Have you ever felt like there are endless tasks in DevOps? Deployments, configurations, monitoring, log analysis—always overwhelmed? Don't worry, Python is here to save you! Today, let's talk about Python in DevOps and see how it makes tedious operations easy and efficient.
Automation Wizard
Still deploying applications manually? That's outdated! Let's see how Python helps automate deployment:
from fabric import Connection
def deploy_app():
with Connection('user@hostname') as c:
c.run('git pull origin master')
c.run('pip install -r requirements.txt')
c.run('gunicorn app:app')
deploy_app()
See? Just a few lines of code can automatically pull code, install dependencies, and start services. Doesn't it make the whole world feel better?
You might ask, "What's the difference from manual operations?" Huge difference! Imagine you need to deploy to 100 servers. Are you going to log in one by one? With a Python script, it's one-click! Plus, scripts are reusable; just run them next time, saving time and effort.
Configuration Management Master
Managing configuration files can be a headache. Different environments, different services, configurations everywhere. How to manage? Don't worry, Python is here to help:
import configparser
config = configparser.ConfigParser()
config['DATABASE'] = {'host': 'localhost',
'port': '5432',
'user': 'admin',
'password': 'secret'}
with open('config.ini', 'w') as configfile:
config.write(configfile)
This code dynamically generates configuration files. Cool, right? You can generate different configurations for different environments, no more manual modifications. And reading configurations is super easy:
config = configparser.ConfigParser()
config.read('config.ini')
db_host = config['DATABASE']['host']
One line to get configuration info, convenient and fast! Still troubled by managing lots of configuration files? Try Python, making configuration management so simple!
Monitoring and Alerting Helper
System monitoring is crucial in DevOps. Python can help you collect system metrics and alert you in case of anomalies. Check out this example:
import psutil
import smtplib
from email.mime.text import MIMEText
def check_cpu_usage():
cpu_usage = psutil.cpu_percent(interval=1)
if cpu_usage > 90:
send_alert(f"CPU usage is high: {cpu_usage}%")
def send_alert(message):
msg = MIMEText(message)
msg['Subject'] = 'System Alert'
msg['From'] = '[email protected]'
msg['To'] = '[email protected]'
with smtplib.SMTP('smtp.example.com') as server:
server.sendmail(msg['From'], msg['To'], msg.as_string())
check_cpu_usage()
This script checks CPU usage and sends an alert email if it exceeds 90%. You can easily extend this script to monitor memory, disk, network, and other metrics. With this, you can sleep peacefully; Python will wake you up if there's a problem!
Log Analysis Tool
Log files always long and messy? Don't worry, Python's text processing capabilities can handle it easily:
import pandas as pd
logs = pd.read_csv('app.log', sep='\t', header=None, names=['timestamp', 'level', 'message'])
error_logs = logs[logs['level'] == 'ERROR']
hourly_errors = error_logs.groupby(error_logs['timestamp'].dt.hour).size()
print(hourly_errors)
This code quickly analyzes log files, extracts error information, and counts errors per hour. Still manually grepping log files? Try Python, making log analysis so simple!
Perfect Integration with CI/CD
Python scripts can easily integrate into CI/CD processes. For example, you can run Python scripts in Jenkins build steps:
pipeline {
agent any
stages {
stage('Deploy') {
steps {
sh 'python deploy.py'
}
}
}
}
This way, every code submission will have Jenkins automatically run your Python deployment script. Continuous integration and continuous deployment, all in one go!
Container Management
Docker and Kubernetes have become indispensable tools in DevOps. The good news is, Python can perfectly control them:
from docker import DockerClient
client = DockerClient()
containers = client.containers.list()
for container in containers:
print(f"Container {container.name} is running")
client.containers.run("nginx", detach=True)
Using Python's Docker client library, you can easily manage Docker containers. Similarly, Kubernetes also has a Python client library, allowing you to manage the entire cluster with Python code!
Build Your Own DevOps Tools
Existing tools not enough? Don't worry, use Python to build your own DevOps tools! For example, we can develop a simple service health check tool:
import requests
import time
def check_service(url):
try:
response = requests.get(url, timeout=5)
if response.status_code == 200:
print(f"Service at {url} is UP")
else:
print(f"Service at {url} returned status code {response.status_code}")
except requests.RequestException:
print(f"Service at {url} is DOWN")
services = [
"http://example.com",
"http://api.example.com",
"http://admin.example.com"
]
while True:
for service in services:
check_service(service)
time.sleep(60) # Check every minute
This simple script periodically checks the health status of multiple services. You can expand features according to your needs, eventually creating a complete monitoring system!
