Hey, Python enthusiasts! Today, let's talk about Python's role in the DevOps field. Do you think DevOps is distant from you? Not at all! As a versatile language, Python truly shines in DevOps practices. Let's explore how Python plays a crucial role in automated deployment, configuration management, monitoring, and alerts!
Automated Deployment
When it comes to DevOps, the first thing that comes to mind is automated deployment. That's right, Python has a natural advantage here.
The Tool Fabric
Have you ever faced the situation where every time you deploy a new version, you have to manually SSH into the server and type commands one by one? Annoying, right? Don't worry, Python's Fabric library can solve this problem!
Take a look at this code:
from fabric.api import env, run
env.hosts = ['user@hostname']
def deploy_app():
run('git pull origin master')
run('pip install -r requirements.txt')
run('gunicorn app:app')
deploy_app()
Looks simple, right? But it's quite powerful. This code can help you automatically log into the server, pull the latest code, install dependencies, and then start the application. One-click deployment, that simple!
You might ask, "What's the difference from typing commands manually?" Big difference! Imagine if you have 10 servers to deploy, would you prefer logging into each one and typing commands, or just running this script once to get it all done? The answer is obvious, right?
Ansible Integration
Speaking of automated deployment, we have to mention the powerful tool Ansible. Did you know? Python and Ansible can work perfectly together, making your deployment more flexible and powerful.
For example, you can use Python scripts to dynamically generate Ansible inventory files or write custom Ansible modules. This way, you can adjust your deployment strategy flexibly according to different environments and needs.
Doesn't it feel like Python's application in automated deployment is extensive? That's right, this is just the tip of the iceberg. Next, let's look at how Python performs in configuration management.
Configuration Management
In DevOps practices, configuration management is a very important aspect. Python also performs excellently in this area.
ConfigParser Works Wonders
Still troubled by managing various configuration files? Check out how Python's ConfigParser library easily solves this problem:
import configparser
config = configparser.ConfigParser()
config.read('config.ini')
database_url = config.get('database', 'url')
This code looks simple, but its functionality is not. It can easily read INI format configuration files, allowing your application to load different configurations according to different environments.
You might ask, "Why use ConfigParser? Can't I just hard code it?" Of course, but imagine if your application needs to be deployed in multiple environments like development, testing, and production, each with different configurations. Would you rather change the code each time or just modify a configuration file?
Dynamically Generating Configurations
Besides reading configurations, Python can also dynamically generate configuration files. Imagine you have a large distributed system, and each node's configuration is slightly different. Would you prefer writing configuration files manually for each node or using a Python script to generate them automatically?
import configparser
def generate_config(node_id, ip_address):
config = configparser.ConfigParser()
config['NODE'] = {
'id': node_id,
'ip': ip_address
}
with open(f'node_{node_id}_config.ini', 'w') as configfile:
config.write(configfile)
for i in range(100):
generate_config(i, f'192.168.1.{i}')
See, with just a few lines of code, we can generate unique configuration files for 100 nodes. This is Python's strength in configuration management!
After talking about configuration management, do you feel that Python's application in DevOps is already extensive? Hold on, the best is yet to come. Next, let's see how Python performs in monitoring and alerts.
Monitoring and Alerts
In DevOps practices, monitoring and alerts are key to ensuring system stability. Python also performs excellently in this area.
psutil: A System Monitoring Tool
Still worried about how to monitor system performance? Check out how Python's psutil library easily solves this problem:
import psutil
import smtplib
from email.mime.text import MIMEText
cpu_usage = psutil.cpu_percent(interval=1)
if cpu_usage > 90:
msg = MIMEText(f"CPU usage is high: {cpu_usage}%")
msg['Subject'] = 'High CPU Usage Alert'
msg['From'] = '[email protected]'
msg['To'] = '[email protected]'
server = smtplib.SMTP('smtp.example.com')
server.sendmail(msg['From'], msg['To'], msg.as_string())
server.quit()
This code looks a bit complex, but its functionality is very powerful. It can monitor CPU usage and automatically send an alert email when usage exceeds 90%.
You might ask, "Why use Python for this? Aren't there many ready-made monitoring tools on the market?" True, but Python's flexibility allows you to customize monitoring strategies according to your needs. For example, you can easily modify this code to monitor CPU, memory, disk usage, or even specific metrics of your application.
Log Analysis
Besides system monitoring, log analysis is also a crucial part of DevOps. Python's text processing capabilities can shine here. See how to use the pandas library to analyze log files:
import pandas as pd
logs = pd.read_csv('app.log', sep='\t', header=None, names=['timestamp', 'message'])
error_logs = logs[logs['message'].str.contains('ERROR')]
This code can easily read and analyze log files, allowing you to quickly identify issues in the system. Imagine if your application generates several gigabytes of logs daily, would you prefer manually finding problems or using such scripts to analyze them automatically?
