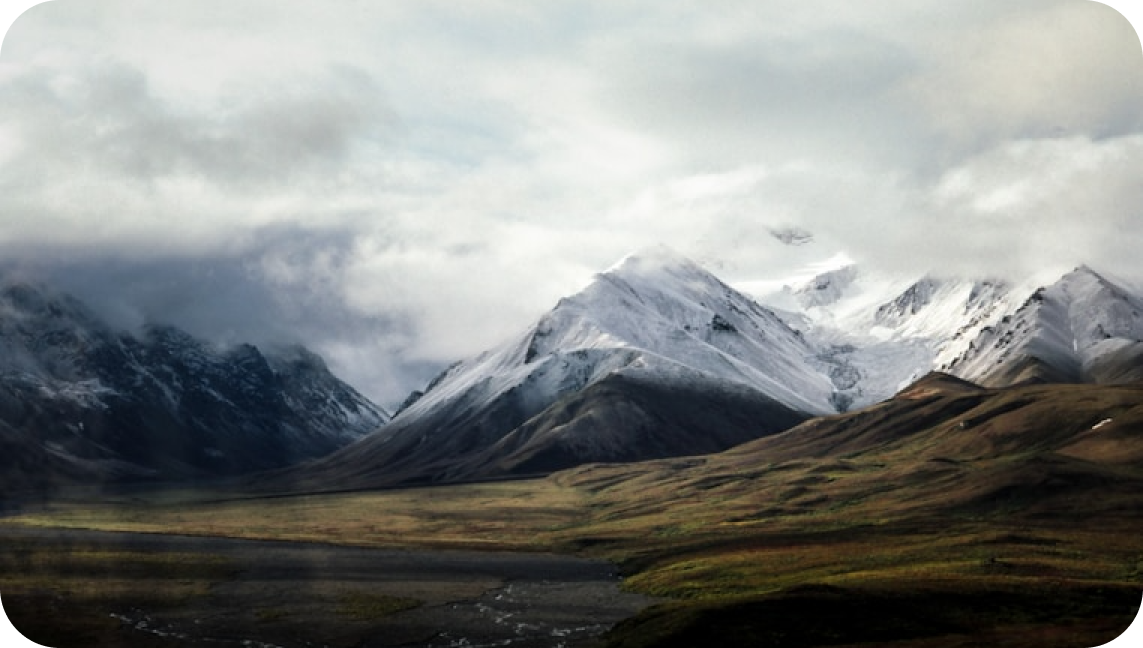
Introduction
Have you ever been frustrated with processing text data? When I first started learning Python, I often needed to extract email addresses from text, parse log files, and validate user input. Using regular string processing methods for these tasks not only resulted in verbose code but was also error-prone. Then I discovered regular expressions - they were like a Swiss Army knife that helped me easily handle these tricky problems.
Today, I'd like to share my experience with regular expressions. Have you had similar experiences? Let's dive deep into this powerful text processing tool.
Basics
Before we start, let's understand the basic concepts of regular expressions. Regular expressions are essentially special string matching patterns that use special characters to express complex search rules.
Python provides a dedicated re module to support regular expression operations. To use regular expressions, you first need to import this module:
import re
The most basic application of regular expressions is pattern matching. For example, if we want to find all words in a text, we can do this:
text = "Hello 123 World"
pattern = r"\w+"
matches = re.findall(pattern, text)
print(matches) # ['Hello', '123', 'World']
Do you see that? Text tokenization was accomplished in just a few lines of code. What does "\w+" mean here? "\w" matches any letter, number, or underscore, and "+" matches one or more of the preceding character. That's the magic of regular expressions.
Practical Applications
In my work, I often need to handle data in various formats. Let me share some practical examples - you'll definitely encounter similar scenarios.
