Introduction
Have you ever been troubled by processing large amounts of text data? Confused when validating email formats? Or felt helpless when extracting specific content from web pages? Today, let's dive deep into regular expressions in Python, this powerful and elegant text processing tool.
As a Python developer, I frequently use regular expressions in my daily programming. I remember once needing to extract specifically formatted timestamps from tens of thousands of log files. Using ordinary string processing methods would have required dozens of lines of code. But with regular expressions, the problem was elegantly solved with just one line. This made me deeply appreciate the charm of regular expressions.
Basics
Regular expressions may seem mysterious, but they're not difficult to understand. Imagine searching for a book in a library - you would use the author's name, book title, or ISBN number. Regular expressions are like more flexible "search conditions" that can describe the text patterns you want to match.
Let's start with a simple example. Suppose you want to find all phone numbers in a text, the code might look like this:
import re
text = "Xiao Ming's phone number is 13912345678, Xiao Hong's phone number is 13887654321"
pattern = r'1[3-9]\d{9}'
phone_numbers = re.findall(pattern, text)
print(phone_numbers) # ['13912345678', '13887654321']
Would you like to know how this code works?
Syntax
The syntax of regular expressions is like a miniature programming language. Let's look at some basic syntax rules:
- Character Matching First is basic character matching. Regular characters match themselves, for example, 'python' matches the string "python". However, in practical applications, we often need more flexible matching methods.
text = "I like Python programming, python is interesting"
pattern = r'[Pp]ython' # Matches Python or python
matches = re.findall(pattern, text)
print(matches) # ['Python', 'python']
-
Special Characters Special characters in regular expressions each have special meanings:
-
.
matches any single character (except newline) *
matches the preceding pattern zero or more times+
matches the preceding pattern one or more times?
matches the preceding pattern zero or one time^
matches the start of the string$
matches the end of the string
Let's look at a practical example:
text = """
Xiao Ming's scores:
Chinese: 89 points
Math: 95 points
English: 92 points
"""
pattern = r'\d{2}points' # Matches two digits followed by "points"
scores = re.findall(pattern, text)
print(scores) # ['89 points', '95 points', '92 points']
Advanced
After mastering basic syntax, let's look at some more advanced usage. In my development experience, these techniques often come in handy:
- Group Capture Using parentheses creates capture groups, which is very useful when extracting specific information:
text = "Birthday: 1990-12-15"
pattern = r'(\d{4})-(\d{1,2})-(\d{1,2})'
match = re.search(pattern, text)
if match:
year, month, day = match.groups()
print(f"Year: {year}, Month: {month}, Day: {day}")
- Greedy vs Non-greedy Matching Regular expressions are greedy by default, but sometimes we need non-greedy matching:
text = "<div>First div</div><div>Second div</div>"
pattern1 = r'<div>.*</div>'
print(re.findall(pattern1, text)) # ['<div>First div</div><div>Second div</div>']
pattern2 = r'<div>.*?</div>'
print(re.findall(pattern2, text)) # ['<div>First div</div>', '<div>Second div</div>']
Practical Applications
Now that we've covered the theory, let's reinforce our knowledge with some practical examples:
- Extracting all links from a webpage:
text = """
<a href="https://www.python.org">Python Official Website</a>
<a href="https://docs.python.org">Python Documentation</a>
"""
pattern = r'href="(.*?)"'
links = re.findall(pattern, text)
print(links) # ['https://www.python.org', 'https://docs.python.org']
- Validating Password Strength:
def check_password_strength(password):
# At least 8 characters, including uppercase, lowercase, numbers, and special characters
pattern = r'^(?=.*[a-z])(?=.*[A-Z])(?=.*\d)(?=.*[@$!%*?&])[A-Za-z\d@$!%*?&]{8,}$'
if re.match(pattern, password):
return "Password strength acceptable"
return "Password strength insufficient"
print(check_password_strength("Abc123!@")) # Password strength acceptable
print(check_password_strength("abc123")) # Password strength insufficient
- Processing Log Files:
log_text = """
2023-10-01 10:15:30 INFO: System startup
2023-10-01 10:15:35 ERROR: Database connection failed
2023-10-01 10:15:40 INFO: Retrying connection
"""
pattern = r'(\d{4}-\d{2}-\d{2} \d{2}:\d{2}:\d{2}) (INFO|ERROR): (.+)'
for line in log_text.strip().split('
'):
match = re.match(pattern, line)
if match:
timestamp, level, message = match.groups()
print(f"Time: {timestamp}")
print(f"Level: {level}")
print(f"Message: {message}")
print("---")
Optimization
There are some optimization tips worth noting when using regular expressions:
- Compiling Regular Expressions If you need to use the same regular expression multiple times, it's better to compile it first:
pattern = re.compile(r'\d+') # Compile the regular expression
text = "123 456 789"
numbers = pattern.findall(text) # Use the compiled regular expression
- Using Raw Strings In Python, using the r prefix to create raw strings can avoid escape character issues:
pattern1 = '\\d+' # Needs double backslash
pattern2 = r'\d+' # Clearer and more readable
- Avoiding Overly Complex Regular Expressions Sometimes, breaking down a complex regular expression into multiple simple ones might be better:
pattern = r'^(?=.*[A-Z])(?=.*[a-z])(?=.*\d)(?=.*[@$!%*?&])[A-Za-z\d@$!%*?&]{8,}$'
def check_password(password):
if len(password) < 8:
return False
if not re.search(r'[A-Z]', password):
return False
if not re.search(r'[a-z]', password):
return False
if not re.search(r'\d', password):
return False
if not re.search(r'[@$!%*?&]', password):
return False
return True
Summary
Regular expressions are a powerful tool, and mastering them can make our text processing work much more efficient. Remember, the best way to learn regular expressions is through practice and hands-on experience. You can start with simple patterns and gradually try more complex matching.
In actual development, I suggest you: 1. Start with simple regular expressions and gradually refine them 2. Use online regular expression testing tools to verify your expressions 3. Maintain readability in your regular expressions and add appropriate comments 4. Consider performance impacts and use compiled regular expressions when necessary
Do you find regular expressions interesting? Or have you encountered any problems while using them? Feel free to share your experiences and thoughts in the comments. In the next article, we'll explore advanced uses of regular expressions, so stay tuned.
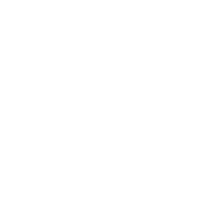
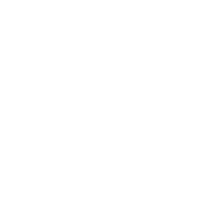