Have you ever been troubled by complex text processing problems? Or felt overwhelmed when dealing with large amounts of data? Don't worry, Python regular expressions are here to save the day. As a powerful text processing tool, regular expressions can help you easily handle various text matching, extraction, and replacement tasks. Today, let's delve into the mysteries of Python regular expressions, from basic concepts to advanced techniques, to comprehensively improve your text processing skills.
Getting Started with Regular Expressions
Do you remember how you felt when you first encountered regular expressions? I guess, like me, most people would feel confused when seeing those strange symbol combinations. But don't worry, let's start with the most basic concepts and gradually unveil the mystery of regular expressions.
First, what are regular expressions? Simply put, they are powerful tools for matching string patterns. Imagine you have a large amount of text and need to find all the phone numbers. If you search manually, it would be a huge task. But with regular expressions, you can easily complete this task by writing a simple pattern.
In Python, we mainly use regular expressions through the re
module. Let's look at a simple example:
import re
text = "My phone number is 123-4567-8900, my friend's phone number is 987-6543-2100"
pattern = r'\d{3}-\d{4}-\d{4}'
matches = re.findall(pattern, text)
print(matches)
When you run this code, you'll get:
['123-4567-8900', '987-6543-2100']
See? We successfully extracted all the phone numbers with a simple pattern \d{3}-\d{4}-\d{4}
. Isn't it amazing?
The Magic of Metacharacters
The power of regular expressions lies in their metacharacters. These special characters give regular expressions the ability to match various complex patterns. Let's look at some commonly used metacharacters:
.
: Matches any character except a newline^
: Matches the start of the string$
: Matches the end of the string*
: Matches the previous pattern zero or more times+
: Matches the previous pattern one or more times?
: Matches the previous pattern zero or one time\d
: Matches any digit\w
: Matches any letter, digit, or underscore\s
: Matches any whitespace character
These metacharacters may look simple, but when combined, they can exert powerful capabilities. For example, we can use ^\w+@\w+\.\w+$
to match a simple email address.
import re
email = "[email protected]"
pattern = r'^\w+@\w+\.\w+$'
if re.match(pattern, email):
print("Valid email address")
else:
print("Invalid email address")
In this example, ^\w+
matches the username part at the beginning of the email address, @
matches the @ symbol, \w+\.
matches the domain part, and the final \w+$
matches the top-level domain.
Group Capture
Another powerful feature of regular expressions is group capture. By using parentheses in the pattern, we can group matched parts and use these groups separately in subsequent processing.
Let's look at an example:
import re
text = "My birthday is 1990-12-31"
pattern = r'(\d{4})-(\d{1,2})-(\d{1,2})'
match = re.search(pattern, text)
if match:
year, month, day = match.groups()
print(f"Year: {year}, Month: {month}, Day: {day}")
In this example, we used three groups to capture the year, month, and day separately. When you run this code, you'll see:
Year: 1990, Month: 12, Day: 31
Isn't it amazing? We not only matched the date but also successfully extracted the year, month, and day separately.
Greedy vs Non-Greedy
When using regular expressions, you might encounter an interesting phenomenon: sometimes the match results are more than you expected. This involves the greedy matching characteristic of regular expressions.
By default, regular expression matching is greedy, meaning it will match as many characters as possible. But sometimes, we need the minimum match. In this case, we can use non-greedy matching.
Let's understand this concept through an example:
import re
text = "<div>Hello, World!</div><div>Python is awesome!</div>"
greedy_pattern = r'<div>.*</div>'
greedy_match = re.findall(greedy_pattern, text)
print("Greedy match result:", greedy_match)
non_greedy_pattern = r'<div>.*?</div>'
non_greedy_match = re.findall(non_greedy_pattern, text)
print("Non-greedy match result:", non_greedy_match)
When you run this code, you'll see:
Greedy match result: ['<div>Hello, World!</div><div>Python is awesome!</div>']
Non-greedy match result: ['<div>Hello, World!</div>', '<div>Python is awesome!</div>']
Do you see the difference? Greedy matching will match as many characters as possible, resulting in only one result being returned. Non-greedy matching (achieved by adding ?
after *
) will match as few characters as possible, thus correctly matching the contents of the two div tags separately.
