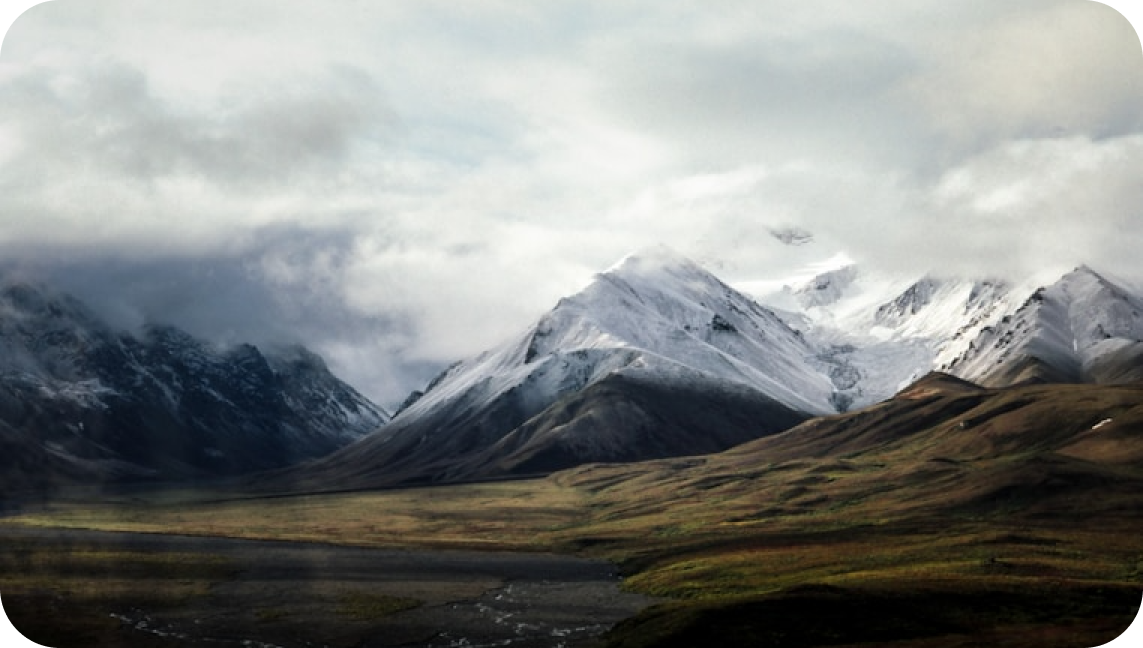
Origins
Do you often need to process large amounts of text data? Do you find yourself struggling when extracting, searching, or replacing specific text patterns? Today, let's dive deep into Python regular expressions, a powerful text processing tool. As a Python developer, I deeply understand the importance of regular expressions in text processing. Years of development experience have taught me: mastering regular expressions is like having a Swiss Army knife in the field of text processing.
Basics
Before diving deeper, let's understand what regular expressions are. Simply put, a regular expression is a text matching pattern represented by special symbols. You can think of it as a "universal text searcher" that finds and matches text meeting specific conditions through defined rules.
Here's a real-life example: Suppose you have a pile of delivery tracking numbers to organize, from different courier companies like SF Express, JD, and YTO. This is where regular expressions come in handy. For instance, SF Express tracking numbers all start with "SF" followed by 12 characters. We can match these using the following code:
import re
text = """
SF1234567890
JD987654321
YT5678901234
SF9876543210
"""
sf_pattern = r'SF\d{10}'
sf_numbers = re.findall(sf_pattern, text)
print("SF Express tracking numbers:", sf_numbers)
Want to understand how this code works? Let's break it down:
r'SF'
matches the letters "SF"\d{10}
matches 10 digits- The
findall()
function returns all matches
Advanced
At this point, you might ask: regular expressions seem powerful, but those special symbols look overwhelming - how do you memorize them? Actually, we can understand regex syntax by breaking it down into core concepts.
