Hey, Python enthusiasts! Today let's talk about a hot topic - Python microservices architecture. Have you been hearing the word "microservices" a lot but feeling a bit lost? Don't worry, I'm here to help you navigate this complex yet fascinating topic.
Concept
First, what is microservices architecture? Simply put, it's about breaking down a large application into multiple small, independent services. Each service focuses on completing a specific function and collaborates with others through lightweight communication mechanisms (like HTTP APIs). This architectural approach allows us to develop, deploy, and scale applications more flexibly.
So what advantages does Python have in microservices development? I think there are mainly the following points:
- Concise syntax, high development efficiency
- Rich support from third-party libraries and frameworks
- Powerful asynchronous programming capabilities
- Active community support
Communication
When it comes to microservices, we can't avoid talking about inter-service communication. In this regard, RabbitMQ is a very popular choice. So, how to efficiently handle messages from RabbitMQ?
My suggestion is to use asynchronous processing and message confirmation mechanisms. This ensures message reliability while improving system efficiency. Specifically, you might consider using the aio_pika
library to implement asynchronous message processing. This library can help you significantly improve message processing throughput and response speed.
For example, suppose we have a microservice that handles user registration, which needs to receive user information from RabbitMQ and process it. Using aio_pika
, we can implement it like this:
import asyncio
from aio_pika import connect_robust, IncomingMessage
async def process_message(message: IncomingMessage):
async with message.process():
user_data = message.body.decode()
# Process user data
print(f"Processing user data: {user_data}")
await asyncio.sleep(1) # Simulate time-consuming operation
async def main():
connection = await connect_robust("amqp://guest:guest@localhost/")
channel = await connection.channel()
queue = await channel.declare_queue("user_registration")
async with queue.iterator() as queue_iter:
async for message in queue_iter:
await process_message(message)
if __name__ == "__main__":
asyncio.run(main())
This code demonstrates how to use aio_pika
to asynchronously process messages from RabbitMQ. By using asynchronous functions and the async with
statement, we can efficiently handle a large number of messages without blocking the execution of the entire program.
High Performance
Speaking of high performance, you might ask: How to build a high-load microservices architecture using Flask? This is an interesting question because Flask itself is a lightweight framework, but with some techniques, we can make it handle high-load scenarios.
The key is to use asynchronous programming to handle high concurrency requests. We can combine Flask with asyncio
, and use aiohttp
as an asynchronous HTTP client. This combination can greatly improve the system's concurrent processing capability.
Let's look at a specific example:
from flask import Flask
from flask_aiohttp import AioHTTP
import asyncio
app = Flask(__name__)
aiohttp = AioHTTP(app)
@app.route('/')
async def index():
async with aiohttp.session.get('https://api.example.com/data') as response:
data = await response.json()
return data
if __name__ == '__main__':
app.run()
In this example, we used the flask_aiohttp
extension to add asynchronous support to Flask. This way, we can use the async/await
syntax in route handler functions to achieve non-blocking HTTP requests.
However, using asynchronous programming alone is not enough. To truly handle high loads, we also need to consider decoupling between services. This is where message brokers (like RabbitMQ) come in handy. By using message queues, we can separate request processing from subsequent business logic, thereby improving the system's scalability and responsiveness.
Authentication
In microservices architecture, authentication and authorization between services is also an important topic. I personally recommend using JWT (JSON Web Tokens) to implement secure communication between services.
JWT works like this: When a user logs in successfully, the server generates a token containing user information and returns it to the client. Afterwards, the client carries this token in every request, and the server verifies the token to confirm the user's identity.
Let's look at an example of implementing JWT verification using the PyJWT library:
import jwt
from flask import Flask, request, jsonify
from functools import wraps
app = Flask(__name__)
app.config['SECRET_KEY'] = 'your-secret-key'
def token_required(f):
@wraps(f)
def decorated(*args, **kwargs):
token = request.args.get('token')
if not token:
return jsonify({'message': 'Token is missing!'}), 403
try:
data = jwt.decode(token, app.config['SECRET_KEY'])
except:
return jsonify({'message': 'Token is invalid!'}), 403
return f(*args, **kwargs)
return decorated
@app.route('/protected')
@token_required
def protected():
return jsonify({'message': 'This is a protected route!'})
if __name__ == '__main__':
app.run()
In this example, we defined a decorator token_required
that checks the validity of the token on each request. This way, we can easily add authentication to routes that need protection.