Data Cleaning
Suppose you're processing a text file containing user information and need to extract all email addresses:
text = """
Contact information:
Zhang San [email protected]
Li Si [email protected]
Wang Wu [email protected]
"""
email_pattern = r'[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}'
emails = re.findall(email_pattern, text)
print(emails)
This pattern looks complicated, right? Let's break it down: - [a-zA-Z0-9._%+-]+ matches the email name part - @ matches the @ symbol - [a-zA-Z0-9.-]+ matches the domain part - . matches the dot - [a-zA-Z]{2,} matches the top-level domain
Data Validation
When developing websites, we often need to validate user input. For example, validating phone numbers:
def is_valid_phone(phone):
pattern = r'^1[3-9]\d{9}$'
return bool(re.match(pattern, phone))
test_phones = ["13812345678", "1381234567", "23812345678"]
for phone in test_phones:
print(f"{phone}: {'valid' if is_valid_phone(phone) else 'invalid'}")
This pattern means: - ^ indicates the start - 1 means the first digit must be 1 - [3-9] means the second digit must be between 3-9 - \d{9} means there must be 9 digits afterward - $ indicates the end
Advanced Topics
As I gained experience, I discovered many advanced uses of regular expressions. These techniques can make our code more flexible and powerful.
Group Capture
Grouping is one of the most powerful features in regular expressions. Using parentheses (), we can save matched content in groups:
log_line = "2024-03-15 10:30:45 [ERROR] Failed to connect to database"
pattern = r'(\d{4}-\d{2}-\d{2}) (\d{2}:\d{2}:\d{2}) \[(.*?)\] (.*)'
match = re.match(pattern, log_line)
if match:
date, time, level, message = match.groups()
print(f"Date: {date}")
print(f"Time: {time}")
print(f"Level: {level}")
print(f"Message: {message}")
Greedy vs Non-Greedy Matching
This is an issue I encounter frequently. Look at this example:
text = "<div>First Part</div><div>Second Part</div>"
print(re.findall(r'<div>.*</div>', text))
print(re.findall(r'<div>.*?</div>', text))
By default, regular expressions use greedy matching, which matches as many characters as possible. Adding a question mark ? makes it non-greedy mode, which matches as few characters as possible.
Lookahead and Lookbehind Assertions
This is a more advanced feature but very useful in certain scenarios:
text = "¥100 $200 €300"
print(re.findall(r'[¥$€](?=\d+)', text)) # ['¥', '$', '€']
print(re.findall(r'(?<=[¥$€])\d+', text)) # ['100', '200', '300']
Optimization
When using regular expressions, performance is also an important factor to consider. Here are some optimization tips I've summarized:
- Use re.compile() to precompile frequently used regular expressions:
email_pattern = re.compile(r'[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}')
for line in large_file:
matches = email_pattern.findall(line)
- Avoid overly complex regular expressions. Sometimes, breaking down a complex pattern into multiple simple patterns is better:
complex_pattern = r'^(?=.*[A-Z])(?=.*[a-z])(?=.*\d)(?=.*[@$!%*?&])[A-Za-z\d@$!%*?&]{8,}$'
def is_strong_password(password):
patterns = [
r'[A-Z]', # At least one uppercase letter
r'[a-z]', # At least one lowercase letter
r'\d', # At least one digit
r'[@$!%*?&]', # At least one special character
]
return all(re.search(p, password) for p in patterns) and len(password) >= 8
Conclusion
Regular expressions are like a mini programming language - mastering them takes time and practice. But once you become proficient, they become a powerful ally in handling text data.
I'm curious about how you use regular expressions. Have you encountered any interesting applications? Feel free to share your experiences and thoughts in the comments. Let's explore this powerful tool together and solve more practical problems.
By the way, I suggest you bookmark this article for future reference. Whenever you encounter text processing needs, come back and take a look - you might find inspiration for solving your problems.