Character Classes
Character classes are like sets of characters, represented by square brackets []
. For example:
- [abc]
matches any single character a, b, or c
- [0-9]
matches any single digit
- [a-zA-Z]
matches any single English letter
Let's look at a practical example, suppose we want to extract all Chinese mobile phone numbers from text:
text = """
Contact: 13812345678
Customer Service: +86 17698765432
Landline: 010-12345678
Mobile: 19987654321
"""
phone_pattern = r'1[3-9]\d{9}'
phone_numbers = re.findall(phone_pattern, text)
print("Phone numbers:", phone_numbers)
This pattern means: 1. Starts with 1 2. Second digit is any number from 3-9 3. Followed by 9 digits
Practical Applications
The real power of regular expressions lies in their practical applications. Let's look at some common scenarios in actual development:
Data Cleaning
Suppose you've scraped some product price data from websites, but the format isn't consistent:
price_text = """
Product A: ¥99.9
Product B: 99.90元
Product C: RMB 99.9
Product D: 99.9
"""
price_pattern = r'\d+\.?\d*'
prices = re.findall(price_pattern, price_text)
print("Extracted prices:", prices)
formatted_prices = [f"¥{float(price):.2f}" for price in prices]
print("Formatted prices:", formatted_prices)
Email Validation
Email validation is a common requirement in web application development:
def is_valid_email(email):
pattern = r'^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}$'
return bool(re.match(pattern, email))
test_emails = [
"[email protected]",
"invalid.email@com",
"[email protected]",
"@invalid.com"
]
for email in test_emails:
print(f"{email}: {'valid' if is_valid_email(email) else 'invalid'}")
Performance
When discussing regular expressions, we must address an important topic: performance optimization. Regular expression performance is crucial when processing large amounts of text data.
Pattern Precompilation
If you need to use the same regular expression multiple times, it's recommended to use precompiled patterns:
def find_emails_slow(text):
return re.findall(r'\b[\w\.-]+@[\w\.-]+\.\w+\b', text)
email_pattern = re.compile(r'\b[\w\.-]+@[\w\.-]+\.\w+\b')
def find_emails_fast(text):
return email_pattern.findall(text)
import time
text = "[email protected] [email protected] " * 10000
start_time = time.time()
find_emails_slow(text)
print(f"Without compilation: {time.time() - start_time:.4f} seconds")
start_time = time.time()
find_emails_fast(text)
print(f"With precompilation: {time.time() - start_time:.4f} seconds")
Greedy vs Non-Greedy Matching
Regular expressions use greedy matching by default, but sometimes this isn't what we want:
text = "<div>First part</div><div>Second part</div>"
greedy_pattern = r'<div>.*</div>'
print("Greedy match:", re.findall(greedy_pattern, text))
non_greedy_pattern = r'<div>.*?</div>'
print("Non-greedy match:", re.findall(non_greedy_pattern, text))
Practical Tips
From years of Python development experience, I've summarized some practical tips for using regular expressions:
-
Break Down Complex Problems When facing complex text processing needs, don't try to write the perfect regex at once. Breaking the problem into smaller steps and improving gradually is easier.
-
Use Online Tools Recommend using online regex testing tools to see matching results in real-time, speeding up the debugging process.
-
Comments Are Important Add comments to complex regular expressions explaining what each part does:
pattern = re.compile(
r'''
^ # Start
[a-zA-Z0-9._%+-]+ # Username part
@ # @ symbol
[a-zA-Z0-9.-]+ # Domain name part
\.[a-zA-Z]{2,} # Top-level domain
$ # End
''', re.VERBOSE)
Conclusion
Regular expressions are like a mini programming language; mastering them takes time and practice. But once mastered, they become your powerful ally in text data processing. What do you find most difficult to understand about regular expressions? Feel free to share your experiences and questions in the comments.
Remember, the best way to learn regular expressions is through practice. Start with simple patterns and gradually try more complex applications. Through continuous practice, you'll surely become proficient with this powerful tool.
So, are you ready to begin your regular expression journey? Let's improve our text processing skills together through practice.