Advanced Techniques
After mastering the basics, let's look at some advanced techniques that can help you use regular expressions more efficiently.
1. Using re.compile() to Improve Efficiency
If you need to use the same regular expression multiple times, using re.compile()
can significantly improve efficiency. This function compiles the regular expression into an object, so you don't need to recompile it every time you use it.
import re
pattern = re.compile(r'\d+')
text1 = "I have 3 apples and 5 oranges"
text2 = "There are 10 cats and 15 dogs"
print(pattern.findall(text1))
print(pattern.findall(text2))
2. Using Named Groups
In addition to using numeric indices to reference groups, we can also name groups, which can make our code clearer and easier to read.
import re
text = "My phone number is 123-4567-8900"
pattern = r'(?P<area>\d{3})-(?P<middle>\d{4})-(?P<last>\d{4})'
match = re.search(pattern, text)
if match:
print(f"Area code: {match.group('area')}")
print(f"Middle four digits: {match.group('middle')}")
print(f"Last four digits: {match.group('last')}")
3. Using Assertions
Positive and negative assertions are advanced features in regular expressions that can help you control matching more precisely.
import re
text = "I love python and javascript"
pattern1 = r'python(?= and)'
print(re.search(pattern1, text).group()) # Output: python
pattern2 = r'python(?!script)'
print(re.search(pattern2, text).group()) # Output: python
pattern3 = r'(?<=java)script'
print(re.search(pattern3, text).group()) # Output: script
pattern4 = r'(?<!python)script'
print(re.search(pattern4, text).group()) # Output: script
These advanced techniques might look a bit complex, but once you master them, you'll be able to write more precise and efficient regular expressions.
Practical Application
Now that we've learned so much theoretical knowledge, let's see how to apply regular expressions in actual projects. Let's solve a common problem: extracting all URLs from an article.
import re
text = """
Welcome to visit my blog https://www.myblog.com .
You can also follow me on GitHub (https://github.com/myaccount) .
If you have any questions, you can email me at [email protected] .
"""
url_pattern = r'https?://[^\s]+'
email_pattern = r'\b[A-Za-z0-9._%+-]+@[A-Za-z0-9.-]+\.[A-Z|a-z]{2,}\b'
urls = re.findall(url_pattern, text)
emails = re.findall(email_pattern, text)
print("Extracted URLs:")
for url in urls:
print(url)
print("
Extracted emails:")
for email in emails:
print(email)
When you run this code, you'll get:
Extracted URLs:
https://www.myblog.com
https://github.com/myaccount
Extracted emails:
myemail@example.com
See? We successfully extracted all URLs and email addresses with just a few lines of code. This is particularly useful when dealing with large amounts of text data.
Summary and Outlook
Through this article, we started from the basic concepts of regular expressions and gradually delved into some advanced techniques and practical applications. Regular expressions are indeed a powerful tool that can help us solve various complex text processing problems.
However, regular expressions also have their limitations. For some more complex text analysis tasks, we might need to combine other natural language processing techniques. For example, if you need to understand the semantics of the text, not just match patterns, you might need to consider using natural language processing libraries like NLTK or spaCy.
Finally, I want to say that regular expressions are like a Swiss Army knife - small but powerful, but not the best solution for all problems. When using regular expressions, we need to balance efficiency and readability. Sometimes, using ordinary string methods might be simpler and more direct. Choosing the right tool to solve the problem is the real programming wisdom.
So, are you ready to apply regular expressions in your next project? Remember, practice makes perfect. Practice more, think more, and you will surely become an expert in regular expressions. I wish you smooth sailing on your Python programming journey.
Do you have any questions about regular expressions? Or do you have any unique experiences using regular expressions that you'd like to share? Feel free to leave a comment, let's discuss and progress together.