Service Discovery
When talking about microservices architecture, we can't ignore the topic of service discovery. As the number of services increases, manually managing service addresses becomes increasingly difficult. This is where service discovery tools come in handy.
Consul and Eureka are two commonly used service discovery tools. They can help microservices dynamically register and discover other services, greatly simplifying communication between services.
Taking Consul as an example, we can use it like this:
import consul
c = consul.Consul()
c.agent.service.register("web", service_id="web-1", port=8080)
index, services = c.health.service("web", passing=True)
for service in services:
print(service['Service']['Address'], service['Service']['Port'])
In this example, we first registered a service named "web", then queried all healthy "web" service instances through Consul's API. This way, we can dynamically obtain the addresses and ports of services without hardcoding this information.
Combined with load balancers, we can further improve the availability and performance of the system. For example, we can use Nginx as a load balancer to distribute requests to multiple service instances.
Transaction Processing
Finally, let's talk about a tricky issue in microservices architecture: how to handle long-running transactions?
In traditional monolithic applications, we can use database transactions to ensure the atomicity of operations. But in microservices architecture, this approach is not very suitable as it involves multiple services.
A common solution is to use the compensating transaction pattern. Simply put, it's about breaking down a large transaction into multiple small transactions, each with a corresponding compensating operation. If a step fails, we execute the compensating operations of the previous steps to ensure data consistency.
For example, suppose we have an order processing system involving three steps: creating an order, reducing inventory, and payment. We can implement it like this:
from functools import wraps
def compensating_transaction(compensate_func):
@wraps(compensate_func)
def wrapper(func):
def inner(*args, **kwargs):
try:
return func(*args, **kwargs)
except Exception as e:
compensate_func(*args, **kwargs)
raise e
return inner
return wrapper
def cancel_order(order_id):
# Logic for canceling order
print(f"Canceling order: {order_id}")
def restore_inventory(product_id, quantity):
# Logic for restoring inventory
print(f"Restoring inventory: Product {product_id}, Quantity {quantity}")
@compensating_transaction(cancel_order)
def create_order(order_id):
# Logic for creating order
print(f"Creating order: {order_id}")
@compensating_transaction(restore_inventory)
def reduce_inventory(product_id, quantity):
# Logic for reducing inventory
print(f"Reducing inventory: Product {product_id}, Quantity {quantity}")
def process_payment(order_id, amount):
# Logic for processing payment
print(f"Processing payment: Order {order_id}, Amount {amount}")
def process_order(order_id, product_id, quantity, amount):
try:
create_order(order_id)
reduce_inventory(product_id, quantity)
process_payment(order_id, amount)
print("Order processing successful")
except Exception as e:
print(f"Order processing failed: {str(e)}")
process_order("order-001", "product-001", 2, 100)
In this example, we added compensating operations for the create_order
and reduce_inventory
functions. If an exception occurs during processing, the corresponding compensating operation will be executed, thus ensuring data consistency.
In addition to the compensating transaction pattern, using message queues to process transactions asynchronously is also an effective strategy. This approach can improve system response speed while better handling dependencies between services.
Summary
Alright, we've discussed quite a bit about Python microservices architecture today. From basic concepts to specific implementation techniques, we've covered various aspects including communication, high performance, authentication, service discovery, and transaction processing.
While microservices architecture is complex, it also brings many benefits, such as better scalability and higher development efficiency. As Python developers, we have many excellent tools and libraries at our disposal, making building microservices simpler and more interesting.
What are your thoughts on microservices architecture? What challenges have you encountered in practice? Feel free to share your experiences and ideas in the comments section. Remember, there's no silver bullet in software development, choosing the architecture that suits your project is the most important thing.
Let's continue exploring together in this challenging and opportunistic world of microservices!