Next
Introduction to Python Regular Expressions: Master Essential Text Processing Skills from Scratch
A comprehensive guide to Python regular expressions, covering fundamental concepts, special characters, re module functionality, and practical text processing examples for efficient pattern matching and manipulation
Python Regular Expressions: Mastering the Art of Text Processing from Scratch
A comprehensive guide to regular expressions in Python, covering basic concepts, core features of the re module, special characters usage, and practical email matching examples
Python Regular Expressions: A Practical Guide from Beginner to Master
A comprehensive guide to Python regular expressions, covering basic concepts, re module usage, metacharacters, common functions, and practical examples including email matching and text replacement
Next
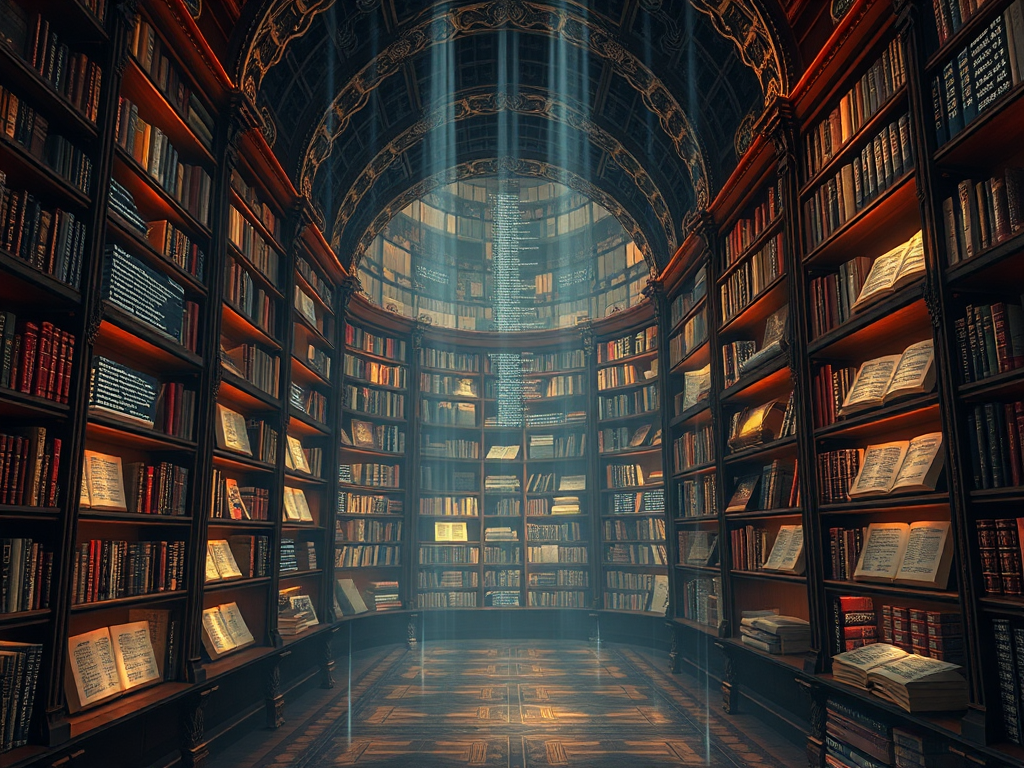