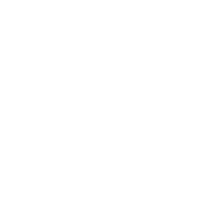
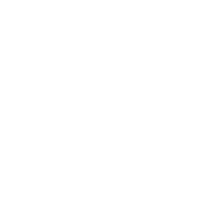
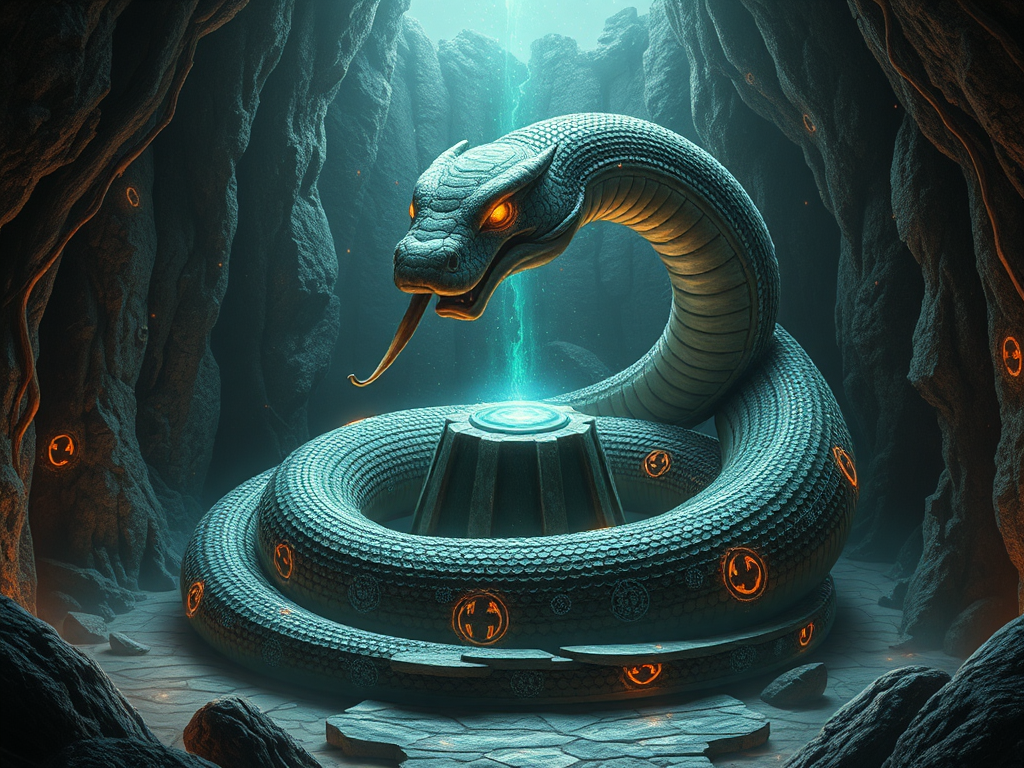
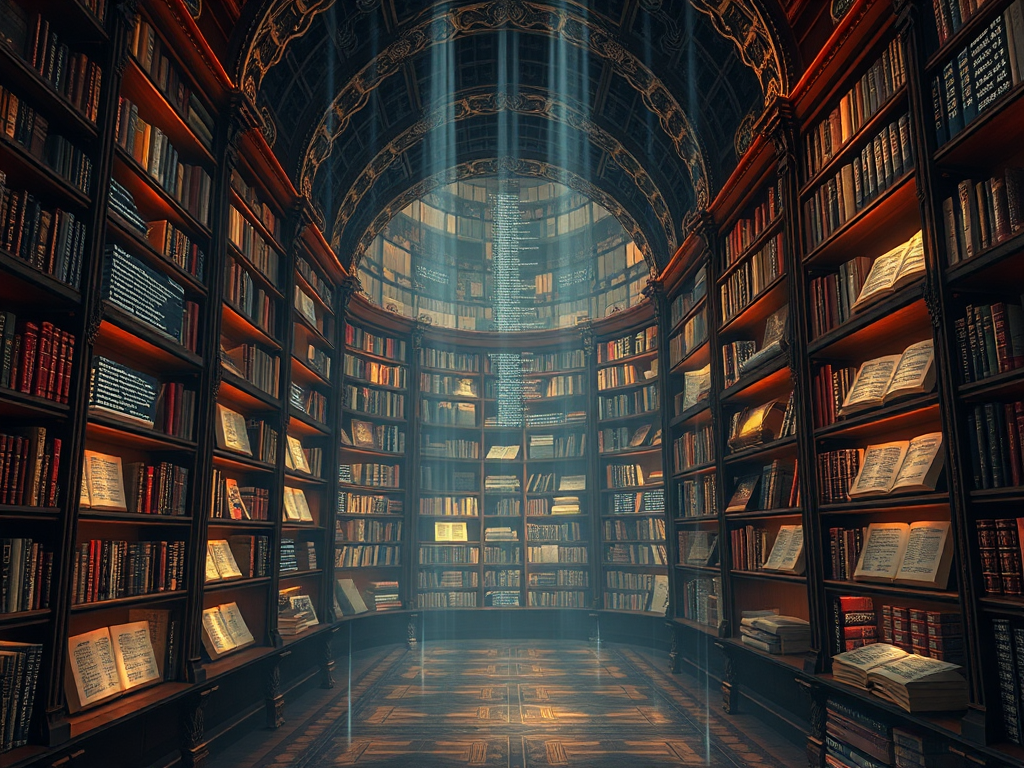
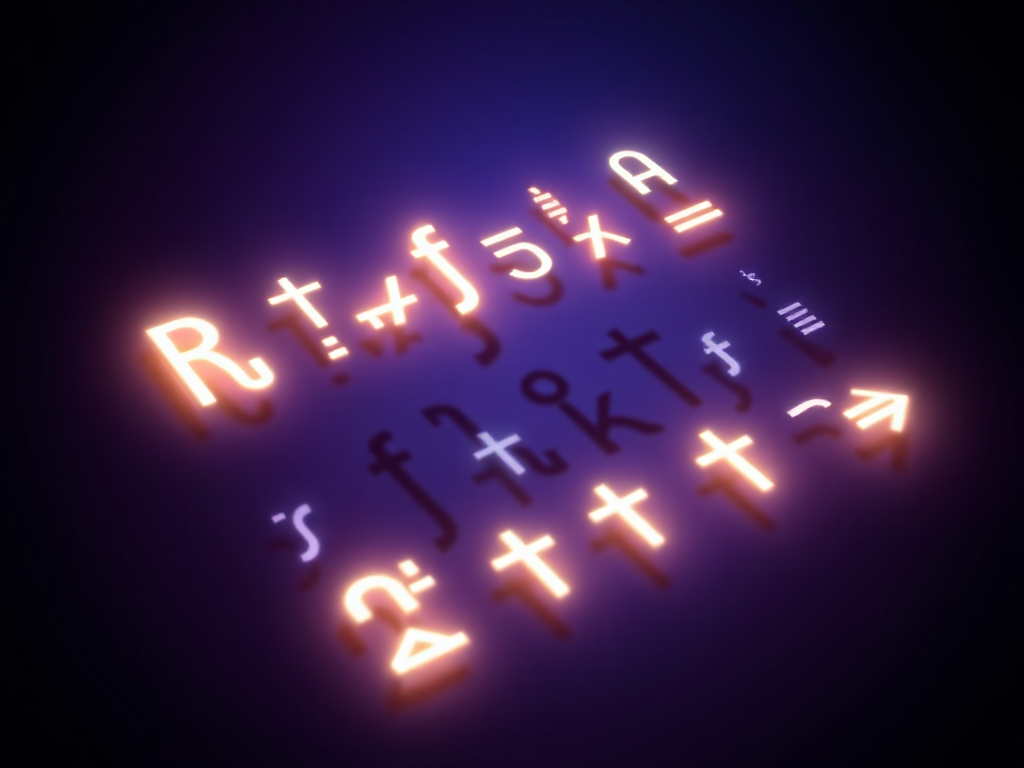