Let's see how to configure it:
FROM python:3.9-slim-buster
WORKDIR /app
RUN pip install --no-cache-dir \
prometheus_client \
statsd \
newrelic
COPY . .
COPY newrelic.ini .
ENV NEW_RELIC_CONFIG_FILE=newrelic.ini \
NEW_RELIC_LICENSE_KEY=your_license_key
CMD ["newrelic-admin", "run-program", "gunicorn", "app:app"]
This configuration integrates some common monitoring tools that can help us identify and resolve performance issues promptly.
Summary and Reflection
Through this article, we've discussed various aspects of Python application containerization deployment in detail. From basic environment configuration to production environment optimization, from development environment convenience to production environment security, we've had in-depth discussions.
Have you noticed that containerization deployment isn't just about writing a simple Dockerfile? It involves many aspects of software engineering. I particularly suggest thinking about several questions during practice:
- How to balance image size and functionality completeness?
- How should development and production environment configurations be differentiated?
- How to ensure containerized application security?
- How should performance monitoring and debugging be done?
These questions are worth our deep thought and discussion. What problems have you encountered in practice? Feel free to share your experiences with me.
Finally, I want to say that containerization deployment is a continuous optimization process. As projects evolve, our deployment solutions also need constant adjustment and improvement. I hope this article gives you some inspiration to help you build better Python containerization solutions.
Next
Python Containerization Deployment in Practice: A Complete Guide to Dockerfile Writing from Beginner to Expert
