Hey, Python enthusiasts! Last time we talked about how to develop a basic DevOps automation tool with Python. Today, let's go further and explore more possibilities of Python in the DevOps field. From automated testing to container management, to the application of artificial intelligence, Python's potential in DevOps is simply endless! Ready? Let's begin this exciting journey of exploration!
Automated Testing
Did you know? Python can not only help us automate deployment and monitoring but also greatly improve our testing efficiency. Imagine running thousands of test cases automatically after each code submission and knowing if there are any bugs within minutes - isn't that cool?
Let's see how to implement automated testing with Python:
import unittest
from selenium import webdriver
class TestWebApp(unittest.TestCase):
def setUp(self):
self.driver = webdriver.Chrome()
def test_homepage(self):
self.driver.get("http://www.example.com")
self.assertIn("Welcome", self.driver.title)
def test_login(self):
self.driver.get("http://www.example.com/login")
username = self.driver.find_element_by_id("username")
password = self.driver.find_element_by_id("password")
submit = self.driver.find_element_by_id("submit")
username.send_keys("testuser")
password.send_keys("testpass")
submit.click()
self.assertIn("Dashboard", self.driver.title)
def tearDown(self):
self.driver.quit()
if __name__ == "__main__":
unittest.main()
This code uses Python's unittest
framework and Selenium
library to perform automated testing of web applications. It simulates user actions of visiting the homepage and logging in, and verifies if the results meet expectations. You can easily integrate such test scripts into your CI/CD pipeline to run automatically after each code submission.
Container Management
In modern DevOps practices, container technology plays an increasingly important role. Python, with its powerful library support, can easily manage Docker containers. Let's see how to operate Docker with Python:
import docker
client = docker.from_env()
containers = client.containers.list()
for container in containers:
print(f"Container ID: {container.id}, Name: {container.name}")
new_container = client.containers.run("nginx", detach=True)
print(f"Started new container: {new_container.id}")
container_to_stop = client.containers.get("container_name")
container_to_stop.stop()
print(f"Stopped container: {container_to_stop.name}")
client.images.build(path=".", tag="my-image:latest")
print("Built new image: my-image:latest")
This script demonstrates how to use Python's docker
library to manage Docker containers and images. You can list running containers, start new containers, stop containers, and even build new Docker images. These operations can be easily integrated into your DevOps workflow to achieve automated container management.
Infrastructure as Code
Infrastructure as Code (IaC) is an important concept in DevOps. Python can work perfectly with IaC tools like Terraform, allowing you to define and manage infrastructure with code. Take a look at this example:
from python_terraform import *
tf = Terraform()
tf.init()
return_code, stdout, stderr = tf.plan()
print(stdout)
return_code, stdout, stderr = tf.apply(skip_plan=True)
print(stdout)
return_code, stdout, stderr = tf.destroy()
print(stdout)
This script uses the python-terraform
library to call Terraform commands. You can use it to initialize Terraform, plan changes, apply changes, and even destroy resources. This way, you can use Python to control the entire lifecycle of your infrastructure, achieving true "Infrastructure as Code".
Log Aggregation and Analysis
In distributed systems, log management is a huge challenge. But don't worry, Python can help us solve this problem! We can use Python to collect, aggregate, and analyze logs from multiple services. Look at this example:
from elasticsearch import Elasticsearch
import pandas as pd
es = Elasticsearch(['http://localhost:9200'])
result = es.search(index="app-logs", body={
"query": {
"match": {
"level": "ERROR"
}
},
"size": 1000
})
logs = pd.DataFrame([hit['_source'] for hit in result['hits']['hits']])
error_count = logs['message'].value_counts()
print("Top 5 most common errors:")
print(error_count.head())
logs['timestamp'] = pd.to_datetime(logs['timestamp'])
errors_over_time = logs.set_index('timestamp').resample('1H').size()
print("
Errors over time:")
print(errors_over_time)
This script demonstrates how to use Python's elasticsearch
library and pandas
library to collect and analyze logs. It retrieves error logs from Elasticsearch and then uses pandas for data analysis to find the most common errors and error trends over time. This analysis can help you quickly identify and resolve system issues.
Performance Optimization
Python can not only help us monitor system performance but also help us optimize it. Let's see how to perform performance analysis with Python:
import cProfile
import pstats
from io import StringIO
def slow_function():
total = 0
for i in range(1000000):
total += i
return total
pr = cProfile.Profile()
pr.enable()
slow_function()
pr.disable()
s = StringIO()
ps = pstats.Stats(pr, stream=s).sort_stats('cumulative')
ps.print_stats()
print(s.getvalue())
This script uses Python's cProfile
module to analyze function performance. It will tell you the execution time of each line of code in your function, helping you identify performance bottlenecks. With this tool, you can optimize your code targeted and improve system performance.
AI Applications in DevOps
Finally, let's discuss the future of DevOps - the application of artificial intelligence. Python, as the dominant language in AI and machine learning, opens up infinite possibilities for us. Look at this example:
from sklearn.ensemble import IsolationForest
import numpy as np
data = np.random.randn(1000, 5) # 1000 samples, 5 features each
clf = IsolationForest(contamination=0.1, random_state=42)
clf.fit(data)
new_data = np.random.randn(1, 5)
prediction = clf.predict(new_data)
if prediction[0] == -1:
print("Anomaly detected!")
else:
print("System running normally.")
This script uses the Isolation Forest algorithm from the scikit-learn library for anomaly detection. It can learn normal system behavior patterns and then detect abnormal system states. This technique can be used to predict system failures, allowing us to take action before problems occur.
Summary
Wow, we've explored so many applications of Python in DevOps today! From automated testing to container management, from infrastructure as code to log analysis, to performance optimization and AI applications, Python is everywhere. These technologies not only can improve our work efficiency but also help us build more stable and reliable systems.
But this is just the beginning. As technology continues to evolve, there will be more possibilities for Python in DevOps. Perhaps in the future, we'll see more AI-driven DevOps tools or fully automated system operations.
What are your thoughts on Python's applications in DevOps? Are you already using these technologies in your work? Or do you have new ideas you want to try? Feel free to share your experiences and thoughts in the comments. Let's work together to push the development of DevOps with Python and create more possibilities!
Remember, in the world of DevOps, learning never ends. Keep your curiosity and continue exploring, and you'll find that the combination of Python and DevOps always brings surprises. Let's use Python's magic to change the future of DevOps together!
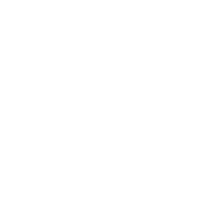
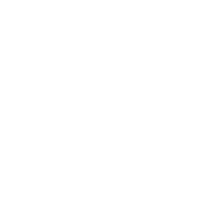