Python's application in monitoring and alerting is not limited to this. You can also use it to build complex monitoring systems, achieving automated problem diagnosis and resolution. Does it feel like Python's application in DevOps is becoming more extensive?
CI/CD Integration
Speaking of DevOps, we must mention Continuous Integration and Continuous Deployment (CI/CD). Did you know? Python also plays an important role in the CI/CD process.
Jenkins with Python Wings
Jenkins is a widely used CI/CD tool, and Python can make it even more powerful. Imagine, you can use Python scripts to customize your build process, automate your tests, and even dynamically generate your deployment configurations.
pipeline {
agent any
stages {
stage('Build') {
steps {
sh 'python build.py'
}
}
stage('Test') {
steps {
sh 'python run_tests.py'
}
}
stage('Deploy') {
steps {
sh 'python deploy.py'
}
}
}
}
See, with just a few lines of code, we can integrate Python scripts into Jenkins to achieve fully automated build, test, and deployment processes. Are you still manually managing your CI/CD process?
Python Magic in GitLab CI/CD
It's not just Jenkins; Python can also work its magic in GitLab CI/CD. You can use Python scripts to automate your code review process, generate test reports, and even automatically release versions.
stages:
- test
- deploy
test:
stage: test
script:
- python run_tests.py
deploy:
stage: deploy
script:
- python deploy.py
only:
- master
This configuration file looks simple, but it can make your GitLab project automatically run tests and deploy to the production environment once the tests pass. Imagine, you'll never have to worry about forgetting to run tests or deploy the latest version again—everything is automatic!
Python's application in CI/CD is not limited to this. You can also use it to perform automated performance tests, security scans, and even automatically generate change logs. Does it feel like Python makes your CI/CD process smarter and more efficient?
Cloud-Native Applications
In this era of cloud computing, Python plays an important role in cloud-native application development. Let's see how Python shines in microservices architecture and containerized applications.
Python in Microservices Architecture
Microservices architecture has become mainstream for cloud-native applications, and Python's lightweight and efficiency make it an ideal choice for developing microservices. Imagine, you can quickly build RESTful APIs with Flask or FastAPI, achieve efficient service communication with gRPC, and handle asynchronous tasks with Celery.
from fastapi import FastAPI
app = FastAPI()
@app.get("/")
async def root():
return {"message": "Hello World"}
@app.get("/items/{item_id}")
async def read_item(item_id: int):
return {"item_id": item_id}
See, with just a few lines of code, we've created a simple microservice. You can easily package this service into a Docker container and deploy it to a Kubernetes cluster. Doesn't it feel like Python makes microservice development so easy?
Python Magic in Containerization
Speaking of cloud-native applications, we must mention containerization. Did you know? The combination of Python and Docker is a match made in heaven. You can easily package Python applications into Docker images, achieving true "build once, run anywhere."
FROM python:3.9-slim
WORKDIR /app
COPY requirements.txt .
RUN pip install --no-cache-dir -r requirements.txt
COPY . .
CMD ["python", "app.py"]
This Dockerfile looks simple, but it allows your Python application to run in any Docker-supported environment. Imagine, you'll never have to worry about "it works on my machine" issues again!
Python's application in cloud-native scenarios is not limited to this. You can also use it to write Kubernetes operators, achieving custom application deployment and management logic. Does it feel like Python makes your cloud-native journey smoother?
Conclusion
In this article, we explored various applications of Python in DevOps, from automated deployment to configuration management, from monitoring and alerts to CI/CD integration, and finally to cloud-native application development. Do you feel that Python's application in DevOps is so extensive and powerful?
Actually, this is just the tip of the iceberg. Python's flexibility and powerful ecosystem give it unlimited potential in the DevOps field. Whether writing automation scripts or building complex DevOps tools, Python can handle it.
So, are you ready to use Python in your DevOps practices? Remember, DevOps is not just a set of tools or processes but a culture and mindset. And Python is a powerful assistant for realizing this culture and mindset.
Let's use Python to make DevOps smarter, more efficient, and more fun! Do you have any thoughts or experiences to share? Feel free to leave a comment and let's discuss the endless possibilities of Python in DevOps!
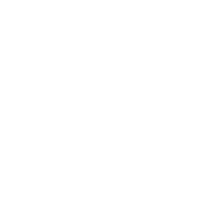
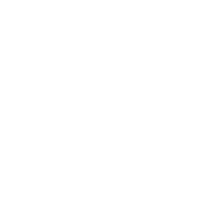