Hello, dear Python enthusiasts! Today we're going to talk about regular expressions in Python. Regular expressions might sound complex, but they're actually like a Swiss Army knife, helping us easily tackle various text processing challenges. Have you ever struggled to extract specific information from a large chunk of text? Or been frustrated trying to batch replace certain strings? If so, regular expressions are definitely your savior!
When I first encountered regular expressions, I also thought their syntax looked as incomprehensible as hieroglyphics. But with continuous learning and practice, I discovered they are actually very powerful and flexible. Now, whenever I need to process text data, regular expressions are always my go-to tool. Today, let's dive deep into the mysteries of Python regular expressions together and see how they can make our programming lives easier and more enjoyable!
Basic Knowledge
Importing the Module
To use regular expressions in Python, we first need to import the re module. This module provides all the regular expression functionality we need. The import is very simple:
import re
It's that simple! Now we can start using the various functions in the re module. Do you find this import process simple? Personally, I think Python's module import mechanism is designed very elegantly, allowing us to easily use a wealth of functionality.
Pattern Matching
The core of regular expressions is pattern matching. We define a pattern, then use it to match text. The most basic matching is directly searching for a string:
text = "Hello, world!"
pattern = "world"
match = re.search(pattern, text)
if match:
print("Found:", match.group())
else:
print("Not found")
This code will output "Found: world". Isn't it intuitive? We defined a pattern "world", then searched for this pattern in the text. If found, we print it out.
You might ask, why use re.search() instead of the in operator directly? That's a good question! Although in this simple example, using in would achieve the same effect, re.search() is more powerful. It can not only find substrings but also perform more complex pattern matching, as we'll see next.
Metacharacter Magic
The Dot Wildcard
The power of regular expressions lies in their metacharacters. These special characters allow us to define more flexible patterns. For example, the dot (.) can match any character except a newline:
text = "cat, hat, rat, mat"
pattern = "..t"
matches = re.findall(pattern, text)
print(matches) # Output: ['cat', 'hat', 'rat', 'mat']
See that? We used "..t" to match all three-letter words ending with "t". This pattern means: "any two characters, followed by a t". Isn't it amazing?
When I first saw this feature, I was absolutely stunned. Think about it, without regular expressions, we might need to write a complex loop to achieve the same functionality. But with this little dot, everything becomes so simple!
The Power of Quantifiers
Quantifiers allow us to specify how many times a pattern should repeat. The most commonly used quantifiers are:
- *: Match 0 or more times
- +: Match 1 or more times
- ?: Match 0 or 1 time
Let's look at an example:
text = "color colour"
pattern = "colou?r"
matches = re.findall(pattern, text)
print(matches) # Output: ['color', 'colour']
This pattern "colou?r" means: "u may or may not be present". So it can match both the American spelling "color" and the British spelling "colour". Isn't that clever?
I remember once, I needed to process a large amount of text containing different national spellings. Without regular expressions, I might have needed to write many if-else statements. But with this little question mark, the problem was solved effortlessly. This is the charm of regular expressions!
Character Classes and Grouping
The Magic of Character Classes
Character classes allow us to define a set of characters, matching any one of them. We use square brackets [] to represent character classes:
text = "The quick brown fox jumps over the lazy dog."
pattern = "[aeiou]"
matches = re.findall(pattern, text)
print(matches) # Outputs all vowels
This pattern will match any vowel. Isn't it much more concise than writing them out one by one?
I once used this trick to analyze the ratio of vowels to consonants in an article. With just a few lines of code, I could get interesting linguistic data. Have you ever thought about using regular expressions to do some language analysis work?
Group Capture
Grouping allows us to combine parts of a regular expression together, which is particularly useful for extracting information:
text = "My phone number is 123-456-7890"
pattern = r"(\d{3})-(\d{3})-(\d{4})"
match = re.search(pattern, text)
if match:
print("Area code:", match.group(1))
print("First three digits:", match.group(2))
print("Last four digits:", match.group(3))
This example divides the phone number into three parts. We can extract the area code, the first three digits, and the last four digits separately. Doesn't it feel powerful?