Introduction to Python Regular Expressions: Master Essential Text Processing Skills from Scratch
A comprehensive guide to Python regular expressions, covering fundamental concepts, special characters, re module functionality, and practical text processing examples for efficient pattern matching and manipulation
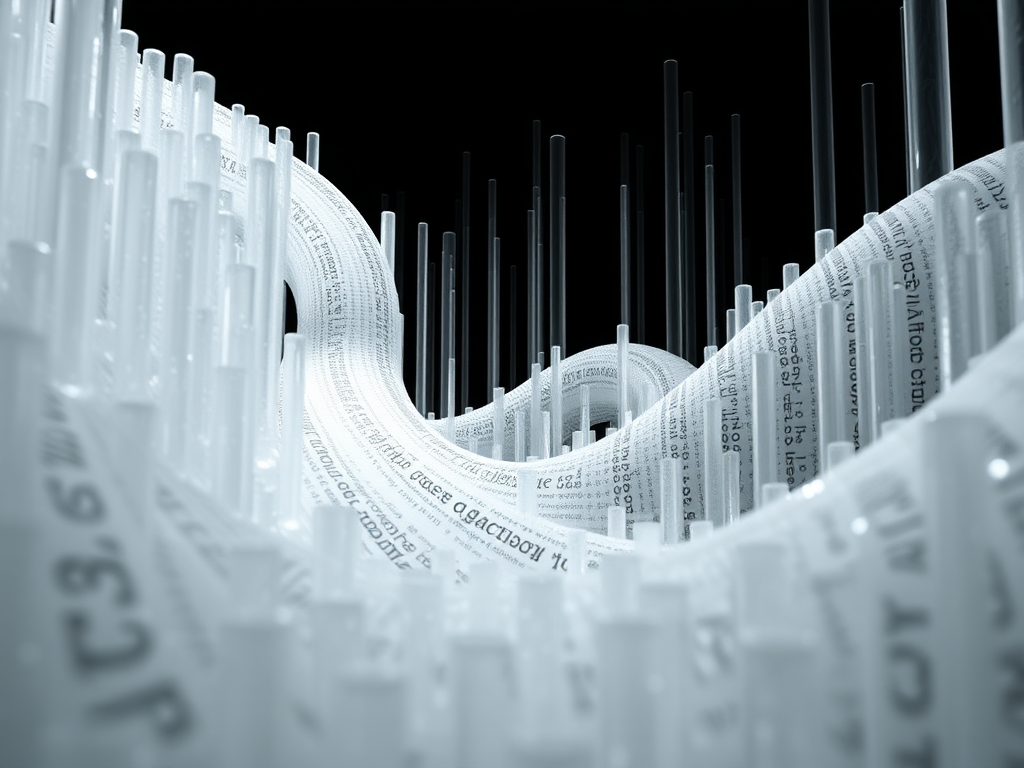
Python Regular Expressions: Mastering the Art of Text Processing from Scratch
A comprehensive guide to regular expressions in Python, covering basic concepts, core features of the re module, special characters usage, and practical email matching examples
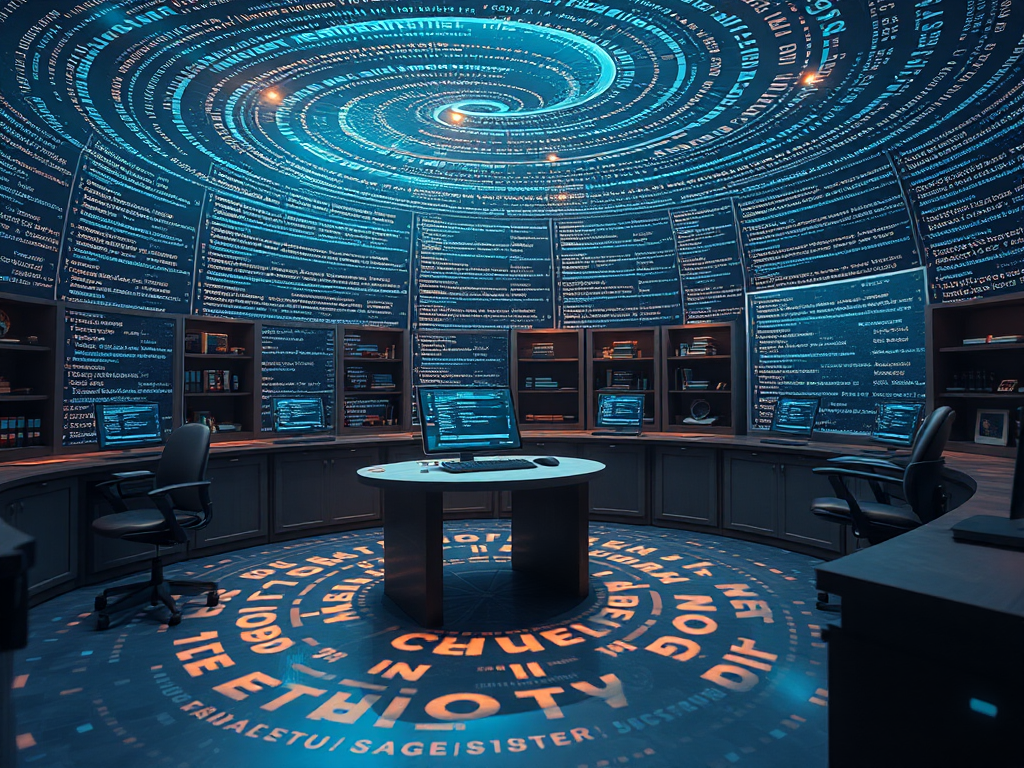
Python Regular Expressions: A Practical Guide from Beginner to Master
A comprehensive guide to Python regular expressions, covering basic concepts, re module usage, metacharacters, common functions, and practical examples including email matching and text replacement