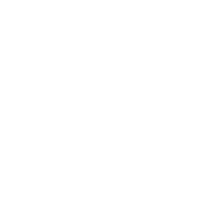
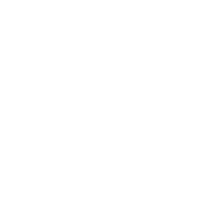
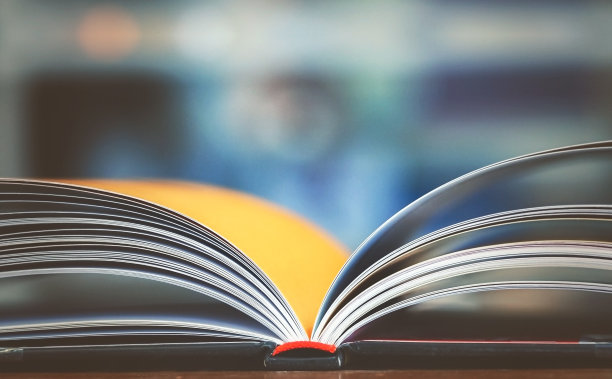
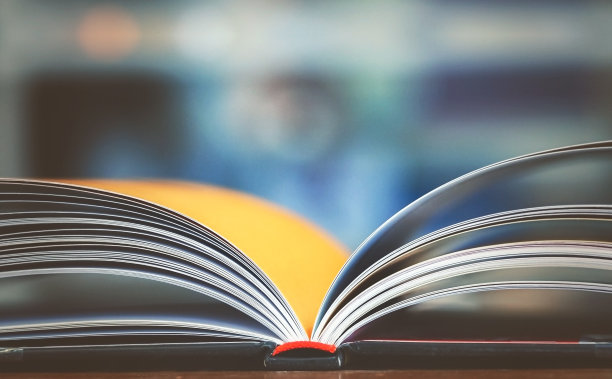
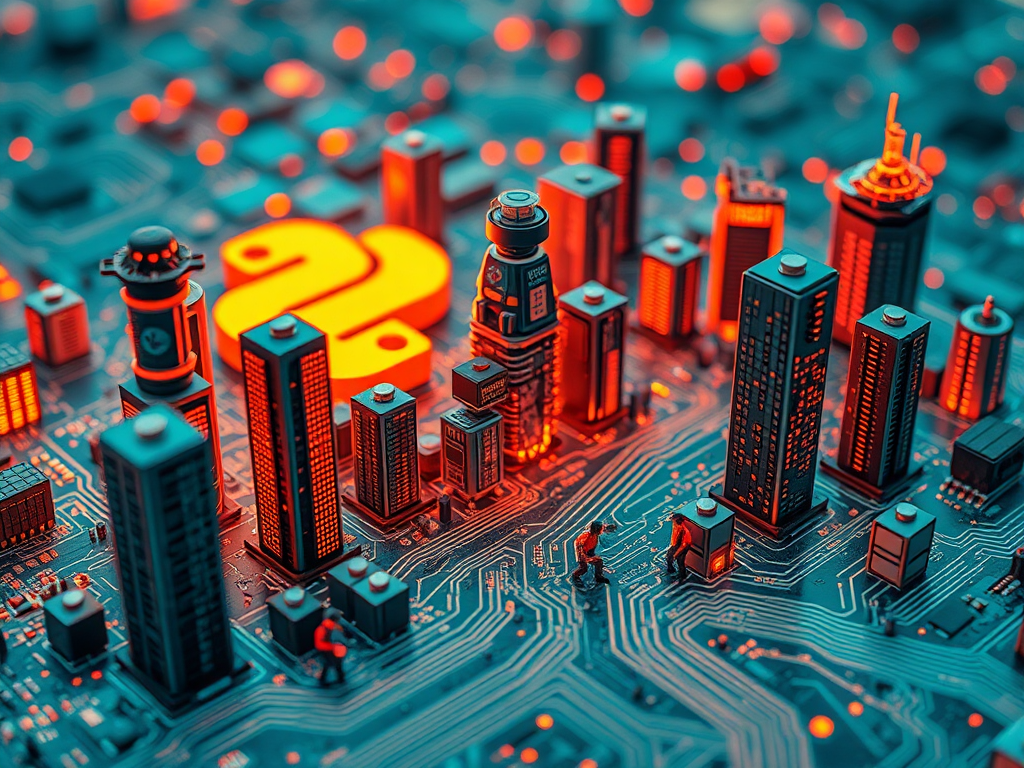