I once used this trick to process a dataset containing thousands of phone numbers. With just a few lines of code, I could parse all the numbers into structured data. Can you imagine how troublesome it would be to do this manually?
Advanced Techniques
Greedy vs Non-Greedy
By default, regular expressions are greedy, meaning they will match as much as possible. But sometimes we need non-greedy matching:
text = "<p>This is a paragraph.</p><p>This is another paragraph.</p>"
greedy_pattern = "<p>.*</p>"
non_greedy_pattern = "<p>.*?</p>"
print(re.findall(greedy_pattern, text)) # Matches only once, including all content
print(re.findall(non_greedy_pattern, text)) # Matches twice, each paragraph separately
Do you see the difference? The greedy mode will keep matching until the last
, while the non-greedy mode will stop at the first it encounters.This trick is particularly useful when dealing with HTML or XML. I remember once, I needed to extract specific data from a complex webpage. Initially, I used greedy mode and kept getting the content of the entire page. Later, when I switched to non-greedy mode, the problem was immediately solved. This experience made me deeply realize the importance of understanding greedy and non-greedy matching.
Lookahead and Lookbehind
Lookahead and lookbehind allow us to match based on what comes before or after a pattern, without actually consuming these characters:
text = "I love python programming and pythons"
pattern = r"python(?=\s)"
matches = re.findall(pattern, text)
print(matches) # Only matches "python" followed by a space
This example will only match "python" followed by a space, so it won't match "pythons". This is very useful when precise matching is needed.
I once used this trick to analyze program source code, looking for specific function calls. By using lookahead, I could precisely find function names without mistakenly matching similar variable names. Can you think of scenarios in your work where lookahead and lookbehind might come in handy?
Practical Applications
Email Address Validation
Validating email addresses is a common requirement, let's see how to implement it using regular expressions:
def is_valid_email(email):
pattern = r'^[\w\.-]+@[\w\.-]+\.\w+$'
return re.match(pattern, email) is not None
emails = ["[email protected]", "invalid.email@com", "[email protected]"]
for email in emails:
print(f"{email} is {'valid' if is_valid_email(email) else 'invalid'}")
This regular expression might look a bit complex, but it's actually very powerful. It can match most common email formats.
I remember in a web project, we needed to validate user-input email addresses. Initially, we used a simple check, just looking for the @ symbol. Later we found that many invalid addresses were passing the check. After switching to this regular expression, our validation became much more reliable. How do you handle email validation in your projects?
Log Parsing
Suppose we have some log files in the following format:
2023-06-15 14:30:15 INFO User logged in: johndoe
2023-06-15 14:35:22 ERROR Failed to connect to database
2023-06-15 14:40:01 WARNING Disk space low
We can use regular expressions to parse these logs:
import re
log_pattern = r'(\d{4}-\d{2}-\d{2} \d{2}:\d{2}:\d{2}) (\w+) (.+)'
with open('logfile.txt', 'r') as file:
for line in file:
match = re.match(log_pattern, line)
if match:
timestamp, level, message = match.groups()
print(f"Time: {timestamp}, Level: {level}, Message: {message}")
This script can easily parse the log file into structured data. Don't you find it practical?
I once used a similar script in the operations work of a large system. There were large amounts of logs to analyze every day, and manual checking was impossible. With this script, we could quickly find all errors and warnings, greatly improving work efficiency. Have you encountered situations where you needed to process large amounts of logs? Did regular expressions give you a good solution?