Summary and Outlook
After reading this, are you eager to use Python to simplify your DevOps work? Python's applications in DevOps are vast, from automated deployment to monitoring and alerting, from log analysis to container management, Python can help greatly.
Moreover, Python's learning curve is relatively smooth, with clear and concise syntax, very suitable for operations personnel to get started. If you haven't started using Python yet, now is the best time!
Do you have any ideas? Want to share your experience using Python in DevOps? Or do you have special needs you want to solve with Python? Feel free to leave a comment and let's explore the infinite possibilities of Python in DevOps together!
Remember, in the world of DevOps, Python is your magic wand—wave it, and problems are solved! Let's use Python to make DevOps work more efficient and fun!
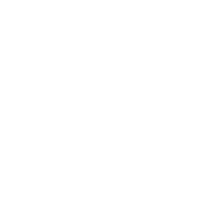
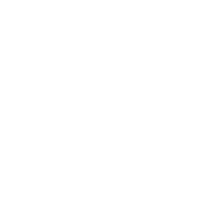
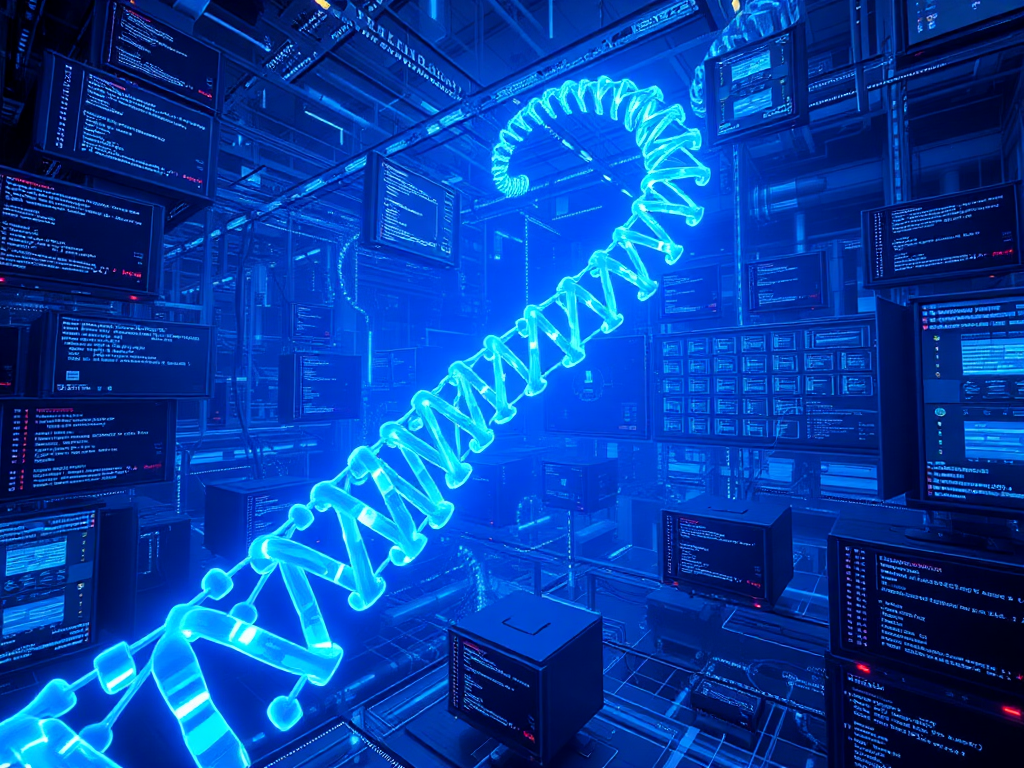
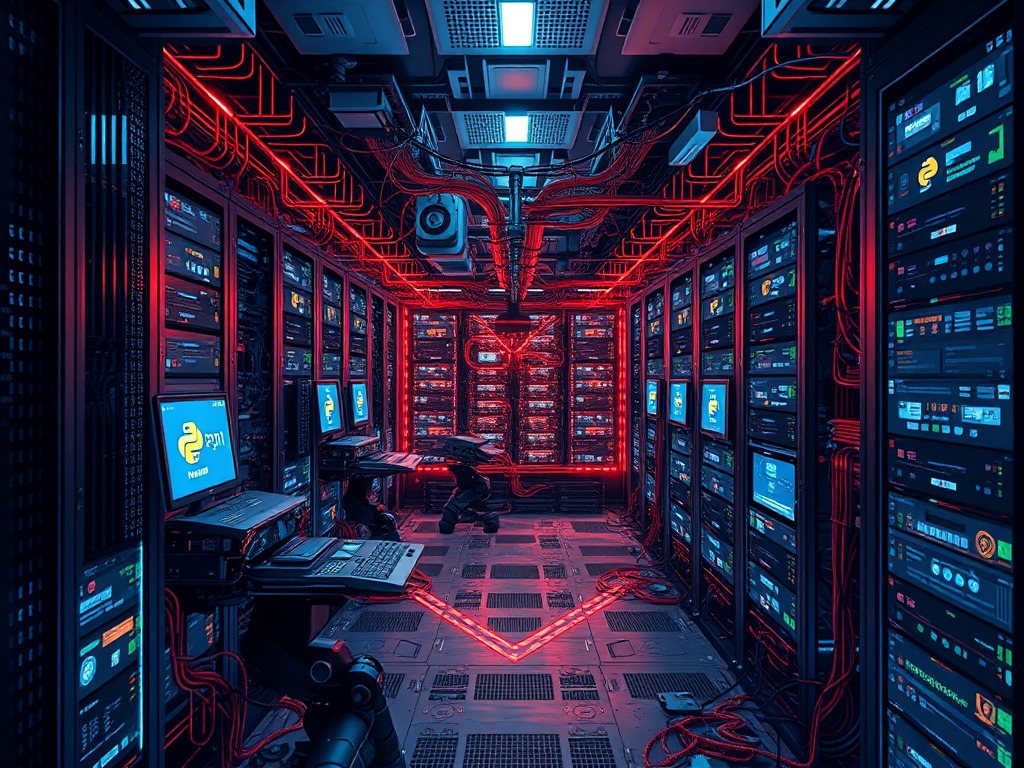
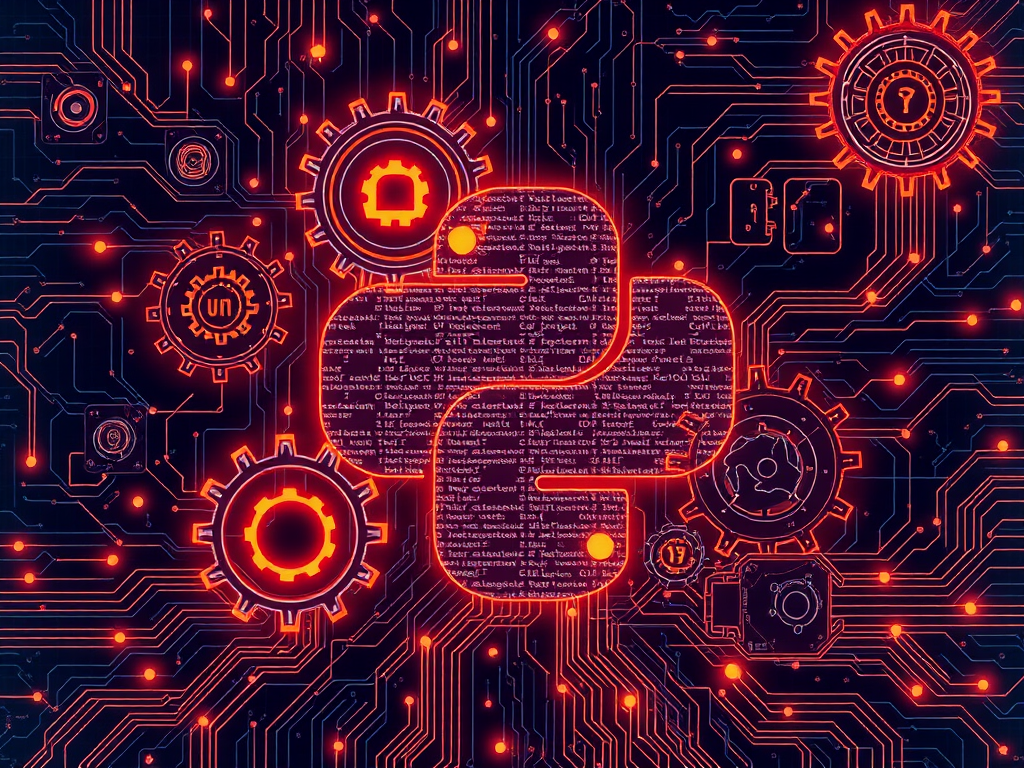