A comprehensive guide on containerizing Python applications with Docker, covering infrastructure setup, dependency management strategies, and multi-stage build implementations, with focus on handling complex dependencies
Five Key Tips for Containerizing Python Applications to Make Your Code Run Anywhere
An in-depth exploration of integrating Python applications with containerization technology, covering container fundamentals, working principles, and practical implementation methods in Python projects for efficient application deployment
A Comprehensive Guide to Python Containerization: From Beginner to Expert
A comprehensive guide exploring the integration of Python programming and containerization technology, covering container fundamentals, Docker build processes, Python containerization practices, and automation management solutions
Next
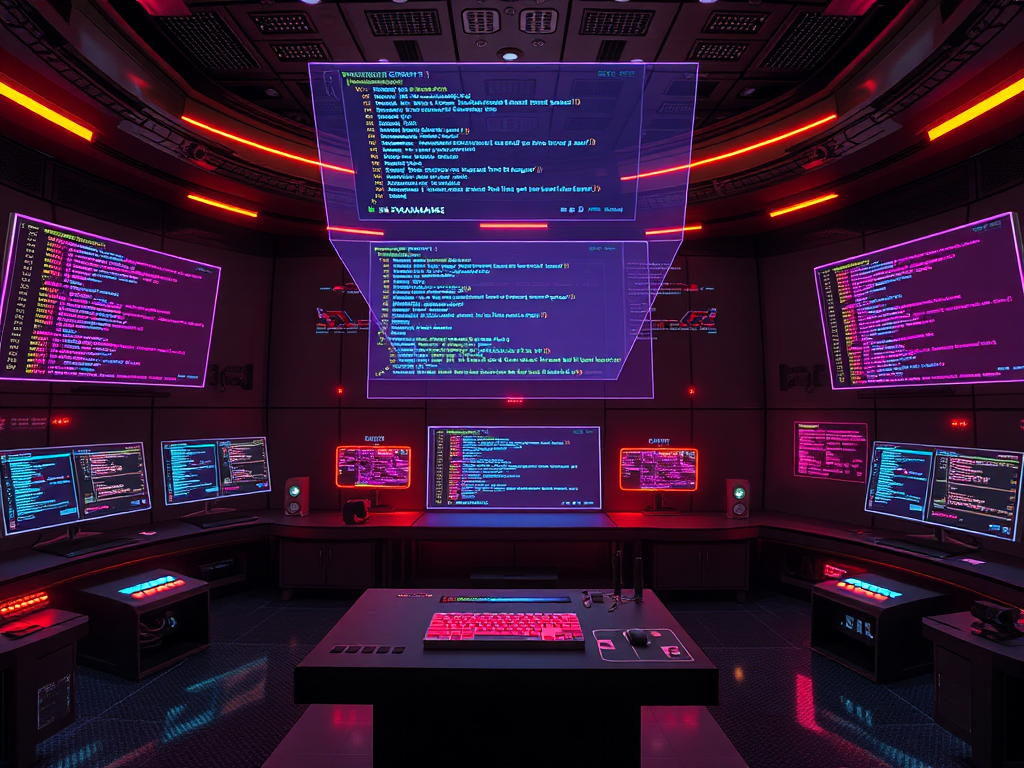
Python Containerization Deployment in Practice: A Complete Guide to Dockerfile Writing from Beginner to Expert
A comprehensive guide on containerizing Python applications with Docker, covering infrastructure setup, dependency management strategies, and multi-stage build implementations, with focus on handling complex dependencies
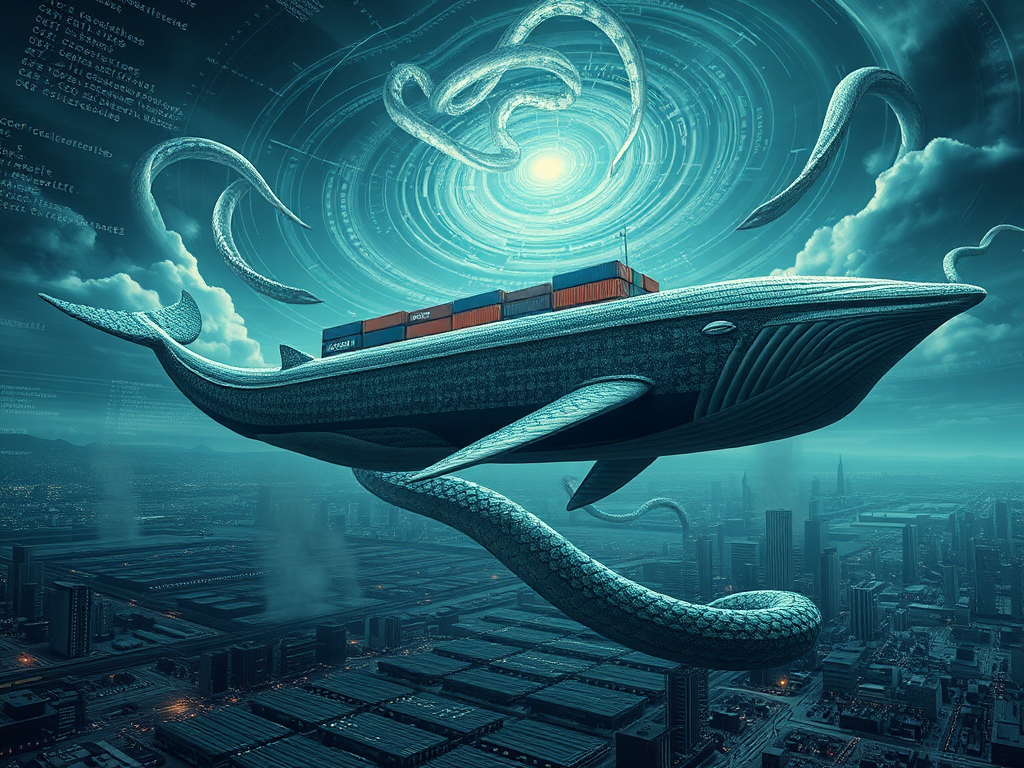
Five Key Tips for Containerizing Python Applications to Make Your Code Run Anywhere
An in-depth exploration of integrating Python applications with containerization technology, covering container fundamentals, working principles, and practical implementation methods in Python projects for efficient application deployment
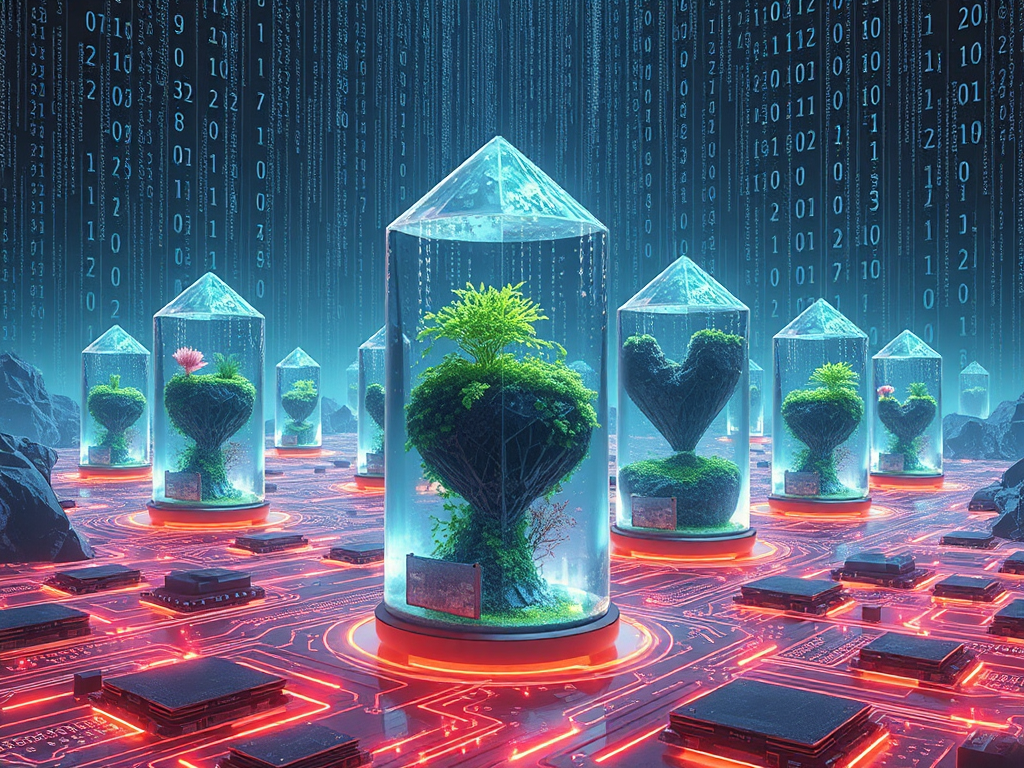
A Comprehensive Guide to Python Containerization: From Beginner to Expert
A comprehensive guide exploring the integration of Python programming and containerization technology, covering container fundamentals, Docker build processes, Python containerization practices, and automation management solutions