Performance Considerations
Although regular expressions are very powerful, we also need to consider performance issues when dealing with large amounts of data. Here are a few tips:
- Use raw strings: In Python, adding r before a regular expression creates a raw string, avoiding unnecessary escaping:
pattern = r'\d+' # More readable than '\\d+'
- Compile regular expressions: If you're going to use the same regular expression multiple times, you can compile it first:
pattern = re.compile(r'\d+')
matches = pattern.findall(text)
- Use non-capturing groups: If you don't need to capture a group, you can use non-capturing groups to improve performance:
pattern = r'(?:ab)+c' # (?:...) indicates a non-capturing group
I once used these techniques in a project that needed to process several GB of text data. Initially, the script took several hours to run. After applying these optimizations, the running time was reduced to less than an hour. This made me deeply realize that in large-scale data processing, details really matter. Have you encountered performance issues when using regular expressions? How did you solve them?
Conclusion
Well, dear readers, our journey into regular expressions ends here. We've covered everything from basic pattern matching to complex grouping and lookarounds, to practical application cases, covering all aspects of regular expressions.
To recap, we learned about: 1. Basic pattern matching 2. Use of metacharacters 3. Character classes and grouping 4. Greedy vs non-greedy matching 5. Lookahead and lookbehind 6. Practical application cases 7. Performance optimization techniques
Regular expressions are like a small language, which might seem a bit difficult to understand at first. But trust me, once you master it, you'll find it's almost omnipotent when it comes to text processing! Every time I solve a complex problem with regular expressions, I get a sense of achievement that "technology changes the world".
Do you have any experiences or questions about regular expressions? Feel free to share your thoughts in the comments! If you found this article helpful, don't forget to share it with your friends.
Finally, I want to say, don't be intimidated by the complexity of regular expressions. Like learning any new skill, practice makes perfect. With more practice and application, you'll surely discover the wonder of regular expressions. Let's continue on this Python journey together!
Related searches:
+ Python Regular Expressions Tutorial
+ Python Regular Expressions Examples
+ Python Regular Expressions Cheat Sheet
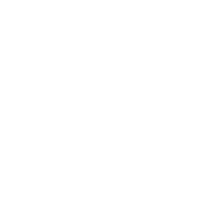
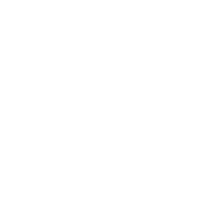
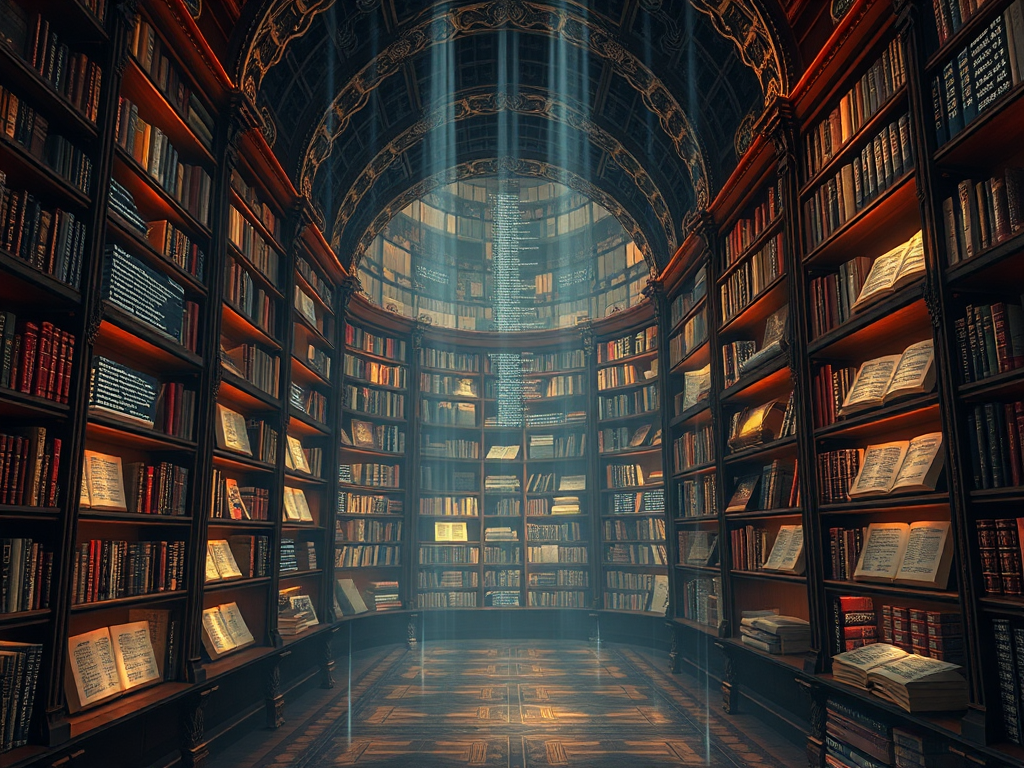
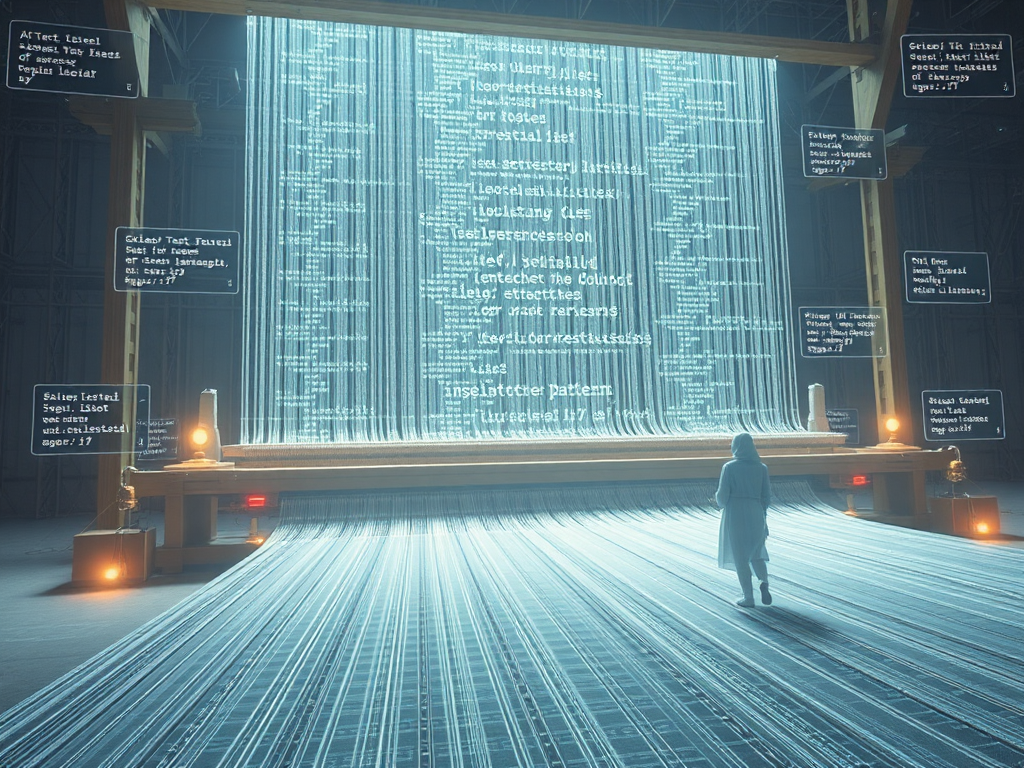
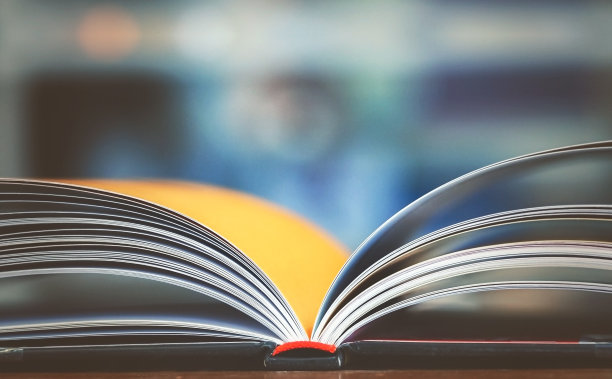