Are you tired of manually executing repetitive tasks on the command line? Have you ever wished you could automate certain operational processes? Worry no more, Python is here to save you! As a DevOps engineer, we can leverage Python's powerful features to simplify workflows and increase efficiency. Let's explore the basics and advanced applications of Python in DevOps together!
Automate Everything
The core concept of DevOps is automation and continuous integration/continuous delivery. Python, as an expressive language, can easily integrate with various tools and libraries to help us achieve this goal.
The Magic of Fabric
Want to execute commands on multiple servers? Fabric is your reliable assistant! It allows you to use Python code to connect to remote hosts and execute commands on them. Are you still bothered by writing lengthy Shell scripts?
from fabric import Connection
hosts = ['host1', 'host2']
def deploy():
for host in hosts:
conn = Connection(host)
conn.run('git pull')
conn.run('docker-compose up -d --build')
Look, with just a few lines of Python code, we can implement the function of pulling code and redeploying applications on multiple hosts!
The Magic of Ansible
Ansible is a powerful configuration management and automation tool. Although it's not written in Python itself, we can use Python to call Ansible Playbooks. This provides us with greater flexibility and scalability.
from ansible_runner import run
def run_playbook():
r = run(private_data_dir='/tmp/.ansible', playbook='myplaybook.yml')
print(r.status)
print(r.rc)
See, with just a few lines of Python code, we can run Ansible Playbooks! This is very useful when you need to dynamically generate Playbooks or execute specific tasks based on certain conditions.
The Magic of Docker SDK
Docker has become the de facto standard for container orchestration. Using the Docker SDK for Python, you can interact directly with the Docker engine in Python code, managing containers and images.
import docker
client = docker.from_env()
client.images.build(path='.', tag='myapp')
container = client.containers.run('myapp', detach=True, ports={'8000/tcp': 8000})
In this way, we can integrate the building, running, and management of containers into automated processes, achieving true "infrastructure as code".
The Magic of Kubernetes
As applications continue to scale, Kubernetes has become the go-to choice for orchestrating containers. Fortunately, Python also provides us with client libraries for interacting with the Kubernetes API.
from kubernetes import client, config
config.load_kube_config()
apps_api = client.AppsV1Api()
deployment = client.V1Deployment(
metadata=client.V1ObjectMeta(name="myapp"),
spec=client.V1DeploymentSpec(
replicas=3,
selector=client.V1LabelSelector(
match_labels={"app": "myapp"}
),
template=client.V1PodTemplateSpec(
metadata=client.V1ObjectMeta(
labels={"app": "myapp"}
),
spec=client.V1PodSpec(containers=[
client.V1Container(
name="myapp",
image="myapp:latest"
)
])
)
)
)
apps_api.create_namespaced_deployment(body=deployment, namespace="default")
By using the Kubernetes Python client, we can define and manage Kubernetes resources in code, achieving automated configuration and deployment of infrastructure.
Handling Environment Variables
In actual development and deployment processes, we often need to use different configurations for different environments (such as development, testing, production). Python provides simple ways to read and process environment variables.
import os
db_host = os.getenv('DB_HOST', 'localhost')
db_port = os.getenv('DB_PORT', '5432')
print(f"Connecting to database at {db_host}:{db_port}")
In this way, we can reference environment variables in our code without hardcoding configuration values. This not only improves the portability of the code but also ensures that sensitive information (such as passwords) is not leaked into the codebase.
The Importance of Logging
In a DevOps environment, logging is crucial for monitoring the running status of applications and diagnosing problems. Python has a built-in powerful logging
module that can meet most of our needs.
import logging
logging.basicConfig(level=logging.INFO, format='%(asctime)s - %(levelname)s - %(message)s')
logging.info('Application started')
try:
result = 1 / 0
except ZeroDivisionError:
logging.error('Division by zero', exc_info=True)
By setting different log levels and formats, we can record different levels of information as needed. At the same time, Python's logging also supports advanced features such as writing logs to files and sending them to remote servers, providing us with great flexibility.
API Testing Automation
In modern software development, APIs have become the standard way for applications to communicate with each other. Therefore, ensuring the correctness and reliability of APIs becomes particularly important. Python provides various tools and libraries to help us achieve API testing automation.
import requests
import unittest
class TestAPI(unittest.TestCase):
def test_get_users(self):
response = requests.get('https://api.example.com/users')
self.assertEqual(response.status_code, 200)
self.assertIsInstance(response.json(), list)
def test_create_user(self):
data = {'name': 'John Doe', 'email': '[email protected]'}
response = requests.post('https://api.example.com/users', json=data)
self.assertEqual(response.status_code, 201)
self.assertIn('id', response.json())
if __name__ == '__main__':
unittest.main()
In this example, we used the requests
library to send HTTP requests and the unittest
module to write test cases. By running these tests in the continuous integration/continuous delivery process, we can ensure that the API is thoroughly tested and validated before deployment, thereby improving the quality and reliability of the software.
The Power of Containerization
With the rise of microservice architecture and cloud-native applications, containerization technologies (such as Docker) have become an indispensable part of DevOps practices. Python provides us with an SDK that seamlessly integrates with the Docker engine, allowing us to manage containers and images directly in our code.
import docker
client = docker.from_env()
client.images.build(path='.', tag='myapp')
container = client.containers.run('myapp', detach=True, ports={'8000/tcp': 8000})
print(f"Container started: {container.name}")
By integrating the building, running, and management of containers into automated processes, we can achieve true "infrastructure as code". This not only improves the consistency and repeatability of deployments but also provides us with better portability and scalability.
Summary
Python's applications in the DevOps field are very extensive, from process automation to environment variable handling, from logging to API testing, to the deployment of containerized applications. By mastering Python and its rich libraries and tools, we can greatly improve work efficiency, reduce the risk of human errors, and ensure high-quality software delivery.
So, what are you waiting for? Embrace Python and start your DevOps journey now! Remember, automation is the core of DevOps, and Python is the perfect tool for achieving automation. Let's work together to create more efficient and reliable software delivery processes!
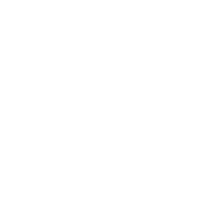