Have you ever written a piece of code and then realized you need to add some extra functionality before and after it, like logging or timing execution? You might think, "Oh no, I have to modify the original code!" Don't worry, Python decorators were created to solve this problem. Today, let's explore the mystery of Python decorators and see how they make our code more elegant and efficient.
What is a Decorator?
As the name suggests, a decorator is a tool used to "decorate" functions or classes. It allows us to add new functionality to functions or classes without modifying the original code. You can think of it like wallpapering a room: you don't change the structure of the room, but it looks refreshed.
In Python, a decorator is essentially a function that takes another function as a parameter and then returns a new function. This new function usually wraps the original function, executing some additional code before and after the original function is called.
Basic Syntax
Let's look at the basic syntax of a decorator:
def my_decorator(func):
def wrapper():
print("Something is happening before the function is called.")
func()
print("Something is happening after the function is called.")
return wrapper
@my_decorator
def say_hello():
print("Hello!")
say_hello()
In this example, my_decorator
is a decorator. We use the @my_decorator
syntax to apply it to the say_hello
function. When we call say_hello()
, we're actually executing the new function wrapped by the decorator.
The output will be:
Something is happening before the function is called.
Hello!
Something is happening after the function is called.
Isn't it amazing? We didn't modify the code of the say_hello
function, yet successfully added new functionality before and after its execution.
Decorators with Parameters
Sometimes, we might need more flexible decorators, such as those that can accept parameters. The implementation is slightly more complex, but the principle is the same. Let's look at an example:
def repeat(times):
def decorator(func):
def wrapper(*args, **kwargs):
for _ in range(times):
result = func(*args, **kwargs)
return result
return wrapper
return decorator
@repeat(3)
def greet(name):
print(f"Hello, {name}!")
greet("Alice")
In this example, we define a repeat
decorator that can accept a parameter specifying the number of times to repeat the execution. When we use @repeat(3)
to decorate the greet
function, the greet
function is executed 3 times.
The output will be:
Hello, Alice!
Hello, Alice!
Hello, Alice!
Real-World Applications
Decorators have many applications in real-world development. Let's look at some common examples:
1. Timer Decorator
If you want to know the execution time of a function, you can use a timer decorator:
import time
def timer(func):
def wrapper(*args, **kwargs):
start_time = time.time()
result = func(*args, **kwargs)
end_time = time.time()
print(f"{func.__name__} took {end_time - start_time:.2f} seconds to execute.")
return result
return wrapper
@timer
def slow_function():
time.sleep(2)
slow_function()
This decorator records the time before and after the function execution, then calculates and prints the execution time.
2. Logging Decorator
Logging is a common requirement, and we can use a decorator to automatically log function call information:
import logging
logging.basicConfig(level=logging.INFO)
def log_function_call(func):
def wrapper(*args, **kwargs):
logging.info(f"Calling {func.__name__}")
result = func(*args, **kwargs)
logging.info(f"{func.__name__} finished")
return result
return wrapper
@log_function_call
def my_function(x, y):
return x + y
result = my_function(3, 4)
print(f"Result: {result}")
This decorator logs information before and after the function call, making it easier to track the program's execution flow.
3. Caching Decorator
For some computationally intensive functions, we can use a caching decorator to store previously computed results, avoiding redundant calculations:
def memoize(func):
cache = {}
def wrapper(*args):
if args in cache:
return cache[args]
result = func(*args)
cache[args] = result
return result
return wrapper
@memoize
def fibonacci(n):
if n < 2:
return n
return fibonacci(n-1) + fibonacci(n-2)
print(fibonacci(100))
This decorator stores the function's arguments and return values in a dictionary. When the function is called again with the same arguments, it directly returns the cached result, significantly improving efficiency.
Considerations
While decorators are powerful, some issues need attention:
-
Function Signature Changes: Decorators might change the original function's signature (i.e., the parameter list and return value type). This can affect the
help()
function or other tools that rely on the function signature. We can use thefunctools.wraps
decorator to preserve the original function's metadata. -
Execution Order: When multiple decorators are applied to the same function, the execution order is from bottom to top. That is, the decorator closest to the function definition executes first.
-
Performance Impact: Although decorators can make code more concise, overuse might affect performance. Each decorator adds a layer of function calls, so be cautious in performance-sensitive scenarios.
Conclusion
Python decorators are a powerful tool that helps us write more concise and maintainable code. By using decorators, we can add new functionality to functions or classes without modifying the original code, such as logging, performance measurement, and access control.
Decorators have a wide range of applications, from simple function wrapping to complex class modification. They can be used not only in daily programming but also in web development, data processing, and other fields.
Do you find decorators interesting? Have you thought about places in your projects where you could use decorators? Feel free to share your thoughts and experiences in the comments. If you have other questions about Python programming or topics you'd like to learn about, please let me know. Let's continue exploring the ocean of Python and discover more interesting knowledge together!
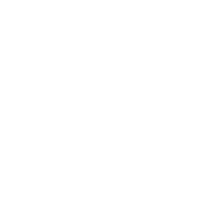
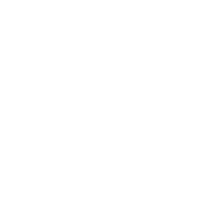