Next
Introduction to Python Regular Expressions: Master Essential Text Processing Skills from Scratch
A comprehensive guide to Python regular expressions, covering fundamental concepts, special characters, re module functionality, and practical text processing examples for efficient pattern matching and manipulation
Python Regular Expressions: Mastering the Art of Text Processing from Scratch
A comprehensive guide to regular expressions in Python, covering basic concepts, core features of the re module, special characters usage, and practical email matching examples
Python Regular Expressions: A Practical Guide from Beginner to Master
A comprehensive guide to Python regular expressions, covering basic concepts, re module usage, metacharacters, common functions, and practical examples including email matching and text replacement
Next
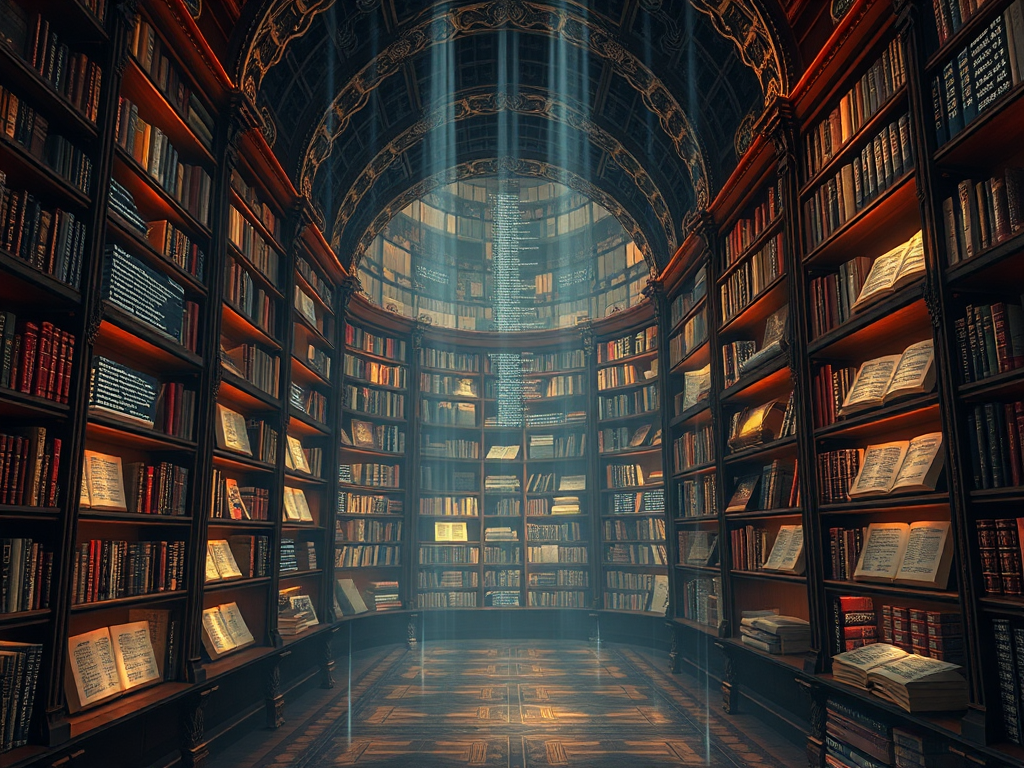
