Have you ever felt that some functionality in your Python code needs to be repeatedly written? Or wanted to add extra features to existing functions without modifying the original function's code? If so, Python's decorators are a magical tool you can't miss! Today, let's delve into this powerful yet mysterious Python feature.
What is a Decorator?
A decorator is a fascinating feature in Python. Simply put, it is a function that allows other functions to gain additional functionality without needing any code changes. Does that sound abstract? Don't worry, let me explain with a real-life example.
Imagine you have a plain T-shirt, which is your basic function. Now, you want to make this T-shirt cooler, so you pin a badge on it. This badge is equivalent to a decorator; it adds new functionality (a cooler appearance) to your T-shirt (function), and you can remove it anytime without changing the T-shirt itself.
Why Use Decorators?
You might ask, why use decorators? Isn't directly modifying the function more straightforward? Great question! Let me give you a few reasons to use decorators:
-
Code Reuse: If you find yourself writing the same code in different functions (like logging, performance testing, etc.), using decorators can greatly reduce repetitive code.
-
Keep Original Functions Clean: Sometimes we need to add auxiliary functions to a function, but these are unrelated to the function's main logic. Using decorators can keep the main function clean and focused on core logic.
-
Dynamically Modify Function Behavior: Decorators allow us to dynamically modify function behavior at runtime, which is very useful in some scenarios.
-
Improve Code Readability: Proper use of decorators can make code structure clearer and intentions more explicit.
Basic Syntax of Decorators
Alright, I've said enough. You must be eager to see what decorators look like. Let's look at the simplest decorator example:
def my_decorator(func):
def wrapper():
print("Something is happening before the function is called.")
func()
print("Something is happening after the function is called.")
return wrapper
@my_decorator
def say_hello():
print("Hello!")
say_hello()
The output of this code will be:
Something is happening before the function is called.
Hello!
Something is happening after the function is called.
See? We defined a decorator my_decorator
, then used the @my_decorator
syntax to apply it to the say_hello
function. Thus, every time the say_hello
function is called, it gets "wrapped" by the my_decorator
decorator.
Isn't it magical? This is the power of decorators! They allow us to add new functionality to a function without modifying the original function.
Decorators with Parameters
The previous example was the most basic decorator, but in actual use, we often need to handle functions with parameters. Don't worry, decorators can easily handle this situation. Check out this example:
def repeat(times):
def decorator(func):
def wrapper(*args, **kwargs):
for _ in range(times):
result = func(*args, **kwargs)
return result
return wrapper
return decorator
@repeat(3)
def greet(name):
print(f"Hello, {name}!")
greet("Alice")
In this example, we defined a decorator repeat
that can accept parameters. It allows the decorated function to be executed a specified number of times. Running this code, you'll see "Hello, Alice!" printed three times.
Real-World Applications
After all this theory, you might wonder: what are these decorators useful for in real work? Let me give you some practical examples:
- Performance Testing: You can create a decorator to measure a function's execution time.
import time
def timing_decorator(func):
def wrapper(*args, **kwargs):
start_time = time.time()
result = func(*args, **kwargs)
end_time = time.time()
print(f"{func.__name__} took {end_time - start_time:.2f} seconds to execute.")
return result
return wrapper
@timing_decorator
def slow_function():
time.sleep(2)
slow_function()
- Logging: Using decorators can conveniently add logging functionality to functions.
import logging
logging.basicConfig(level=logging.INFO)
def log_decorator(func):
def wrapper(*args, **kwargs):
logging.info(f"Calling {func.__name__}")
result = func(*args, **kwargs)
logging.info(f"{func.__name__} finished")
return result
return wrapper
@log_decorator
def some_function():
print("Doing something...")
some_function()
- Authentication: In web applications, you can use decorators to check if a user is logged in.
def login_required(func):
def wrapper(*args, **kwargs):
if not user.is_authenticated:
return redirect(url_for('login'))
return func(*args, **kwargs)
return wrapper
@login_required
def protected_view():
return "This is a protected view"
These are just the tip of the iceberg for the applications of decorators. In actual development, decorators can be used for caching, permission checks, exception handling, and more.
Things to Note
While decorators are very powerful, there are some issues to be aware of when using them:
-
Performance Impact: Decorators add overhead to function calls. Be mindful of performance impacts, especially when used on frequently called functions.
-
Readability: Overusing decorators can reduce code readability. Ensure your team members understand the decorators you use.
-
Debugging Difficulty: Using decorators can make debugging more difficult because they change the function's behavior.
-
Preserve Function Metadata: Simple decorators will lose the decorated function's metadata (like function name, docstring, etc.). You can use
functools.wraps
to solve this issue.
from functools import wraps
def my_decorator(func):
@wraps(func)
def wrapper(*args, **kwargs):
"""This is the wrapper function"""
return func(*args, **kwargs)
return wrapper
@my_decorator
def example():
"""This is the example function"""
pass
print(example.__name__) # Output: example
print(example.__doc__) # Output: This is the example function
Conclusion
Python decorators are a powerful and flexible feature that allows us to extend and modify function behavior in an elegant way. By using decorators, we can achieve code reuse, separation of concerns, and dynamically modify function behavior.
In this article, we explored the basic concept and syntax of decorators, parameterized decorators, and some practical application scenarios. We also mentioned some issues to be aware of when using decorators.
Do you find decorators interesting? Have you thought of places where you can use decorators in your projects? Feel free to share your thoughts and experiences in the comments!
Remember, like all programming tools, decorators are a double-edged sword. Proper use can make your code more elegant and powerful, but overuse can bring negative effects. So, when using decorators, weigh the pros and cons based on actual situations.
Finally, I want to say that learning and mastering decorators may take some time and practice, but once you understand how they work, you'll find them a very useful tool in Python. So, don’t be afraid to try and practice. Programming is all about learning and exploring!
So, are you ready to try using decorators in your next Python project? Let's embark on this interesting programming journey together!
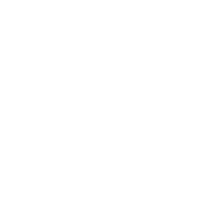
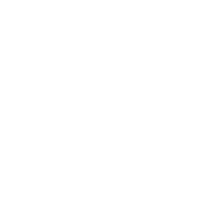