Introduction to Python Regular Expressions: Master Essential Text Processing Skills from Scratch
A comprehensive guide to Python regular expressions, covering fundamental concepts, special characters, re module functionality, and practical text processing examples for efficient pattern matching and manipulation
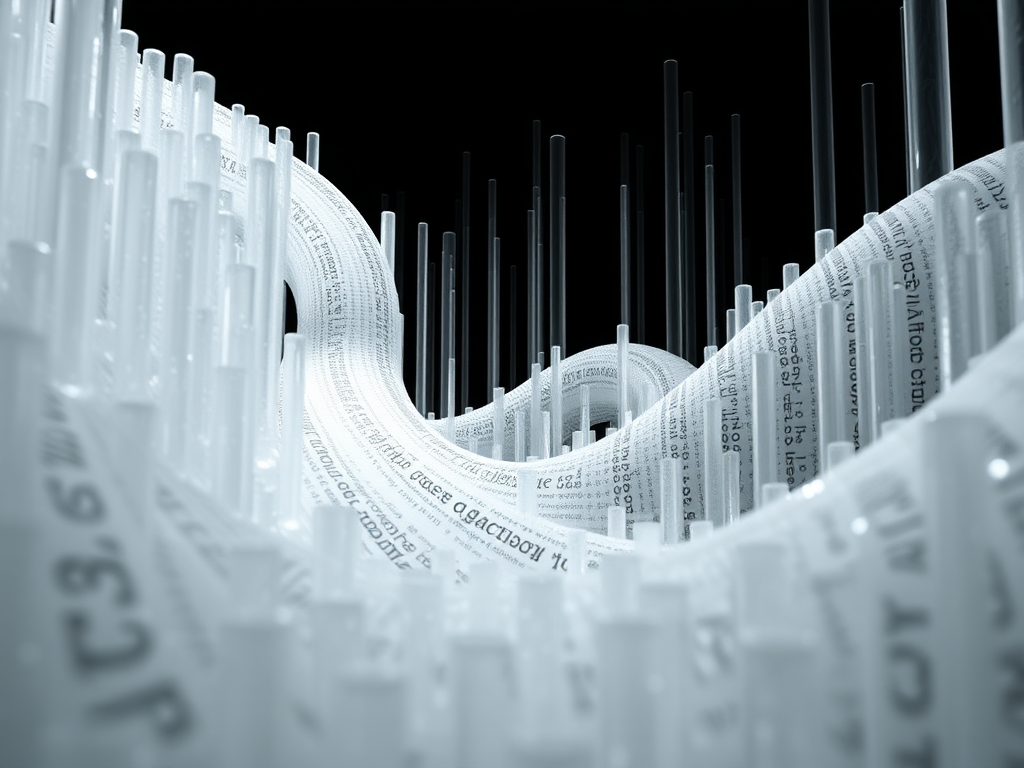
Python Regular Expressions: Mastering the Art of Text Processing from Scratch
A comprehensive guide to regular expressions in Python, covering basic concepts, core features of the re module, special characters usage, and practical email matching examples
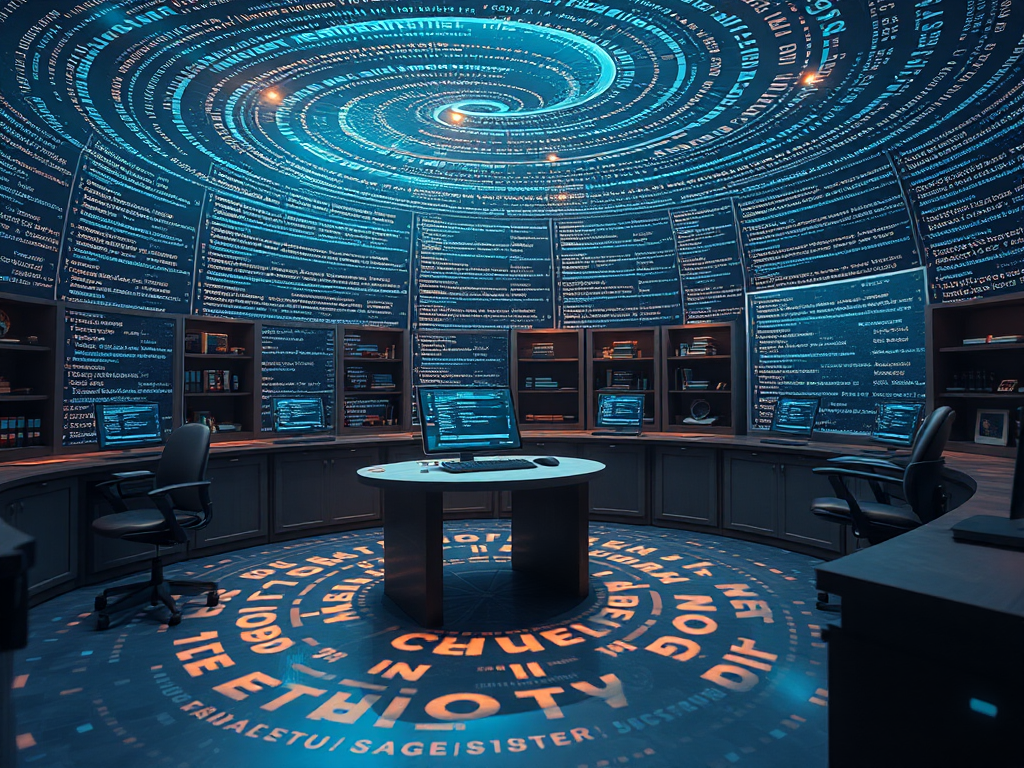
Python Regular Expressions: A Practical Guide from Beginner to Master
A comprehensive guide to Python regular expressions, covering basic concepts, re module usage, metacharacters, common functions, and practical examples including email matching and text replacement